Building Robust Web Applications with Symfony
Building robust web applications requires a combination of a solid development framework and best practices. Symfony, one of the most popular PHP frameworks, provides a powerful toolset to create scalable and maintainable web applications. In this blog post, we will explore the key concepts, best practices, and code samples that will help you build robust web applications with Symfony.
1. Understanding Symfony
1.1 Introduction to Symfony:
Symfony is an open-source PHP framework that follows the Model-View-Controller (MVC) architectural pattern. It provides a set of reusable components, libraries, and tools that simplify the development process. Symfony promotes code reusability, testability, and scalability, making it an excellent choice for building web applications.
1.2 Key Features of Symfony:
Symfony offers several key features that contribute to building robust web applications:
- Powerful Routing: Symfony provides a flexible routing system that allows mapping URLs to controllers and actions easily.
- Templating Engine: Symfony integrates with Twig, a popular templating engine that provides a clean and efficient way to separate HTML from PHP code.
- Object-Relational Mapping (ORM): Symfony utilizes Doctrine, a robust ORM, for managing database interactions, making it easy to work with databases.
- Authentication and Authorization: Symfony provides a comprehensive security component that handles user authentication, authorization, and role-based access control.
- Caching and Performance: Symfony offers various caching mechanisms, including HTTP caching and Doctrine caching, to improve application performance.
- Testing and Debugging: Symfony provides a testing framework with tools like PHPUnit and a built-in profiler for efficient debugging and performance optimization.
1.3 Symfony Components:
Symfony is built upon a collection of decoupled and reusable components. Some of the essential Symfony components are:
- HttpFoundation: Handles HTTP-related tasks, such as request/response handling and session management.
- Routing: Manages URL routing and generates URLs based on defined routes.
- EventDispatcher: Implements the publish-subscribe pattern for event-driven development.
- Form: Simplifies the creation and handling of HTML forms.
- Security: Provides authentication, authorization, and encryption capabilities.
- DependencyInjection: Helps manage object dependencies and promotes loose coupling.
- Console: Enables building command-line interfaces for Symfony applications.
- Translation: Facilitates localization and internationalization of web applications.
2. Setting Up a Symfony Project
2.1 Installing Symfony:
To get started with Symfony, you need to have PHP and Composer installed on your system. Use Composer, the dependency management tool for PHP, to install Symfony by running the following command:
shell composer create-project symfony/website-skeleton myproject
2.2 Creating a New Symfony Project:
After installing Symfony, create a new project using the Symfony CLI or Composer:
shell symfony new myproject
or
shell composer create-project symfony/skeleton myproject
2.3 Directory Structure:
Symfony follows a well-defined directory structure that promotes modularity and organization. Some of the essential directories in a Symfony project are:
- src/: Contains the application’s source code, including controllers, entities, and services.
- config/: Holds configuration files for different environments, routing, services, and more.
- templates/: Contains Twig templates for rendering views.
- public/: Stores publicly accessible files like CSS, JavaScript, and media assets.
- tests/: Holds test files for unit testing and functional testing.
3. Building the Foundation
3.1 Routing and Controllers:
Routing is a crucial aspect of any web application. Symfony provides a flexible routing system that allows defining routes and mapping them to controllers. Here’s an example of defining a route in config/routes.yaml:
yaml # config/routes.yaml homepage: path: / controller: App\Controller\HomeController::index
In the above example, the route with the path ‘/’ is mapped to the index action of the HomeController class.
3.2 Templating with Twig:
Symfony integrates with Twig, a powerful and developer-friendly templating engine. Twig helps separate the presentation layer from the application logic. Here’s an example of rendering a Twig template in a controller:
php // src/Controller/HomeController.php namespace App\Controller; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route; class HomeController extends AbstractController { /** * @Route("/") */ public function index(): Response { return $this->render('home/index.html.twig', [ 'name' => 'John Doe', ]); } }
In the above example, the index action renders the home/index.html.twig template and passes the ‘name’ variable to the template.
3.3 Database Interaction with Doctrine:
Symfony integrates seamlessly with Doctrine, a widely used Object-Relational Mapping (ORM) tool. Doctrine provides an abstraction layer to interact with the database. Here’s an example of defining an entity and querying the database using Doctrine:
php // src/Entity/User.php namespace App\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="users") */ class User { /** * @ORM\Id * @ORM\GeneratedValue * @ORM\Column(type="integer") */ private $id; /** * @ORM\Column(type="string") */ private $name; // Getters and setters... } // src/Controller/UserController.php namespace App\Controller; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route; use App\Entity\User; class UserController extends AbstractController { /** * @Route("/users") */ public function index(): Response { $users = $this->getDoctrine()->getRepository(User::class)->findAll(); return $this->render('user/index.html.twig', [ 'users' => $users, ]); } }
In the above example, the User entity is defined with annotations to map it to the users table in the database. The index action fetches all users from the database and passes them to the user/index.html.twig template.
4. Enhancing Application Security
4.1 Authentication and Authorization:
Symfony provides a comprehensive security component that handles authentication and authorization. It supports various authentication methods such as form-based authentication, token-based authentication (e.g., JWT), and OAuth. Additionally, it allows configuring role-based access control (RBAC) to restrict user access to specific parts of the application.
4.2 Guard Authentication:
Symfony’s Guard authentication component simplifies the implementation of custom authentication methods. It enables you to define your authentication logic, such as validating credentials or retrieving user information from an external API. Here’s an example of implementing a custom Guard authenticator:
php // src/Security/ApiKeyAuthenticator.php namespace App\Security; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\Security\Core\User\UserInterface; use Symfony\Component\Security\Guard\AbstractGuardAuthenticator; use Symfony\Component\Security\Core\Exception\AuthenticationException; use Symfony\Component\HttpFoundation\JsonResponse; class ApiKeyAuthenticator extends AbstractGuardAuthenticator { // Implement the required methods... } // src/Security/ApiKeyUserProvider.php namespace App\Security; use Symfony\Component\Security\Core\User\UserInterface; use Symfony\Component\Security\Core\User\UserProviderInterface; class ApiKeyUserProvider implements UserProviderInterface { // Implement the required methods... }
In the above example, the ApiKeyAuthenticator class extends AbstractGuardAuthenticator to define the authentication logic. The ApiKeyUserProvider class implements UserProviderInterface to load the user based on the API key.
4.3 Handling User Access Control:
Symfony’s security component also provides the ability to restrict user access to specific parts of your application. You can define access control rules based on routes or URL patterns. Here’s an example of restricting access to a specific route:
yaml # config/packages/security.yaml security: access_control: - { path: ^/admin, roles: ROLE_ADMIN }
In the above example, any route starting with /admin requires the user to have the ROLE_ADMIN role.
5. Leveraging Symfony Bundles
5.1 Introduction to Bundles:
Symfony bundles are reusable packages that encapsulate specific functionality. Bundles can be easily installed and configured in a Symfony application, providing additional features and integration options.
5.2 Popular Symfony Bundles:
Symfony has a vibrant ecosystem with numerous bundles available. Some popular Symfony bundles include:
- DoctrineBundle: Integrates Doctrine ORM with Symfony.
- TwigBundle: Integrates the Twig templating engine.
- SecurityBundle: Provides security-related features and configurations.
- MonologBundle: Integrates the Monolog logging library.
- MailerBundle: Provides email sending capabilities.
- EasyAdminBundle: Generates an administration interface for managing entities.
5.3 Installing and Configuring Bundles:
You can install bundles using Composer. After installing a bundle, register it in the config/bundles.php file or in the configuration files of your application. Bundle-specific configuration options are usually defined in the config/packages directory.
6. Optimizing Performance
6.1 Caching Strategies:
Caching is crucial for improving application performance. Symfony provides various caching mechanisms, including HTTP caching, database query caching, and full-page caching. You can configure caching at different levels, such as the framework level, Twig templates, or specific parts of your application.
6.2 Query Optimization:
Efficient database querying is essential for a high-performing application. Symfony’s Doctrine integration provides query optimization techniques such as eager loading, result caching, and query tuning. By utilizing these techniques, you can minimize unnecessary database round trips and optimize the overall application performance.
6.3 Asset Management:
Symfony provides an asset management system to handle CSS, JavaScript, and other static assets. By using asset management techniques like asset versioning, asset concatenation, and minification, you can reduce the number of HTTP requests and improve page load times.
7. Testing and Debugging
7.1 Unit Testing with PHPUnit:
Symfony encourages test-driven development (TDD) by providing a seamless integration with PHPUnit, a popular PHP testing framework. You can write unit tests for individual classes, controllers, or services to ensure their correctness and maintainability.
7.2 Functional Testing with Symfony’s Testing Framework:
Symfony’s testing framework allows you to write functional tests that simulate HTTP requests and assert the responses. This enables you to test the behavior of your application from end to end, ensuring proper integration between different components.
7.3 Debugging Techniques:
Symfony provides a built-in web profiler that gives you detailed information about each request, including executed queries, memory usage, and timeline data. Additionally, Symfony integrates with popular debugging tools like Xdebug, enabling you to step through your code and identify and fix issues efficiently.
8. Continuous Integration and Deployment
8.1 Integrating Symfony with CI/CD Pipelines:
Symfony applications can be seamlessly integrated into Continuous Integration (CI) and Continuous Deployment (CD) pipelines. You can use popular CI/CD tools like Jenkins, Travis CI, or GitLab CI/CD to automate the testing, building, and deployment processes.
8.2 Deploying Symfony Applications:
Symfony applications can be deployed to various hosting platforms, including shared hosting, virtual private servers (VPS), and cloud platforms like AWS, Azure, or Heroku. You can deploy Symfony applications using deployment tools like Capistrano or by manually transferring the codebase and configuring the server environment.
9. Monitoring and Logging
9.1 Application Logging:
Symfony integrates with the Monolog logging library, allowing you to log application events, errors, and debug information. You can configure different logging channels, log to different output sources (e.g., files, databases, or external services), and define log levels based on the severity of the message.
9.2 Performance Monitoring:
Monitoring the performance of your Symfony application is crucial to identify bottlenecks and optimize the codebase. You can utilize tools like Blackfire or New Relic to profile your application, analyze performance data, and identify areas for improvement.
9.3 Error Tracking:
To ensure the stability of your application, it is essential to track and handle errors effectively. Services like Sentry or Bugsnag can be integrated with Symfony to collect error reports, notify developers about critical issues, and provide detailed information for debugging and resolution.
10. Scaling and Performance Optimization
10.1 Load Balancing:
As your application grows, load balancing becomes necessary to distribute the incoming traffic across multiple servers. Symfony can be easily scaled horizontally by adding more servers and using load balancers like Nginx or HAProxy to distribute the requests.
10.2 Caching Strategies for High Traffic Applications:
To handle high traffic, caching becomes even more critical. Strategies like reverse proxy caching, content delivery networks (CDNs), or distributed caching systems like Redis or Memcached can significantly improve the performance and scalability of your Symfony application.
10.3 Scaling Symfony Components:
Symfony’s decoupled components can be used independently to build microservices or APIs. By dividing your application into smaller, self-contained components, you can scale them independently, achieving better performance and manageability.
Conclusion:
Building robust web applications with Symfony requires understanding the key concepts, best practices, and utilizing the rich ecosystem of Symfony components and bundles. In this blog post, we covered various aspects of Symfony development, including routing, controllers, templating, security, testing, caching, deployment, and performance optimization. By applying these techniques and following Symfony’s best practices, you can create scalable, maintainable, and robust web applications with ease.
Table of Contents
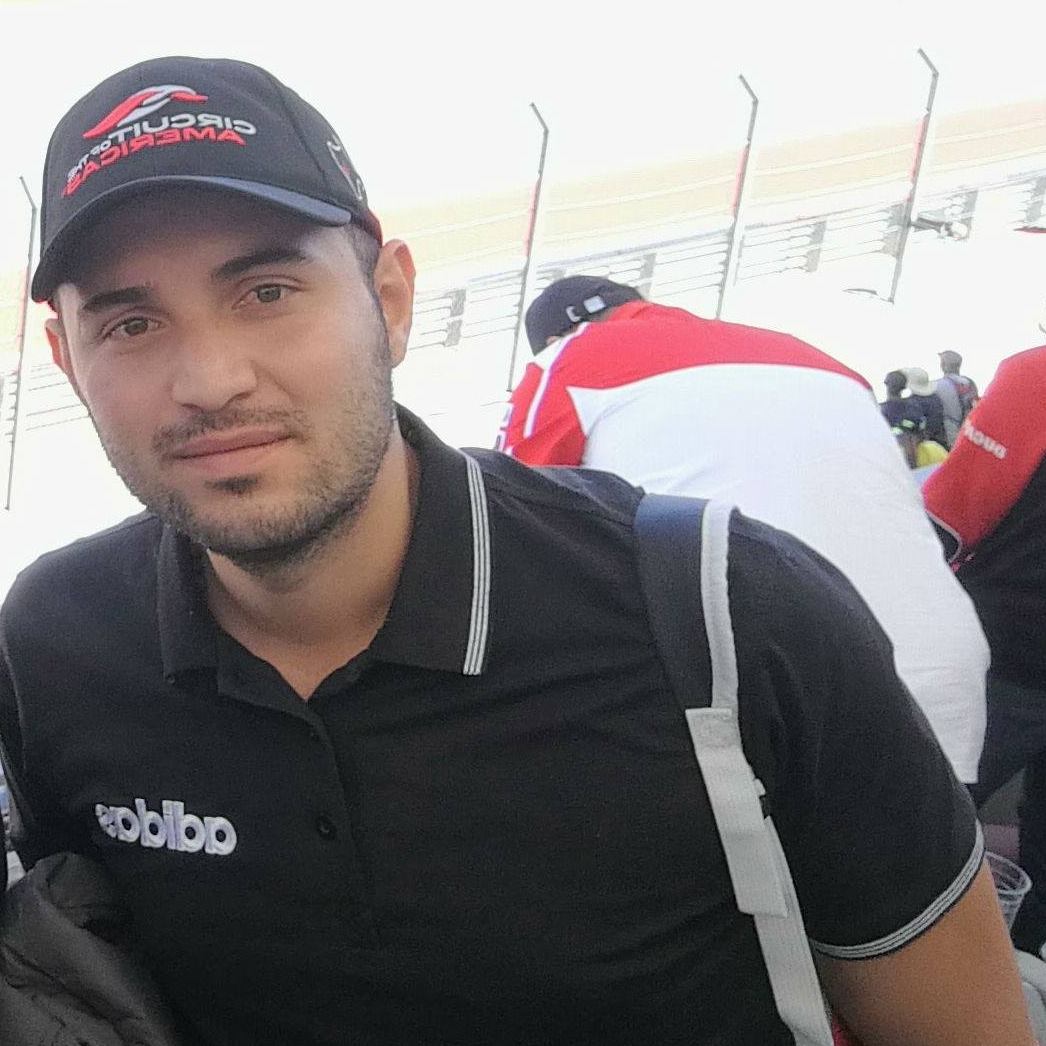
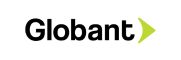