Symfony Caching: Boosting Performance with Cached Data
In today’s fast-paced digital world, users demand websites and applications that respond quickly and deliver a seamless user experience. Slow-loading pages can lead to frustrated users, increased bounce rates, and lost revenue opportunities. Symfony, being a robust PHP framework, offers various caching mechanisms to optimize performance and reduce the response time of web applications. In this blog, we will explore the world of Symfony caching and how it can significantly boost the performance of your Symfony applications. Let’s dive in!
1. Understanding Caching and Its Importance:
Caching is the process of storing frequently accessed data in a temporary storage space to serve future requests quickly. By doing so, we reduce the need for repetitive and resource-intensive computations or database queries, thus boosting the application’s response time and overall performance. Caching plays a crucial role in web development, especially when dealing with data that doesn’t change frequently.
2. Caching in Symfony:
Symfony provides a powerful caching system through its Cache Component, which enables developers to implement various caching strategies seamlessly. The Cache Component acts as an abstraction layer for different caching adapters, making it easy to switch between them based on project requirements.
2.1 The Cache Component:
At the core of Symfony’s caching capabilities is the Cache Component, which offers a simple and consistent API for working with different caching systems. Developers can leverage the Cache Component to store and retrieve data from the cache using a common interface.
2.2 Supported Cache Adapters:
Symfony supports various cache adapters, allowing developers to choose the best option for their projects. Some of the commonly used cache adapters include:
- ArrayAdapter: Suitable for development and testing environments, where data is stored in PHP arrays.
- FilesystemAdapter: Stores cache data in files on the server’s filesystem.
- ApcuAdapter: Utilizes the APCu extension to cache data in memory, providing faster access than file-based caching.
- RedisAdapter: Uses Redis, an in-memory data store, to cache data and support distributed caching across multiple servers.
- MemcachedAdapter: Utilizes Memcached, a high-performance, distributed memory caching system.
3. Cache Strategies and Best Practices:
Symfony caching offers several strategies to enhance application performance based on specific use cases. Understanding these strategies will help developers make informed decisions on which caching technique to use in different scenarios.
3.1 Page-Level Caching:
Page-level caching involves caching the entire rendered output of a webpage. This technique is effective for pages that are relatively static and don’t change frequently. Cached pages can be served quickly, reducing server load and improving response times for end-users.
3.2 Data-Level Caching:
Data-level caching involves caching specific pieces of data, such as database query results or API responses. By caching data at this level, Symfony can retrieve the data from the cache instead of repeating expensive computations or querying the database again, leading to faster responses.
3.3 Result Caching:
Result caching is closely related to data-level caching and is often used when working with Doctrine ORM or the QueryBuilder. It allows developers to cache the results of complex database queries, which can be particularly beneficial for queries that involve aggregations, joins, or complex filtering logic.
3.4 Edge Side Includes (ESI):
ESI caching is a technique that allows different parts of a webpage to be cached separately. With ESI, dynamic components of a page can be cached individually, while the main page remains cached as a whole. This enables faster loading times for dynamic elements without affecting the entire page cache.
3.5 Cache Invalidation:
Cache invalidation is a critical aspect of caching, ensuring that cached data is refreshed when it becomes stale or outdated. Symfony provides mechanisms to handle cache invalidation based on events, tags, or manual cache clearing.
4. Configuration and Setup:
Using Symfony caching in your projects requires some initial setup and configuration. Let’s walk through the steps to get started.
4.1 Installing the Cache Component:
Symfony’s Cache Component is a standalone package that can be installed via Composer. Open your terminal and run the following command to install the Cache Component:
bash composer require symfony/cache
4.2 Configuring Cache Adapters:
After installing the Cache Component, you need to configure the cache adapter you want to use. In your Symfony project’s configuration file (e.g., config/packages/framework.yaml), define the desired cache configuration:
yaml framework: cache: app: cache.adapter.apcu # Replace with the desired adapter (e.g., cache.adapter.filesystem, cache.adapter.redis, etc.)
Remember to adjust the configuration based on the cache adapter you want to use.
5. Code Examples:
Let’s explore some practical examples of using caching techniques in Symfony.
5.1 Simple Cache Usage:
The Cache Component provides an easy-to-use interface for caching arbitrary data. Here’s a simple example of caching an expensive computation:
php use Symfony\Component\Cache\Adapter\ApcuAdapter; use Symfony\Component\Cache\CacheItem; // Create the cache adapter $cache = new ApcuAdapter(); // Generate a cache key for the computation $cacheKey = 'expensive_computation_result'; // Check if the result exists in the cache if (!$cache->hasItem($cacheKey)) { // Perform the expensive computation $result = performExpensiveComputation(); // Cache the result $cacheItem = new CacheItem($cacheKey); $cacheItem->set($result); $cache->save($cacheItem); } else { // Retrieve the result from the cache $cacheItem = $cache->getItem($cacheKey); $result = $cacheItem->get(); } // Use the $result variable as needed
5.2 Page Caching Implementation:
To implement page-level caching, we can use Symfony’s built-in HTTP cache directives. This example demonstrates how to cache an entire page using HTTP caching:
php use Symfony\Component\HttpFoundation\Response; // Create a Response object $response = new Response(); // Check if the page is cached if ($response->isNotModified($request)) { // Return the cached response return $response; } // Perform page rendering and set appropriate headers $response->setContent(renderPage()); $response->setPublic(); $response->setMaxAge(3600); // Cache the page for one hour // Return the response return $response;
5.3 Data Caching with Doctrine:
Caching database query results with Doctrine can significantly improve performance. Here’s an example of caching query results:
php use Doctrine\ORM\EntityManagerInterface; use Symfony\Component\Cache\Adapter\ApcuAdapter; // Create the cache adapter $cache = new ApcuAdapter(); // Get the Doctrine EntityManager $entityManager = $this->getDoctrine()->getManager(); // Generate the cache key for the query $cacheKey = 'user_list_query_result'; // Check if the result exists in the cache if (!$cache->hasItem($cacheKey)) { // Perform the query and get the results $query = $entityManager->createQuery('SELECT u FROM App\Entity\User u'); $result = $query->getResult(); // Cache the result $cacheItem = $cache->getItem($cacheKey); $cacheItem->set($result); $cache->save($cacheItem); } else { // Retrieve the result from the cache $cacheItem = $cache->getItem($cacheKey); $result = $cacheItem->get(); } // Use the $result variable as needed
5.4 Result Caching with QueryBuilder:
For caching QueryBuilder results, we can leverage Symfony’s caching mechanism as follows:
php use Symfony\Component\Cache\Adapter\ApcuAdapter; // Create the cache adapter $cache = new ApcuAdapter(); // Get the Doctrine EntityManager $entityManager = $this->getDoctrine()->getManager(); // Generate the cache key for the QueryBuilder $cacheKey = 'complex_query_result'; // Check if the result exists in the cache if (!$cache->hasItem($cacheKey)) { // Build the query using the QueryBuilder $queryBuilder = $entityManager->createQueryBuilder(); $queryBuilder->select('p') ->from('App\Entity\Product', 'p') ->where('p.price > :price') ->setParameter('price', 100); // Execute the query and get the results $result = $queryBuilder->getQuery()->getResult(); // Cache the result $cacheItem = $cache->getItem($cacheKey); $cacheItem->set($result); $cache->save($cacheItem); } else { // Retrieve the result from the cache $cacheItem = $cache->getItem($cacheKey); $result = $cacheItem->get(); } // Use the $result variable as needed
5.5 ESI Caching for Dynamic Components:
ESI caching allows us to cache dynamic components of a page separately. Here’s an example of using ESI tags to cache a dynamic user widget on a Symfony template:
twig {# templates/user_widget.html.twig #} <h3>Welcome, {{ username }}!</h3> <p>Your account information goes here...</p> twig {# templates/main_page.html.twig #} <!DOCTYPE html> <html> <head> <title>Main Page</title> </head> <body> <h1>Main Page</h1> <esi:include src="/user_widget" /> <p>Other content of the main page...</p> </body> </html>
6. Monitoring and Clearing the Cache:
Monitoring the cache and clearing it when necessary is crucial for maintaining the performance of your Symfony application. Symfony provides a console command to clear the cache:
bash php bin/console cache:clear
Additionally, you can use monitoring tools or log entries to keep track of cache usage and performance.
7. Performance Testing and Benchmarks:
After implementing caching strategies, it’s essential to perform performance testing and benchmarks to measure the impact of caching on your Symfony application. Tools like Apache JMeter, Siege, or Blackfire can help you evaluate response times and identify potential bottlenecks.
Conclusion
Caching is a powerful technique to boost the performance of Symfony applications, reducing response times and enhancing the user experience. In this blog, we explored different caching strategies, including page-level caching, data-level caching, result caching, and ESI caching, and learned how to implement them in Symfony using the Cache Component. By integrating caching best practices into your Symfony projects, you can optimize the performance and scalability of your applications, meeting the demands of modern web users and staying ahead of the competition.
Remember, caching is not a one-size-fits-all solution, and the appropriate caching strategy depends on the nature of your application and its data. Continuously monitor and analyze your Symfony application’s performance to fine-tune caching settings and deliver an exceptional user experience. Happy caching!
Table of Contents
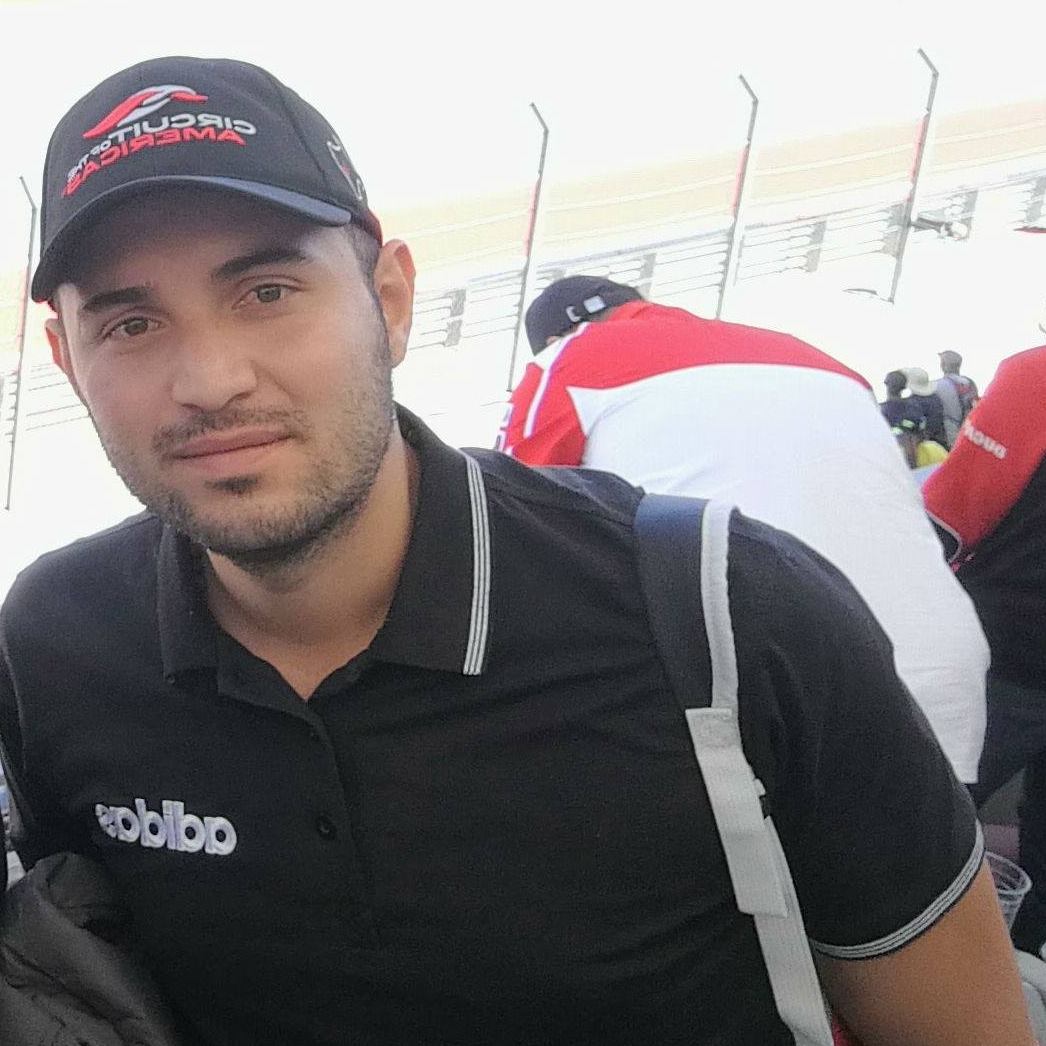
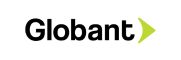