What is a typealias in Swift?
In Swift, a typealias is a way to create a new name for an existing data type, making your code more readable and expressive. It does not create a new type but rather provides a more descriptive and meaningful name for an existing type, which can enhance code clarity and maintainability. Typealiases are particularly useful when you’re working with complex data types or when you want to improve the readability of your code.
Here’s how you can define a typealias:
```swift typealias Distance = Double ```
In this example, we’ve created a typealias named `Distance` for the `Double` data type. Now, instead of using `Double` directly in your code, you can use `Distance` to make it more semantically meaningful:
```swift let length: Distance = 10.5 ```
Typealiases are especially handy when working with closures, custom data structures, or complex generics. For example, you might create a typealias for a closure that takes two integers and returns a boolean:
```swift typealias Predicate = (Int, Int) -> Bool let isEven: Predicate = { (a, b) in return (a + b) % 2 == 0 } ```
This makes the purpose of the closure much clearer when used in your code.
In summary, typealias in Swift is a way to define custom names for existing data types, improving the readability and clarity of your code. It simplifies complex type signatures, enhances code maintainability, and makes your intentions more explicit, ultimately leading to more understandable and expressive Swift code.
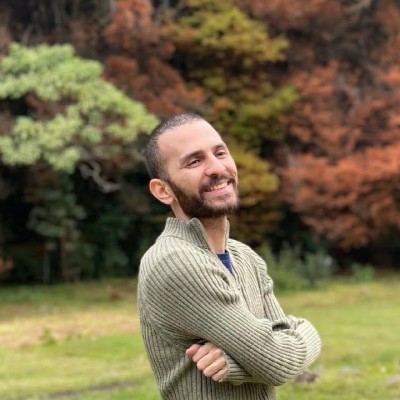
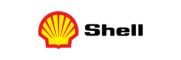