What is a tuple in Swift?
In Swift, a tuple is a lightweight and flexible data structure used to group together multiple values into a single compound value. Unlike arrays or dictionaries, which hold collections of values, tuples allow you to combine different types of values, and each element within a tuple can have its own data type. Tuples are particularly useful when you need to return multiple values from a function, or when you want to group related data together.
Here’s how you can define and use tuples in Swift:
Tuple Declaration:
To create a tuple, you enclose the values in parentheses, separating them with commas. Each element within the tuple can have a name (known as a label) and a value, or it can have just a value without a label. For example:
```swift let person: (String, Int) = ("Alice", 30) ```
In this example, we have a tuple named `person` containing a string (name) and an integer (age).
Tuple Elements:
You can access the elements of a tuple using dot notation along with the element’s label or index. For example:
```swift let name = person.0 // Accessing the first element let age = person.1 // Accessing the second element ```
Tuple Decomposition:
You can also decompose a tuple’s elements into separate variables or constants in a single line, which makes code more readable:
```swift let (personName, personAge) = person ```
Now, `personName` holds “Alice” and `personAge` holds 30.
Named Elements:
You can provide labels for tuple elements to make your code more self-explanatory:
```swift let personInfo: (name: String, age: Int) = (name: "Bob", age: 25) let bobName = personInfo.name let bobAge = personInfo.age ```
Function Return Values:
Tuples are often used to return multiple values from a function. This is a convenient way to package and transmit different pieces of information as a single entity.
In summary, tuples in Swift are versatile and allow you to group together multiple values of different types, making them a handy tool for various programming scenarios. Whether for returning values from functions, representing key-value pairs, or grouping related data, tuples provide a lightweight and expressive way to work with multiple values in a single unit.
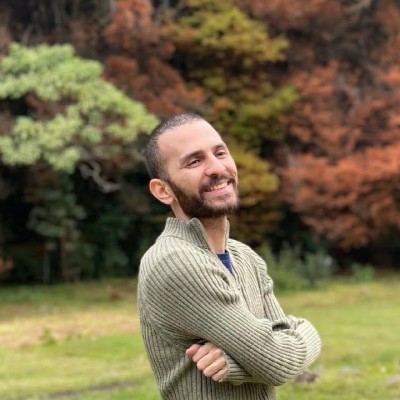
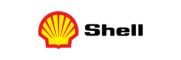