What is the ‘try!’ keyword in Swift?
In Swift, the ‘try!’ keyword is a powerful yet potentially risky construct used for error handling when working with throwing functions and methods. It’s important to understand how ‘try!’ works and when to use it judiciously. Here’s a comprehensive explanation:
- Understanding Throwing Functions: Before delving into ‘try!’, it’s crucial to grasp the concept of throwing functions in Swift. Some functions and methods are marked as “throwing,” which means they can potentially encounter errors during execution. These errors must be handled explicitly.
- Traditional Error Handling: The conventional approach for handling errors from throwing functions involves using do-try-catch blocks. When you use ‘try’ within such a block, you are essentially saying, “Try to execute this code, and if an error occurs, catch it and handle it appropriately.”
```swift do { let result = try someThrowingFunction() // Use the result } catch { // Handle the error } ```
- The ‘try!’ Keyword: ‘try!’ is a variation of ‘try’ that differs significantly in behavior. When you use ‘try!’ with a throwing function, you’re telling Swift, “I am certain that no error will occur at this point, and if it does, I want the program to crash.” In other words, you’re forcefully unwrapping the result of a throwing function.
```swift let result = try! someThrowingFunction() ```
- Use Cases and Considerations: You should use ‘try!’ cautiously and only when you are absolutely certain that the throwing function will not produce an error. It is typically employed in situations where failure is a critical and unrecoverable error for your application. For example, initializing a crucial component of your app may be a place where ‘try!’ is appropriate.
- Risks of ‘try!‘: If you use ‘try!’ and an error actually occurs, your application will crash. Therefore, it’s essential to exercise extreme caution and only use ‘try!’ when you have a high degree of confidence that an error will not happen. Misusing ‘try!’ can lead to instability in your application.
- Alternative Approaches: In most cases, traditional error handling with do-try-catch blocks is preferred because it allows you to gracefully handle errors and provide fallback mechanisms without risking a crash.
In summary, ‘try!’ in Swift is a powerful tool that should be used sparingly and with utmost caution. It forcefully unwraps the result of a throwing function, assuming no errors will occur. While it can be beneficial in specific situations, its misuse can lead to application crashes, making it imperative to use it only when you are absolutely certain that no errors will be encountered.
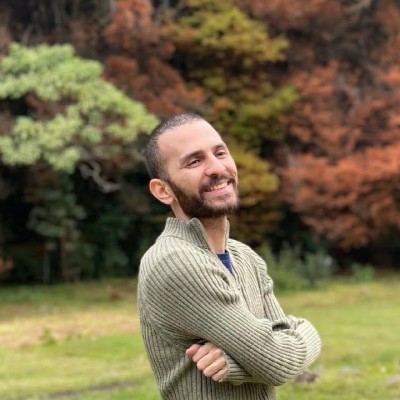
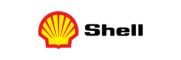