Creating Custom Twig Extensions in Symfony
Symfony is a robust and popular PHP web application framework that follows the MVC (Model-View-Controller) pattern. It utilizes Twig as its default template engine to separate the presentation layer from business logic. Twig is a flexible and powerful template language that simplifies the process of rendering dynamic content in Symfony applications.
However, there might be scenarios where the default Twig functions and filters provided by Symfony do not cover all your templating needs. To address these cases, Symfony allows you to create custom Twig extensions, which give you the freedom to add your own functions, filters, and global variables to Twig templates.
In this blog post, we will dive into the process of creating custom Twig extensions in Symfony. We will cover the following topics:
- What are Twig Extensions?
- Setting up a Symfony Project
- Creating a Custom Twig Extension
- Registering the Custom Extension with Symfony
- Using the Custom Twig Extension
- Advanced Features and Best Practices
Let’s get started!
1. What are Twig Extensions?
Twig extensions are reusable sets of functions, filters, or global variables that extend the capabilities of the Twig templating engine. With Twig extensions, you can encapsulate complex logic and make it available to your Twig templates in a clean and organized way.
Symfony provides a wide range of built-in Twig functions and filters. However, custom Twig extensions are necessary when you need to implement functionality specific to your application that is not available out of the box.
2. Setting up a Symfony Project
Before we start creating a custom Twig extension, ensure you have Symfony installed on your system. If not, you can follow the Symfony documentation for installation instructions.
Once Symfony is installed, create a new Symfony project using Symfony CLI:
bash symfony new my_project_name
Next, navigate to the project directory:
bash cd my_project_name
3. Creating a Custom Twig Extension
To create a custom Twig extension, follow these steps:
Step 1: Create the Extension Class
In Symfony, Twig extensions are regular PHP classes that implement the Twig_ExtensionInterface. Let’s create our custom Twig extension class. In the src/Twig directory, create a new file named CustomExtension.php:
php // src/Twig/CustomExtension.php namespace App\Twig; use Twig\Extension\AbstractExtension; use Twig\TwigFilter; use Twig\TwigFunction; class CustomExtension extends AbstractExtension { // Extension logic will be implemented here }
Step 2: Add Functions and Filters
Inside the CustomExtension class, you can add custom functions and filters. Functions are used to perform actions and return values, while filters modify the output of variables. Let’s add a sample function and filter:
php // src/Twig/CustomExtension.php namespace App\Twig; use Twig\Extension\AbstractExtension; use Twig\TwigFilter; use Twig\TwigFunction; class CustomExtension extends AbstractExtension { public function getFunctions(): array { return [ new TwigFunction('custom_function', [$this, 'customFunction']), ]; } public function getFilters(): array { return [ new TwigFilter('custom_filter', [$this, 'customFilter']), ]; } // Function logic public function customFunction($arg1, $arg2) { // Function implementation here // Return the result } // Filter logic public function customFilter($value) { // Filter implementation here // Return the modified value } }
Replace customFunction and customFilter with the actual implementation of your custom logic.
Step 3: Add Global Variables (Optional)
Optionally, you can also add global variables that are accessible in all Twig templates. Global variables are helpful for sharing data between templates without passing them explicitly.
php // src/Twig/CustomExtension.php namespace App\Twig; use Twig\Extension\AbstractExtension; ... class CustomExtension extends AbstractExtension { public function getGlobals(): array { return [ 'global_variable' => 'Some global value', ]; } }
Replace ‘Some global value’ with the value you want to make available as a global variable.
4. Registering the Custom Extension with Symfony
Now that we have created our custom Twig extension, we need to register it with Symfony’s service container. To do this, open the config/services.yaml file and add the following configuration:
yaml # config/services.yaml services: App\Twig\CustomExtension: tags: ['twig.extension']
This configuration tells Symfony to treat the CustomExtension class as a Twig extension.
5. Using the Custom Twig Extension
After registering the custom Twig extension, you can now use its functions, filters, and global variables in your Twig templates.
5.1. Using Functions:
In any Twig template, call the custom function like this:
twig {{ custom_function(arg1, arg2) }}
Replace custom_function with the actual name of your function, and provide the required arguments arg1 and arg2.
5.2. Using Filters:
You can apply the custom filter to a variable like this:
twig {{ some_variable|custom_filter }}
Replace custom_filter with the actual name of your filter and some_variable with the variable you want to modify.
5.3. Using Global Variables (if added):
Global variables can be accessed directly in your Twig templates:
twig {{ global_variable }}
Replace global_variable with the name of your global variable.
6. Advanced Features and Best Practices
Creating custom Twig extensions provides endless possibilities for enhancing your Symfony application’s templating capabilities. Here are some advanced features and best practices to consider:
6.1. Dependency Injection:
If your custom extension requires other services or dependencies, use Symfony’s dependency injection to inject them into the extension class. This ensures better decoupling and easier testing.
6.2. Performance Considerations:
Keep in mind that custom Twig functions and filters can impact the performance of your application, especially if they involve complex calculations or database queries. Use caching and other optimization techniques to ensure smooth performance.
6.3. Organizing Extensions:
As your Symfony application grows, you might have multiple custom Twig extensions. Organize them into separate files and directories to maintain a clean codebase.
6.4. Reusability:
Design your custom extensions to be reusable across different projects or even different parts of the same project. This improves code maintainability and reduces duplication.
6.5. Unit Testing:
To ensure the correctness of your custom Twig extensions, write unit tests for them. PHPUnit and Symfony’s built-in testing tools can help you with that.
Conclusion
Custom Twig extensions provide a powerful way to extend the functionality of Twig templates in Symfony applications. By following the steps outlined in this blog post, you can create reusable and efficient extensions to meet your specific templating needs. Remember to adhere to best practices and consider performance optimizations to create robust and high-performance custom Twig extensions for your Symfony projects. Happy coding!
Table of Contents
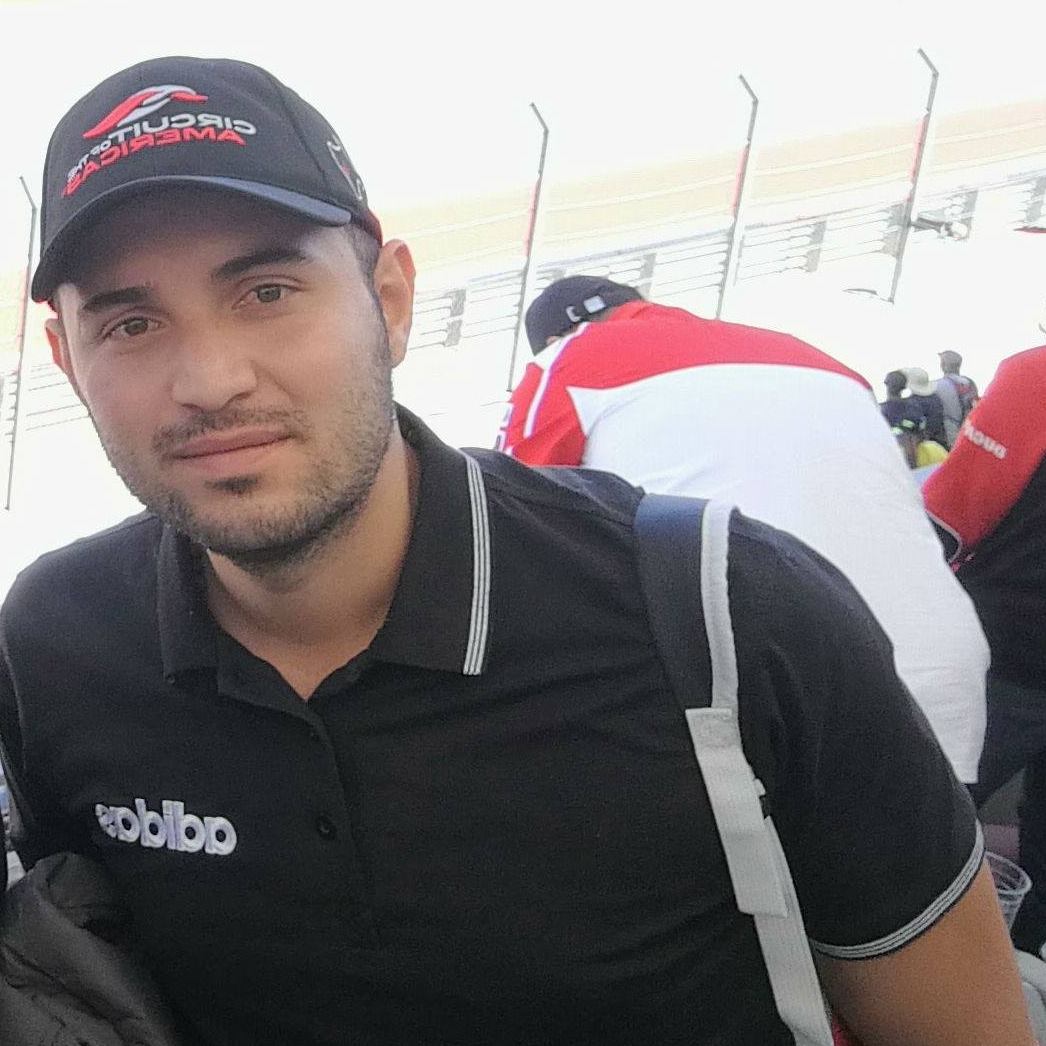
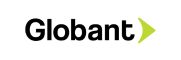