How do I use the ‘throws’ keyword in Swift?
In Swift, the `throws` keyword is used in the context of error handling to indicate that a function or method can potentially throw an error. Error handling in Swift allows you to gracefully handle exceptional situations and failures in your code. Here’s a detailed explanation of how to use the `throws` keyword in Swift:
- Function Signature:
– When you declare a function or method that can potentially throw an error, you include the `throws` keyword in its signature. This informs both the compiler and other developers that the function may propagate errors.
```swift func someFunction() throws -> ReturnType { // Function implementation } ```
- Throwing Errors:
– Within the body of a `throws` function, you can use the `throw` keyword to throw an error when a particular condition or situation warrants it. The error can be of any type that conforms to the `Error` protocol.
```swift func divide(_ a: Int, by b: Int) throws -> Int { guard b != 0 else { throw MyError.divisionByZero } return a / b } ```
In this example, the `divide(_:_:)` function throws a custom error `MyError.divisionByZero` when attempting to divide by zero.
- Calling a Throwing Function:
– When calling a `throws` function, you can either use a `try` keyword or handle the error using a `do-catch` block. The `try` keyword indicates that you acknowledge the potential for an error to occur.
```swift do { let result = try divide(10, by: 0) print("Result: \(result)") } catch { print("Error: \(error)") } ```
In this code, we use `try` to call `divide(_:_:)` and handle the error in the `catch` block.
- Propagation of Errors:
– If a `throws` function is called within another `throws` function or method, errors can be propagated up the call stack until they are caught and handled or until they reach the top-level scope where they terminate the program.
- Error Types:
– Swift provides a range of error types, including built-in ones like `NSError` and custom error types that you can define to represent specific error conditions in your code. Error types must conform to the `Error` protocol.
- Error Handling Strategies:
– When using the `throws` keyword, you should have a clear error-handling strategy. You can handle errors using `do-catch` blocks, propagate them to higher levels of the code, or even convert them into optional values using `try?` if you want to ignore errors in a non-critical context.
In summary, the `throws` keyword in Swift is essential for building robust and reliable code that can gracefully handle errors and exceptional situations. It allows you to indicate where errors may occur, throw specific error types, and handle errors using various error-handling mechanisms, ensuring that your code remains resilient in the face of unexpected issues.
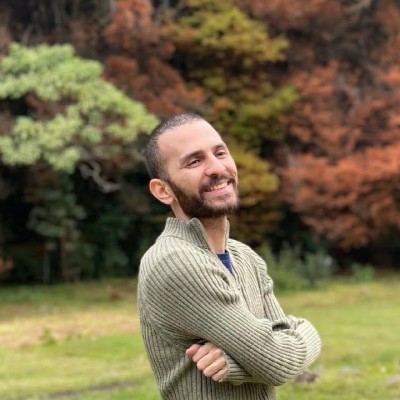
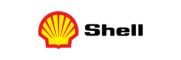