Symfony Console: Automating Command-Line Tasks
In today’s world of software development, automation plays a vital role in streamlining processes and increasing productivity. One area where automation can make a significant impact is command-line tasks. These tasks range from simple repetitive actions to complex operations that require multiple steps to be executed. Symfony Console is a powerful tool that enables developers to automate command-line tasks effortlessly. In this blog post, we will explore the features of Symfony Console, understand its commands, and provide code samples to help you get started.
1. Introduction to Symfony Console
Symfony Console is a component of the Symfony framework that provides a set of tools for building command-line applications. It simplifies the creation of command-line interfaces (CLIs) and enables developers to define and execute commands with ease. Symfony Console adheres to the Unix philosophy of building small, single-purpose tools that can work together, making it an excellent choice for automating command-line tasks.
2. Features of Symfony Console
Symfony Console comes with a wide range of features that make it a robust tool for automating command-line tasks. Some of the notable features include:
2.1. Command Creation and Execution
Symfony Console allows you to define and execute commands effortlessly. You can create custom commands by extending the Symfony\Component\Console\Command\Command class and implementing the necessary logic. These commands can be executed by running a single command-line statement, making it easy to automate complex tasks.
2.2. Input and Output Handling
Symfony Console provides built-in support for handling command-line input and output. It offers various input types such as arguments and options, which allow users to pass data to commands. The output can be customized and formatted using different styles, tables, progress bars, and colors, enhancing the readability and user experience of the command-line application.
2.3. Command Configuration
Symfony Console allows you to configure commands using annotations or YAML/XML configuration files. This configuration capability enables you to define command arguments, options, and other metadata directly in the command class or separate configuration files. It provides flexibility and maintainability for your command-line applications.
2.4. Command Testing
Testing is an essential part of any software development process. Symfony Console provides built-in tools and test helpers that facilitate the testing of your commands. You can simulate command execution, provide input, and assert the expected output, making it easier to ensure the correctness of your command-line tasks.
3. Getting Started with Symfony Console
To start using Symfony Console, you need to install it as a dependency in your project. You can use Composer, the PHP package manager, to manage your project dependencies. Open your terminal and run the following command:
bash composer require symfony/console
Once Symfony Console is installed, you can create a new command by extending the Command class. Let’s create a simple “hello” command that greets the user:
php use Symfony\Component\Console\Command\Command; use Symfony\Component\Console\Input\InputInterface; use Symfony\Component\Console\Output\OutputInterface; class HelloCommand extends Command { protected function configure() { $this->setName('hello') ->setDescription('Greet the user'); } protected function execute(InputInterface $input, OutputInterface $output) { $output->writeln('Hello, World!'); } }
In the code above, we define the configure method to set the command name and description. The execute method contains the logic to be executed when the command is run. In this case, it simply outputs “Hello, World!” to the console.
4. Running Symfony Console Commands
Once you have defined your command, you can execute it by running the Symfony Console application. Symfony Console provides a convenient way to bootstrap your command-line application and execute commands. Here’s an example:
php use Symfony\Component\Console\Application; $application = new Application(); $application->add(new HelloCommand()); $application->run();
In the code snippet above, we create a new instance of the Application class and register our HelloCommand with the application using the add method. Finally, we call the run method to execute the command.
To run the “hello” command, open your terminal and navigate to the project directory. Run the following command:
bash php console hello
You should see the output “Hello, World!” displayed on the console.
Conclusion
Symfony Console is a powerful tool for automating command-line tasks. Its rich feature set, ease of use, and extensibility make it an excellent choice for building robust and efficient command-line applications. In this blog post, we explored the features of Symfony Console, learned how to create and execute commands, and provided code samples to help you get started. By leveraging Symfony Console, you can automate your command-line tasks effectively and improve your development workflow. So why not give it a try and see how it can simplify your command-line operations? Happy automating!
Table of Contents
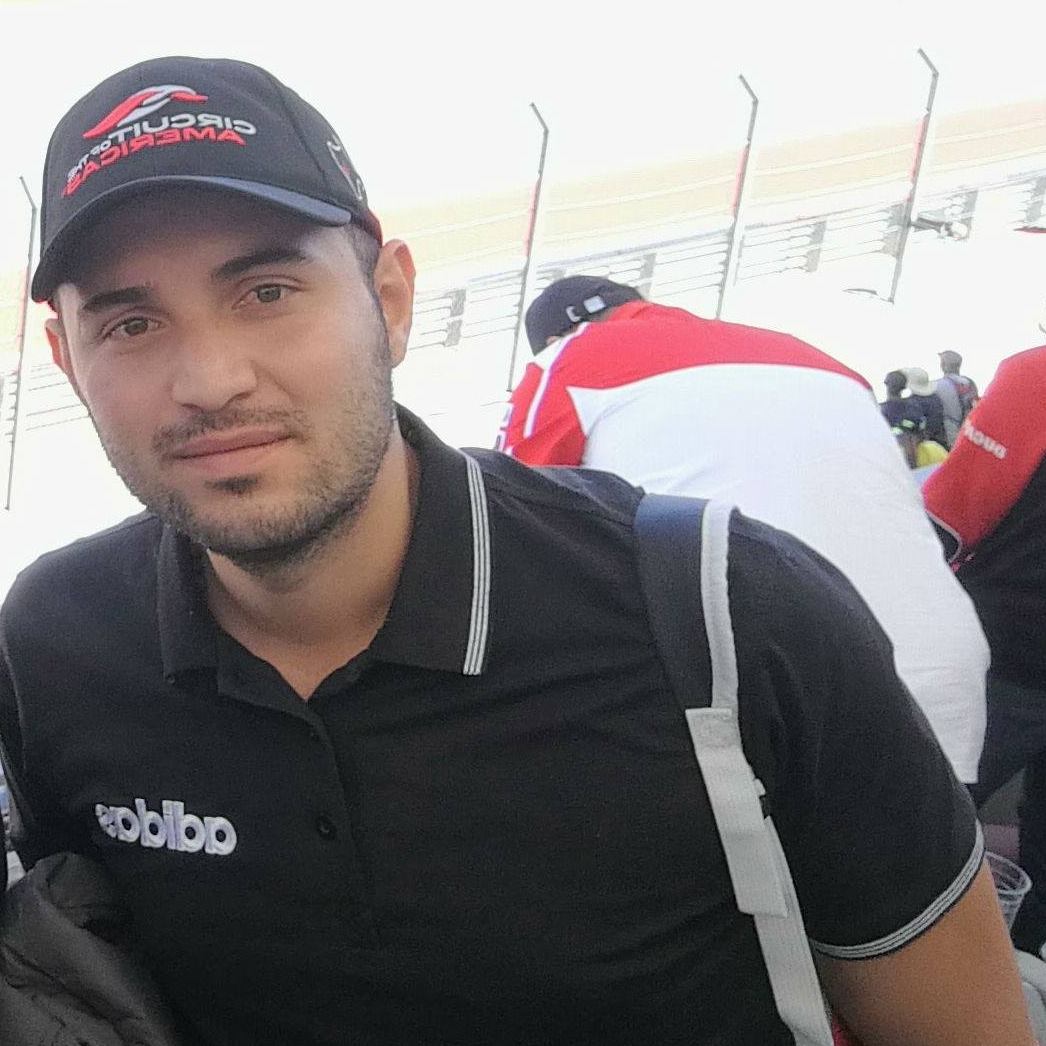
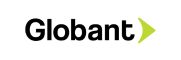