Building RESTful APIs with Symfony’s Serialization Components
In today’s web development landscape, building RESTful APIs is a common requirement for many applications. These APIs allow different systems to communicate and exchange data in a structured manner. Symfony, a popular PHP framework, provides powerful tools to simplify the development of RESTful APIs. One such tool is Symfony’s Serialization Components, which handle the serialization, normalization, and deserialization of data. In this blog post, we will explore how to use these components to build robust and efficient RESTful APIs.
Introduction to Symfony’s Serialization Components
Symfony’s Serialization Components offer a comprehensive set of tools to handle the serialization and deserialization of PHP objects. These components provide a flexible way to convert complex data structures into formats like JSON, XML, or YAML, making it easier to transmit and consume data across different platforms and applications.
The key components of Symfony’s Serialization Components are:
- Serializer: The Serializer component is responsible for converting PHP objects into different formats and vice versa. It supports various serialization formats, including JSON, XML, YAML, and others. The Serializer can handle both serialization (object to string) and deserialization (string to object) operations.
- Normalizer: The Normalizer component is used to transform complex PHP objects into simpler representations suitable for serialization. It helps in normalizing object properties, handling circular references, and resolving relationships between objects.
- Encoder: The Encoder component takes care of encoding and decoding serialized data. It provides support for different formats such as JSON, XML, and others, allowing you to choose the most suitable format for your API.
Now that we have a basic understanding of Symfony’s Serialization Components, let’s dive into the practical implementation of building RESTful APIs using these powerful tools.
Setting Up a Symfony Project
Before we start building our RESTful API, we need to set up a Symfony project. Make sure you have Symfony installed on your system. If not, you can follow the official Symfony documentation to install it.
Once Symfony is installed, open your terminal and run the following command to create a new Symfony project:
bash symfony new my_api_project --full
This command will create a new Symfony project with all the necessary components and dependencies. Navigate to the project directory:
bash cd my_api_project
Creating a Simple API Endpoint
Let’s start by creating a simple API endpoint that returns a list of books. Open your terminal and run the following command to generate a new controller:
bash php bin/console make:controller BookController
This command will generate a new BookController class under the src/Controller directory. Open the generated BookController.php file and modify it as follows:
php namespace App\Controller; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\JsonResponse; use Symfony\Component\Routing\Annotation\Route; class BookController extends AbstractController { /** * @Route("/api/books", name="api_books", methods={"GET"}) */ public function getBooks(): JsonResponse { $books = [ ['title' => 'Book 1', 'author' => 'Author 1'], ['title' => 'Book 2', 'author' => 'Author 2'], ['title' => 'Book 3', 'author' => 'Author 3'], ]; return $this->json($books); } }
In the above code, we have created a getBooks() method that returns a JSON response containing an array of books. The json() method provided by Symfony automatically serializes the array into JSON format.
Serializing Objects with Symfony’s Serializer
In real-world scenarios, we often need to serialize complex objects and return them as JSON responses. Let’s modify our previous example to demonstrate how Symfony’s Serializer component can handle object serialization.
First, create a new directory called Entity under the src directory. Inside the Entity directory, create a new PHP class called Book.php and define the following code:
namespace App\Entity; class Book { private $title; private $author; public function __construct(string $title, string $author) { $this->title = $title; $this->author = $author; } // Getters and setters public function getTitle(): string { return $this->title; } public function setTitle(string $title): void { $this->title = $title; } public function getAuthor(): string { return $this->author; } public function setAuthor(string $author): void { $this->author = $author; } }
Next, update the getBooks() method in BookController.php to use the Book entity:
php use App\Entity\Book; // ... public function getBooks(): JsonResponse { $books = [ new Book('Book 1', 'Author 1'), new Book('Book 2', 'Author 2'), new Book('Book 3', 'Author 3'), ]; return $this->json($books); }
Now, when you access the /api/books endpoint, the Book objects will be automatically serialized into JSON format, thanks to Symfony’s Serializer component.
Normalizing Data with Symfony’s Normalizer
Symfony’s Normalizer component allows you to transform complex objects into simpler representations suitable for serialization. Let’s update our example to demonstrate how the Normalizer works.
Modify the Book entity class to implement the NormalizableInterface provided by Symfony:
php use Symfony\Component\Serializer\Normalizer\NormalizableInterface; use Symfony\Component\Serializer\Normalizer\NormalizerInterface; class Book implements NormalizableInterface { // ... public function normalize(NormalizerInterface $normalizer, string $format = null, array $context = []) { return [ 'title' => $this->title, 'author' => $this->author, ]; } }
In the normalize() method, we define how the Book object should be normalized. We return an associative array containing the book’s title and author.
Now, when you access the /api/books endpoint, the Book objects will be normalized using the normalize() method, and the resulting array will be serialized into JSON format.
Conclusion
Building RESTful APIs with Symfony’s Serialization Components provides a powerful and flexible way to handle serialization, normalization, and deserialization of data. In this blog post, we learned about the key components of Symfony’s Serialization Components and how to use them to build robust and efficient APIs.
We explored the Serializer component, which allows us to convert PHP objects into different formats and vice versa. We also saw how the Normalizer component helps in transforming complex objects into simpler representations suitable for serialization.
By leveraging Symfony’s Serialization Components, you can easily handle data conversion in your RESTful APIs, ensuring smooth communication and interoperability between different systems.
Start using Symfony’s Serialization Components today and unlock the full potential of your RESTful API development!
Table of Contents
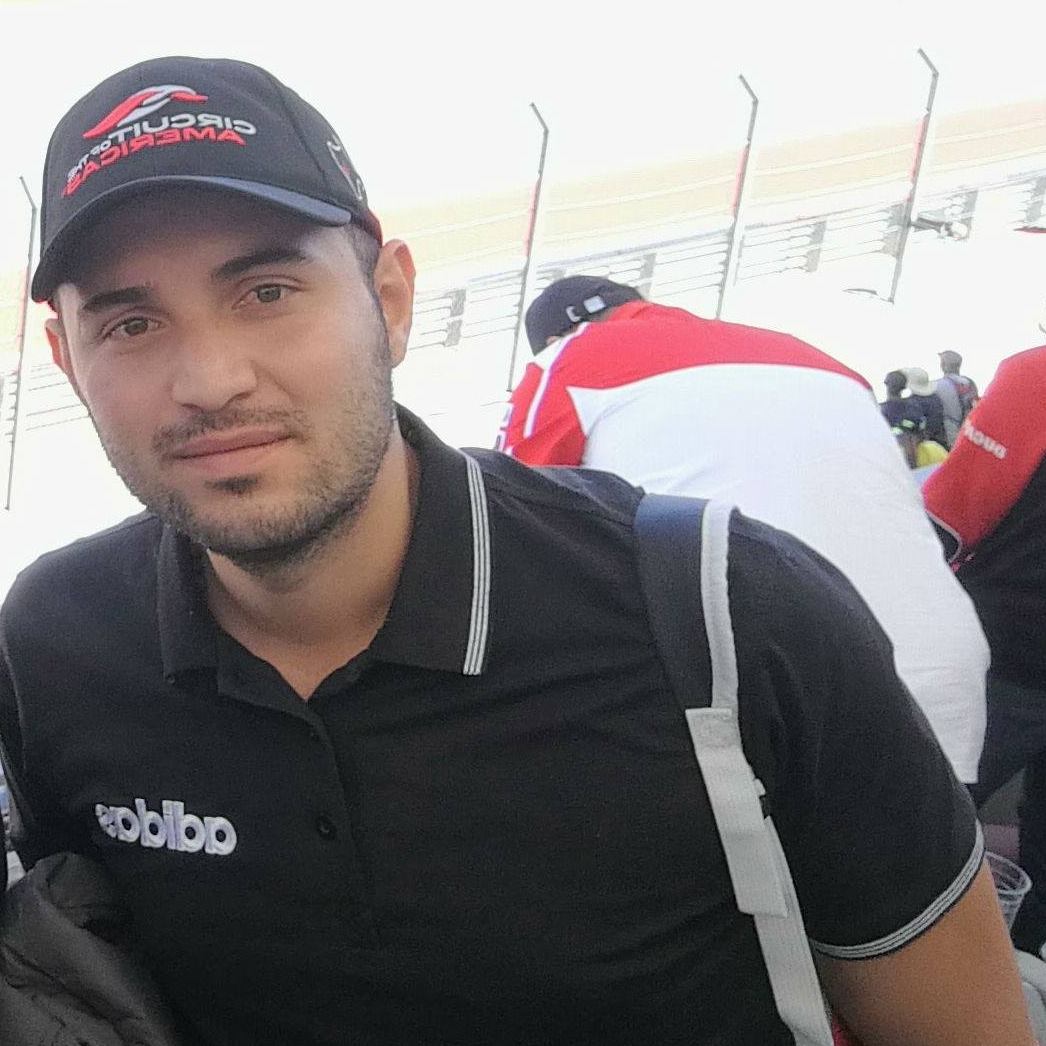
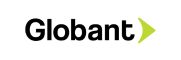