What is type aliasing in Swift?
In Swift, type aliasing is a feature that allows you to create alternative names for existing data types. It provides a way to make your code more readable, expressive, and maintainable by giving custom names to complex or lengthy type declarations. Type aliases are essentially shortcuts or nicknames for existing types and are defined using the `typealias` keyword.
Here’s how you can use type aliasing in Swift:
- Creating Type Aliases: To create a type alias, you use the `typealias` keyword followed by the new name you want to give to an existing type. This can be particularly useful for complex data types or when you want to provide more meaningful names.
```swift typealias Temperature = Double typealias StudentID = String typealias Coordinate = (Double, Double) ```
In this example, we’ve created type aliases for `Double`, `String`, and a tuple `(Double, Double)`.
- Improved Code Clarity: Type aliases make your code more self-explanatory and easier to understand, especially when dealing with custom data structures or domain-specific types. For example, instead of using `Double` throughout your code, you can use the `Temperature` alias, making it clear that you’re working with temperature values.
```swift func convertToFahrenheit(celsius: Temperature) -> Temperature { return (celsius * 9/5) + 32 } ```
Here, it’s clear that the function works with temperature values.
- Code Maintenance: Type aliases also help in code maintenance. If you need to change the underlying type in the future, you only have to update the alias definition rather than hunting down and changing every occurrence of the type throughout your codebase.
```swift typealias Temperature = Float ```
Changing the type of `Temperature` here automatically updates it wherever it’s used.
In summary, type aliasing in Swift is a powerful tool for improving code readability, expressiveness, and maintainability. It allows you to create custom names for existing data types, making your code more self-explanatory and adaptable to changes in your application’s requirements.
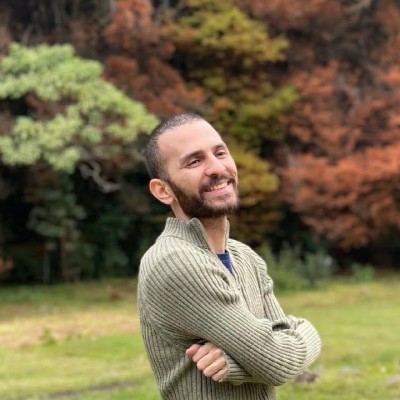
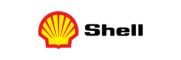