Achieving Quality Assurance in iOS with Swift Testing Frameworks
Quality assurance is a crucial part of software development. When it comes to iOS development, unit tests play an important role in ensuring that your applications function as expected. To effectively navigate this landscape, many teams choose to hire Swift developers experienced in various testing frameworks. Swift offers several tools that make testing a much smoother process. In this post, we’ll discuss some of the most popular and effective Swift testing frameworks, showcasing how they can streamline your testing and quality assurance workflows, thereby enhancing the capabilities of your hired Swift developers.
Table of Contents
1. XCTest
XCTest is Apple’s native testing framework for iOS, macOS, and other platforms. Introduced alongside Xcode 5, it is bundled with Xcode and integrated seamlessly, providing a framework for all types of tests: unit, performance, and UI.
Example
To demonstrate XCTest, consider the following example. Let’s say we have a simple function that concatenates two strings:
```swift func concatenateStrings(stringA: String, stringB: String) -> String { return stringA + stringB } ```
We can use XCTest to ensure this function works as expected:
```swift import XCTest class StringTests: XCTestCase { func testConcatenateStrings() { let stringA = "Hello" let stringB = " World" let result = concatenateStrings(stringA: stringA, stringB: stringB) XCTAssertEqual(result, "Hello World") } } ```
In the above example, `XCTestCase` is a base class that defines a test case, and `XCTAssertEqual` is a function that verifies if two expressions are equal. If the function `concatenateStrings` works correctly, the test will pass.
2. Quick/Nimble
Quick and Nimble are two powerful open-source testing frameworks for Swift and Objective-C. Quick is a behavior-driven development framework, and Nimble is a matching framework that provides more descriptive expectations.
Example
Let’s test the same function as before using Quick/Nimble. The syntax is different, but the principle is the same:
```swift import Quick import Nimble class StringSpec: QuickSpec { override func spec() { describe("Concatenate Strings") { it("concatenates two strings") { let stringA = "Hello" let stringB = " World" let result = concatenateStrings(stringA: stringA, stringB: stringB) expect(result).to(equal("Hello World")) } } } } ```
As you can see, Quick/Nimble provides a more human-readable syntax compared to XCTest, making it easier to understand what each test does.
3. KIF (Keep It Functional)
KIF is an iOS functional testing framework. It allows for easy automation of iOS apps by leveraging the accessibility attributes available in the iOS SDK.
Example
Let’s assume we have a login screen with two text fields (“username” and “password”) and a login button. KIF can simulate user interaction with these elements:
```swift import KIF class LoginTests: KIFTestCase { func testSuccessfulLogin() { tester().enterText("testUser", intoViewWithAccessibilityLabel: "username") tester().enterText("password123", intoViewWithAccessibilityLabel: "password") tester().tapView(withAccessibilityLabel: "Login") tester().waitForView(withAccessibilityLabel: "Welcome") } } ```
In this test, we are simulating a user entering a username and password and clicking the login button. If everything works correctly, we expect a welcome view to appear.
4. Snapshot Testing
Snapshot tests are a way to verify your UI doesn’t change unexpectedly. Instead of asserting against a variety of properties, you can take a snapshot of the whole UI and compare it with a reference snapshot stored in your repo.
Example
Using the framework iOSSnapshotTestCase (previously known as FBSnapshotTestCase), developed by Facebook, you can perform snapshot testing like so:
```swift import FBSnapshotTestCase class ViewControllerSnapshotTest: FBSnapshotTestCase { override func setUp() { super.setUp() self.recordMode = false } func testViewController() { let storyboard = UIStoryboard(name: "Main", bundle: nil) let viewController = storyboard.instantiateViewController(withIdentifier: "ViewController") FBSnapshotVerifyView(viewController.view) } } ```
The `FBSnapshotVerifyView` function captures a snapshot of the view and compares it to a reference image stored in your project. If the two images are different, the test will fail.
Conclusion
Testing is a critical part of iOS development, a fact well-recognized by teams that hire Swift developers to bolster their software quality. It ensures the stability of your app, reduces bugs, and promotes healthy development practices. Swift provides several testing frameworks that make the testing process easier and more effective, including XCTest, Quick/Nimble, KIF, and Snapshot Testing frameworks.
Choosing the right testing framework for your iOS project depends on your needs and requirements. This decision is especially crucial when you hire Swift developers, as their expertise in specific testing frameworks can significantly streamline your workflow. XCTest is a solid choice for most cases, but if you prefer a more human-readable syntax, Quick/Nimble might be better. If your app heavily relies on user interaction, KIF is a great choice, and for UI consistency, Snapshot Testing is extremely beneficial.
By integrating these tools into your development arsenal and aligning them with the skills of your hired Swift developers, you’ll be well-equipped to deliver stable, reliable iOS applications.
Table of Contents
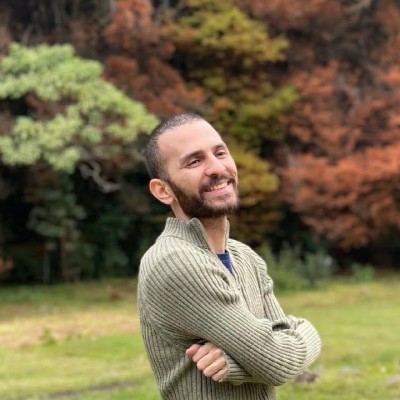
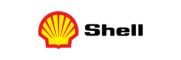