Swift Q & A
How do I use optionals in Swift?
Optionals are a fundamental concept in Swift that allows variables or constants to represent the presence or absence of a value. They are used to handle situations where a value might be missing, and they play a crucial role in ensuring type safety and preventing runtime crashes. Here’s how you can use optionals effectively in Swift:
- Declaration: To declare an optional, you add a question mark `?` after the data type. For example:
```swift var age: Int? // An optional integer that can have a value or be nil var name: String? // An optional string ```
- Assignment: You can assign a value to an optional variable or constant using the `=` operator. For example:
```swift age = 25 ```
- Accessing Values: To access the value stored in an optional, you need to unwrap it. You can use optional binding with `if let` or `guard let` statements to safely unwrap and use the value:
```swift if let validAge = age { // validAge is the unwrapped value of age } else { // age is nil } ```
- Implicitly Unwrapped Optionals: You can declare an implicitly unwrapped optional using `!` after the data type. These optionals are automatically unwrapped when accessed, assuming they have a value:
```swift var phoneNumber: String! = "123-456-7890" let length = phoneNumber.count // No need to unwrap phoneNumber here ```
- Handling Nil: When dealing with optionals, it’s essential to check for nil values to avoid runtime crashes. You can use conditional statements to check for nil before unwrapping.
- Optional Chaining: You can use optional chaining (`?.`) to call methods, access properties, and subscript elements of an optional, safely handling situations where the optional is nil:
```swift let streetName = address?.street?.name ```
- Forced Unwrapping: You can force unwrap an optional using `!`, but this should be done cautiously, as it may lead to runtime crashes if the optional is nil.
In summary, optionals in Swift are a powerful tool for handling situations where values may be missing. They ensure type safety and encourage safe and reliable code by making it explicit when a value can be absent. By understanding how to declare, assign, and safely unwrap optionals, you can write more robust and error-resistant Swift code.
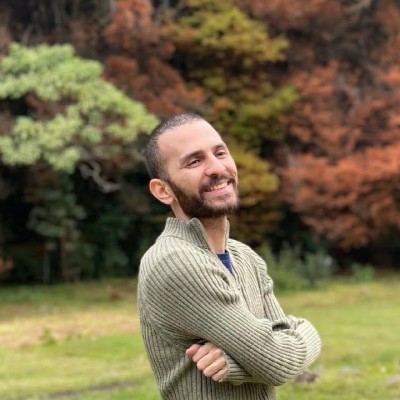
Previously at
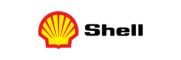
Experienced iOS Engineer with 7+ years mastering Swift. Created fintech solutions, enhanced biopharma apps, and transformed retail experiences.