What is the ‘switch’ statement in Swift?
The `switch` statement in Swift is a powerful and versatile control structure used to evaluate a value against multiple possible cases and execute different blocks of code based on the matching case. It offers a concise and expressive way to handle complex branching logic in your code. Swift’s `switch` statement is more robust and feature-rich compared to traditional switch statements in other programming languages.
Here are the key features and usage of the `switch` statement in Swift:
- Pattern Matching: Swift’s `switch` statement supports extensive pattern matching. You can match not only values but also tuples, ranges, enums, and more complex patterns.
- No Fallthrough: Unlike some other programming languages, Swift’s `switch` statement does not fall through to the next case by default. After a case is matched and executed, the `switch` block exits unless you explicitly use the `fallthrough` keyword.
- Value Binding: You can bind the matched value to a constant or variable within a case, allowing you to use that value within the case block.
- Compound Cases: You can combine multiple cases in a single block, reducing redundancy and making your code cleaner.
- Default Case: You can include a `default` case to handle values that don’t match any of the defined cases.
Here’s a simple example of a `switch` statement in Swift:
```swift let day = "Monday" switch day { case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday": print("It's a weekday.") case "Saturday", "Sunday": print("It's a weekend.") default: print("Invalid day.") } ```
In this example, the `switch` statement evaluates the value of `day` and executes the corresponding block of code based on the matched case. The `default` case is used to handle any unexpected values.
Swift’s `switch` statement is a powerful tool for handling complex conditional logic in a clean and readable way. It’s commonly used not only for simple value matching but also for more intricate scenarios, such as pattern matching with enums or handling different cases based on associated values.
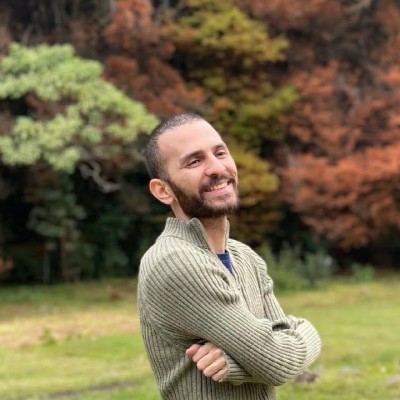
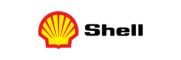