Symfony Bundles: Extending Functionality with Pre-built Components
Symfony, one of the most popular PHP frameworks, is known for its flexibility, robustness, and extensive set of components. These components make development faster and more efficient by providing a range of ready-to-use tools to handle common tasks. Among these components, Symfony Bundles stand out as powerful packages that extend the framework’s functionality significantly.
In this blog, we’ll explore Symfony Bundles and delve into their importance in Symfony projects. We’ll learn how they enhance development and save time by integrating pre-built components into your applications. From essential concepts to practical examples, we’ll cover it all, so let’s get started!
What are Symfony Bundles?
At the core of Symfony lies the concept of Bundles. A Bundle is a structured set of files and directories that encapsulate specific functionality. These bundles can be seamlessly integrated into Symfony projects to extend the framework’s capabilities. Each Bundle is designed to function independently, making it easy to reuse them across various projects.
Symfony itself comes with several built-in Bundles that handle critical tasks such as routing, templating, and form handling. Additionally, the Symfony community actively contributes various third-party Bundles, allowing developers to access a vast library of pre-built components.
The Advantages of Symfony Bundles
Using Symfony Bundles offers several advantages that enhance the development experience:
- Code Reusability: Bundles encourage the reuse of code, which is one of the fundamental principles of software development. By leveraging pre-built components, developers can avoid reinventing the wheel and focus on building unique features specific to their projects.
- Modularity: Symfony Bundles promote a modular approach to application development. Each Bundle encapsulates a particular feature or functionality, making it easier to maintain and understand complex projects.
- Community Contributions: The Symfony community actively contributes to a vast repository of Bundles, covering a wide range of use cases. This thriving ecosystem saves developers time and effort, as they can integrate well-tested solutions seamlessly.
- Flexibility and Customizability: Symfony Bundles can be customized and extended to suit the specific requirements of your project. Developers can override or extend Bundle classes, templates, and configurations to tailor them precisely to their needs.
- Rapid Development: Leveraging pre-built components allows developers to implement features quickly and efficiently. This accelerated development process translates into faster time-to-market for your Symfony application.
Working with Symfony Bundles
Now that we understand the benefits of Symfony Bundles, let’s dive into how to work with them effectively.
1. Installing Bundles
Symfony Bundles can be installed using Symfony’s package manager, Composer. Let’s say we want to install a hypothetical bundle called “MyAwesomeBundle.” Open your terminal and run the following command:
bash composer require vendor/my-awesome-bundle
Composer will handle the installation process and update your Symfony project’s dependencies accordingly.
2. Registering Bundles
Once installed, Symfony requires you to register the Bundle in the config/bundles.php file. This step ensures that Symfony loads and initializes the Bundle during the application’s bootstrap process. Find the appropriate line in the file and add the Bundle class, like so:
php return [ // ... Vendor\MyAwesomeBundle\MyAwesomeBundle::class => ['all' => true], ];
3. Configuring Bundles
Bundles often come with default configurations that you can adjust to fit your project’s needs. These configurations typically reside in the config/packages directory. Create a new file named my_awesome_bundle.yaml to override or customize the Bundle’s default settings:
yaml my_awesome_bundle: setting_one: true setting_two: 'custom_value'
4. Using Bundle Services
Symfony Bundles often provide services that encapsulate specific functionalities. To use a service from a Bundle, you can access it via Symfony’s dependency injection container. For instance, if “MyAwesomeBundle” provides a service called “MyService,” you can inject it into your controller:
php use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route; use Vendor\MyAwesomeBundle\Service\MyService; class MyController extends AbstractController { /** * @Route("/my-route", name="my_route") */ public function myAction(MyService $myService): Response { // Use the service to perform actions $result = $myService->doSomething(); // ... } }
5. Extending Bundle Functionalities
Symfony Bundles are designed to be extensible. If you need to modify or extend the behavior of a Bundle, Symfony provides various techniques to achieve this:
- Configuration Overrides: As we saw earlier, you can override default configurations to customize the behavior of a Bundle.
- Class Extension: If a Bundle contains specific classes, you can extend those classes in your project and override their methods to change their behavior.
- Event Listeners: Many Bundles dispatch events at various points during their execution. You can attach custom event listeners and react to those events to add or modify functionality.
- Service Decorators: Symfony’s service container allows you to decorate existing services provided by a Bundle. This technique is useful when you want to add functionality to a service without altering its original implementation.
Practical Example: SymfonyMailerBundle
Let’s explore a practical example by considering the built-in “SymfonyMailerBundle,” which provides email functionalities for Symfony applications. The bundle simplifies the process of sending emails by encapsulating various services and configurations.
1. Installation
To install the SymfonyMailerBundle, run the following command:
bash composer require symfony/mailer
2. Configuration
Once installed, you can configure the bundle to use a specific email provider. In this example, we’ll use Gmail as the email provider. Add the following configuration to config/packages/mailer.yaml:
yaml framework: mailer: dsn: 'smtp://user:password@gmail.com?encryption=tls&auth_mode=login'
3. Sending Emails
With the SymfonyMailerBundle set up, you can now use it to send emails from your Symfony application. Consider a scenario where you want to send a welcome email to a new user after registration. You can create a service to handle this functionality:
php use Symfony\Component\Mailer\MailerInterface; use Symfony\Component\Mime\Email; class EmailService { private $mailer; public function __construct(MailerInterface $mailer) { $this->mailer = $mailer; } public function sendWelcomeEmail(string $recipientEmail): void { $email = (new Email()) ->from('noreply@example.com') ->to($recipientEmail) ->subject('Welcome to Our Website') ->text('Hello and welcome to our website!'); $this->mailer->send($email); } }
4. Using the Email Service
You can now use the EmailService to send welcome emails in your controller:
php use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route; use App\Service\EmailService; class UserController extends AbstractController { /** * @Route("/register", name="user_register") */ public function register(EmailService $emailService): Response { // ... Process registration logic ... // Send welcome email $emailService->sendWelcomeEmail('newuser@example.com'); // ... Return response ... } }
Conclusion
Symfony Bundles provide a powerful way to extend Symfony’s functionality by integrating pre-built components into your projects. By leveraging Bundles, developers can save time, ensure code reusability, and benefit from a vibrant community ecosystem. In this blog, we covered the significance of Symfony Bundles, their advantages, and practical examples of using one in a Symfony project.
As you continue your Symfony journey, explore the vast collection of Bundles contributed by the Symfony community. By incorporating these pre-built components into your applications, you’ll streamline development, build robust features, and create exceptional web applications with ease. Happy coding!
Table of Contents
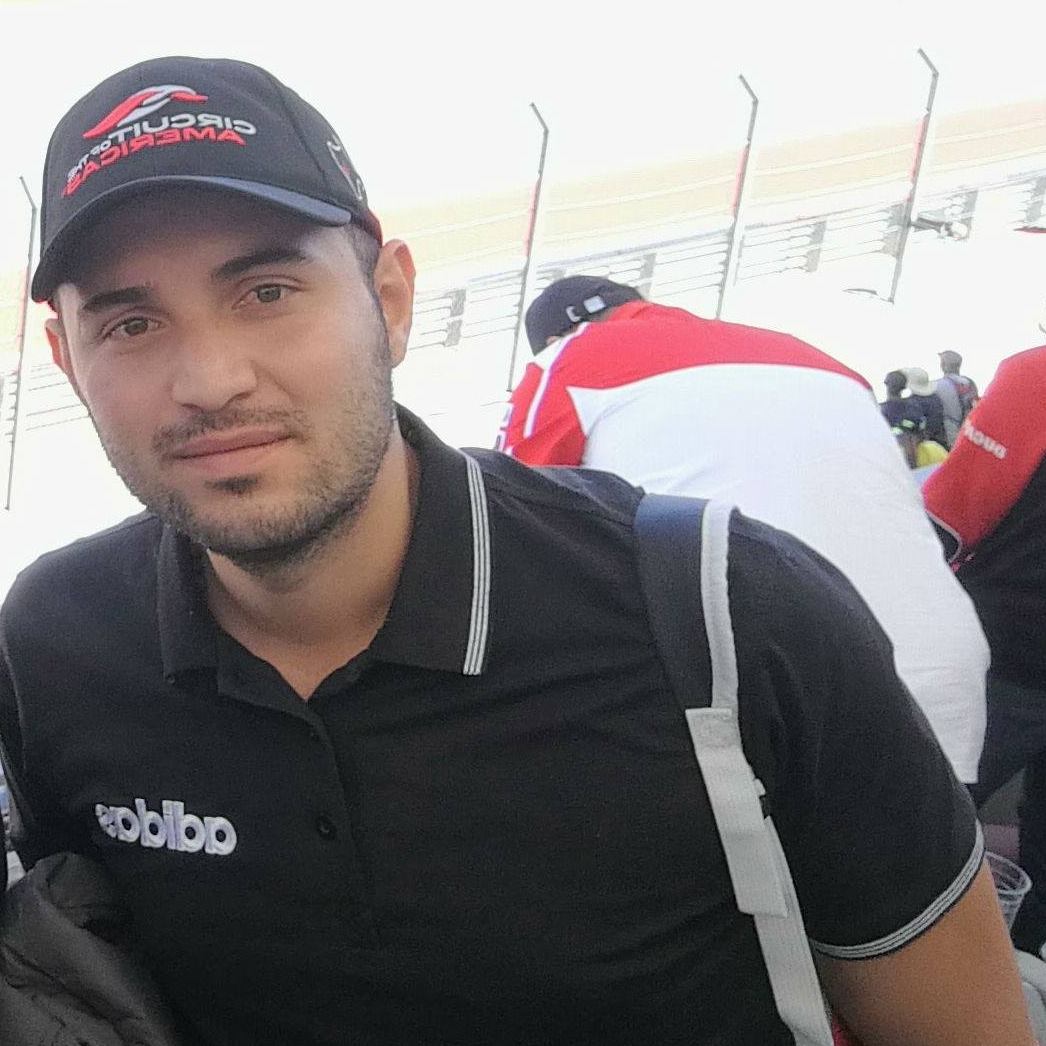
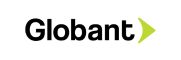