What is a ‘while’ loop in Swift?
A ‘while’ loop in Swift is a fundamental control structure that allows you to repeatedly execute a block of code as long as a specified condition remains true. It’s a powerful tool for creating loops that continue until a particular condition is no longer met, making it ideal for situations where you don’t know in advance how many iterations are needed.
Here’s how a ‘while’ loop works in Swift:
- Initialization: First, you declare any necessary variables and initialize them before entering the loop.
- Condition: Next, you specify a condition that the loop will evaluate before each iteration. If the condition is true, the loop body will execute; if it’s false, the loop terminates.
- Loop Body: The code inside the ‘while’ loop’s block gets executed as long as the condition remains true. It’s crucial to include logic that can potentially change the condition within the loop to avoid infinite loops.
Here’s a simple example of a ‘while’ loop in Swift that counts from 1 to 5:
```swift var counter = 1 while counter <= 5 { print(counter) counter += 1 } ```
In this example, the ‘while’ loop initializes a ‘counter’ variable to 1. It continues looping as long as ‘counter’ is less than or equal to 5. Inside the loop, ‘counter’ is incremented by 1 in each iteration, and the loop prints the value of ‘counter.’ The loop stops when ‘counter’ becomes greater than 5.
‘while’ loops are versatile and can be used in various scenarios, such as reading data from a file until the end is reached, continuously checking for user input, or implementing game loops. However, it’s essential to ensure that the loop’s condition eventually becomes false to prevent infinite looping, which could cause your program to hang or crash.
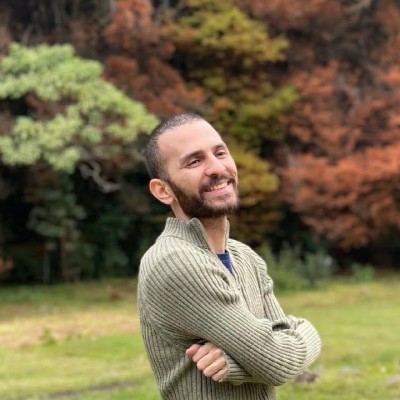
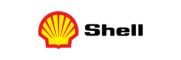