Understanding Symfony’s Dependency Injection Container
In modern software development, managing dependencies is a crucial aspect of building scalable and maintainable applications. Symfony, a widely used PHP framework, offers a robust Dependency Injection Container (DIC) that enables developers to efficiently manage their application’s dependencies. In this blog, we will dive deep into Symfony’s Dependency Injection Container, exploring its key concepts, advantages, and practical usage. Whether you’re a Symfony developer or just getting started with dependency injection, this article will equip you with essential knowledge to harness the full potential of Symfony’s DIC.
1. What is Dependency Injection?
1.1. Understanding Dependency Management:
In software development, dependencies refer to the relationships between different components of an application. A class or module often relies on other classes or objects to perform specific tasks, creating a dependency between them. Proper dependency management is crucial to achieve loose coupling, making the code more maintainable, testable, and extensible.
1.2. Benefits of Dependency Injection:
Dependency Injection (DI) is a design pattern that promotes the inversion of control by externalizing the creation and provision of dependencies. Instead of a class creating its dependencies internally, they are “injected” from the outside, reducing tight coupling and promoting modularity. This results in several benefits, including:
- Testability: Dependencies can be easily mocked or replaced during unit testing, making it easier to isolate components for testing.
- Flexibility: Swapping out implementations becomes effortless, allowing for easy adaptation to changing requirements.
- Modularity: Code becomes more organized, reusable, and easier to maintain.
- Single Responsibility Principle (SRP): Each class focuses on a single responsibility, leading to a cleaner and more maintainable codebase.
2. Symfony’s Dependency Injection Container (DIC)
2.1 What is a DIC?
Symfony’s Dependency Injection Container (DIC) is a powerful tool for managing and injecting dependencies into an application. The container acts as a centralized repository for all the services, allowing developers to define, configure, and retrieve them when needed. It automates the process of resolving dependencies and provides an elegant solution for inversion of control.
2.2. Why Use Symfony’s DIC?
Symfony’s DIC stands out from other dependency injection solutions due to its features and benefits, such as:
- Configuration Flexibility: It provides various configuration formats, including YAML, XML, and PHP, catering to developers’ preferences.
- Automatic Service Wiring: Symfony can automatically wire many services, reducing the need for explicit configuration in many cases.
- Service Tags: Symfony DIC allows developers to tag services and process them collectively through Compiler Passes, streamlining application logic.
- Performance: The DIC is optimized for speed, so the overhead of dependency resolution is minimized.
- Widely Adopted: Symfony’s DIC is used in many PHP projects, making it a valuable skill for developers working in the Symfony ecosystem.
3. Key Concepts of Symfony DIC
3.1. Services:
In Symfony DIC, a service is a reusable object representing a class instance or a shared resource. It is defined in the container and can be accessed and utilized throughout the application. Services are the building blocks of Symfony applications and are crucial for achieving modularity and maintainability.
3.2. Parameters:
Parameters are values that are not objects but are needed in the application’s configuration. They act as placeholders for various settings, such as database connection details, cache settings, etc. Parameters are useful for decoupling configuration from the code and making it more flexible.
3.3. Service Tags:
Service tags are markers attached to services that help to categorize and group them for further processing. Compiler Passes use these tags to perform actions on related services, such as adding them to event listeners or applying transformations.
3.4. Compiler Passes:
Compiler Passes are special classes that manipulate the container during the compilation process. They allow you to modify, remove, or add service definitions based on various conditions or criteria. Compiler Passes are useful for dynamic configuration and managing complex service dependencies.
4. Working with Symfony DIC
4.1. Installation and Configuration:
To start using Symfony’s DIC, you need to have a Symfony project set up. Install the required components by using Composer:
bash composer require symfony/dependency-injection
After installation, Symfony will automatically bootstrap the container, making it ready for use.
4.2. Defining Services:
In Symfony DIC, you define services using a configuration file. Symfony supports several formats, and we’ll use YAML for this example. Create a services.yaml file and define your services:
yaml # services.yaml services: app.mailer: class: App\Mailer arguments: - '@app.transport' - '%mailer.sender%'
In this example, we defined a service called app.mailer, which depends on another service app.transport and a parameter mailer.sender.
4.3. Resolving Services:
Once the services are defined, Symfony’s DIC handles the resolution of dependencies. To access a service, you can use the DIC’s get() method:
php $mailer = $container->get('app.mailer');
4.4. Constructor Injection:
Constructor injection is a common method of dependency injection, where dependencies are passed to a class through its constructor. Symfony DIC automatically resolves constructor dependencies when creating objects.
php class Mailer { private $transport; private $sender; public function __construct(Transport $transport, string $sender) { $this->transport = $transport; $this->sender = $sender; } // ... rest of the class }
4.5. Setter Injection:
Setter injection is another form of dependency injection, where dependencies are injected through setter methods. Symfony DIC can also resolve setter-based dependencies:
php class Mailer { private $transport; private $sender; public function setTransport(Transport $transport) { $this->transport = $transport; } public function setSender(string $sender) { $this->sender = $sender; } // ... rest of the class }
4.6. Configuring Service Tags:
Service tags allow you to categorize services for group processing. To tag a service, use the tags key in the service definition:
yaml # services.yaml services: app.listener.do_something: class: App\EventListener\DoSomethingListener tags: - { name: 'kernel.event_listener', event: 'some.event', method: 'onSomeEvent' }
In this example, we tagged the service app.listener.do_something as a kernel.event_listener with the event some.event and the method onSomeEvent.
4.7. Using Compiler Passes:
Compiler Passes enable advanced manipulation of the container during the compilation process. To create a Compiler Pass, implement the CompilerPassInterface:
php class CustomCompilerPass implements CompilerPassInterface { public function process(ContainerBuilder $container) { // Perform custom actions on the container, such as modifying or removing service definitions. } }
To register the Compiler Pass, add it to your bundle’s build() method:
php class AppBundle extends Bundle { public function build(ContainerBuilder $container) { parent::build($container); $container->addCompilerPass(new CustomCompilerPass()); } }
5. Best Practices and Tips
5.1. Keeping the Container Small:
While Symfony DIC provides immense flexibility, it’s essential to keep the container small and focused. Avoid overloading the container with too many services, as it may lead to performance issues and make the container harder to maintain.
5.2. Avoiding Service Locator Antipattern:
Service Locator is an anti-pattern that undermines the benefits of dependency injection. Avoid using the ContainerAwareInterface or directly accessing the container within your classes. Instead, inject only the required dependencies explicitly.
Conclusion
Symfony’s Dependency Injection Container is a powerful tool that simplifies dependency management and promotes modularity in PHP applications. By externalizing dependency creation and management, Symfony DIC adheres to the principles of inversion of control, making your code more maintainable, testable, and adaptable. With an array of features, including services, parameters, tags, and compiler passes, Symfony DIC empowers developers to build robust, scalable, and flexible applications.
In this blog, we explored the fundamental concepts of dependency injection, the advantages of Symfony DIC, and practical examples of defining and resolving services. Armed with this knowledge, you can now confidently leverage Symfony DIC to architect clean and efficient PHP applications. Embrace the power of dependency injection, and unlock the full potential of your Symfony projects. Happy coding!
Table of Contents
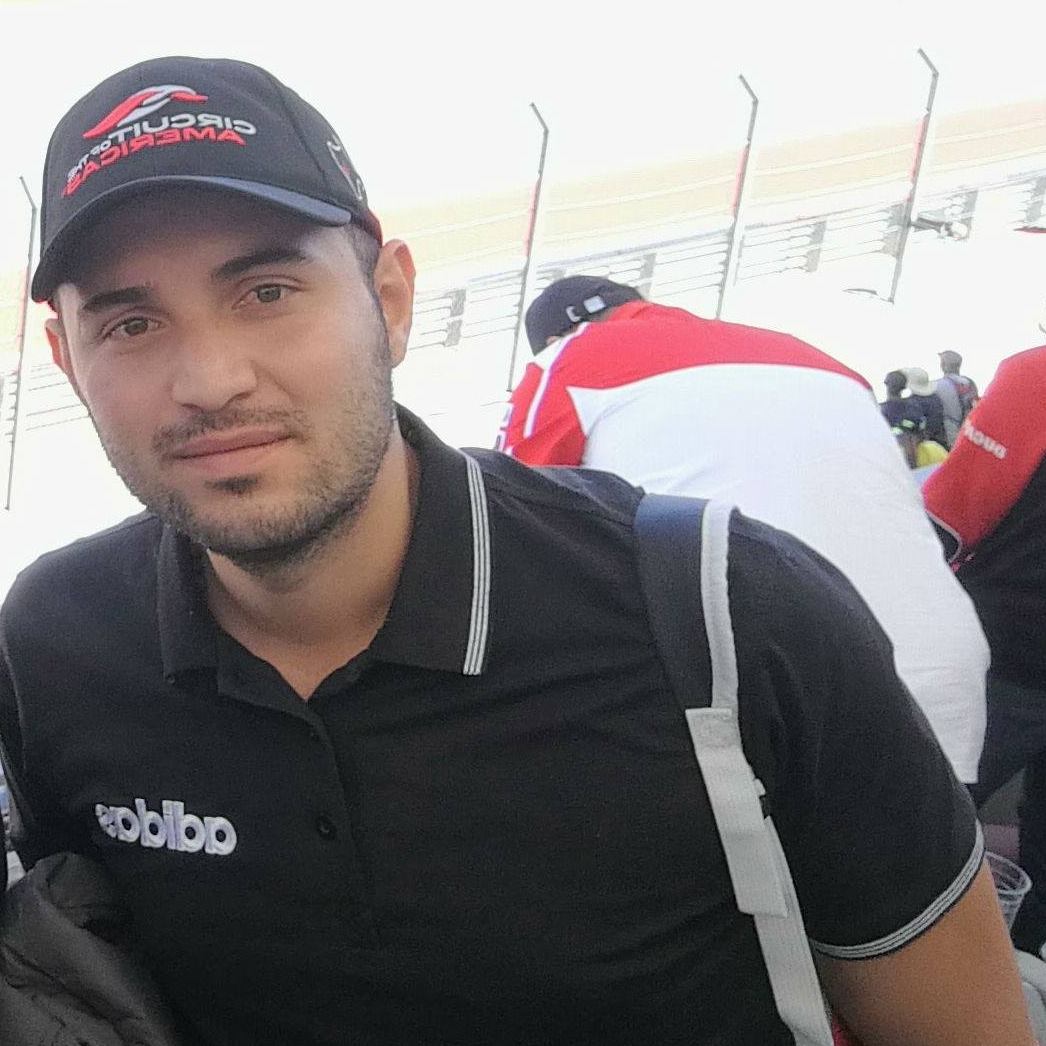
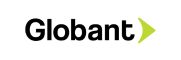