How do I use the ‘try?’ keyword in Swift?
In Swift, the ‘try?’ keyword is a powerful construct used for error handling when working with throwing functions and methods. It provides a convenient way to handle errors while minimizing the need for extensive do-try-catch blocks. Here’s how you use the ‘try?’ keyword in Swift:
- Understanding Throwing Functions: Before delving into ‘try?’ specifically, let’s understand throwing functions. In Swift, some functions and methods can “throw” errors when something goes wrong. For example, when working with file operations, network requests, or parsing data, errors can occur, and Swift enforces handling these errors.
- Conventional Error Handling: In the past, you might have used a do-try-catch block to handle errors from throwing functions. It looks like this:
```swift do { let result = try someThrowingFunction() // Use the result } catch { // Handle the error } ```
This approach requires you to explicitly catch errors and can lead to verbose code.
- The ‘try?’ Keyword: ‘try?’ simplifies error handling. When you use ‘try?’ with a throwing function or method, Swift automatically handles any errors and returns an optional result. If the function throws an error, the result is nil; otherwise, it contains the actual value returned by the function.
```swift let result = try? someThrowingFunction() ```
With ‘try?’, you don’t need a do-try-catch block, and the code is more concise. You can check the ‘result’ for nil to determine if an error occurred:
```swift if let unwrappedResult = result { // Use the unwrapped result } else { // An error occurred } ```
- Advantages of ‘try?’: ‘try?’ is particularly useful in scenarios where you can proceed with a default value or behavior when an error occurs. It simplifies error handling, reduces code clutter, and makes your code more readable.
- Considerations: While ‘try?’ is convenient, it discards the error information, so you won’t know the specific error that occurred. If you need detailed error handling or want to provide custom error messages, you may still opt for traditional do-try-catch blocks.
In summary, ‘try?’ in Swift is a valuable tool for simplifying error handling in scenarios where you can gracefully handle errors by providing a default value or behavior. It streamlines your code and enhances its readability, making your error-handling logic more concise and efficient.
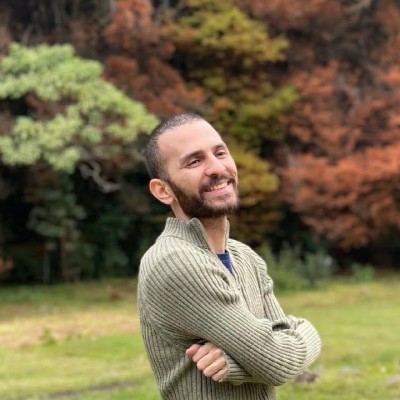
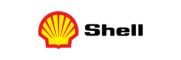