Exploring Symfony’s ORM: Doctrine
Symfony is a widely-used PHP web application framework that offers developers a robust set of tools to build scalable and maintainable applications. One of the most powerful components within Symfony is the Object-Relational Mapping (ORM) system, Doctrine. ORM simplifies database interactions by allowing developers to work with PHP objects instead of writing raw SQL queries. In this blog, we will dive deep into Symfony’s ORM: Doctrine, explore its core features, advantages, and demonstrate how to leverage it effectively to enhance your Symfony applications.
1. What is Symfony’s ORM: Doctrine?
Doctrine is an Object-Relational Mapping (ORM) system for PHP that enables developers to work with databases using object-oriented techniques. It is a powerful and flexible tool for managing database interactions, making it easier to build, query, and maintain databases in Symfony applications. Doctrine follows the Data Mapper pattern, which separates the domain logic from the persistence logic, resulting in a clean and maintainable codebase.
2. Key Features of Doctrine:
2.1. Entity Mapping:
At the core of Doctrine lies the concept of Entity Mapping. Entities are PHP classes that represent the structure of the database tables. By defining annotations or XML/YAML configuration, developers can map the properties of these entities to the corresponding columns in the database table. This abstraction allows you to work with databases using objects, making the code more intuitive and easier to understand.
Code Sample:
php // AppBundle/Entity/Product.php namespace AppBundle\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="products") */ class Product { /** * @ORM\Id * @ORM\GeneratedValue(strategy="AUTO") * @ORM\Column(type="integer") */ private $id; /** * @ORM\Column(type="string", length=255) */ private $name; // Other properties and methods... }
2.2. Query Language (DQL):
Doctrine Query Language (DQL) is a powerful and expressive language that allows you to perform database queries using the entity’s object-oriented syntax, rather than raw SQL. DQL abstracts away the database-specific details, making your queries more portable across different database systems.
Code Sample:
php // Fetch products whose price is greater than $100 $query = $entityManager->createQuery( 'SELECT p FROM AppBundle\Entity\Product p WHERE p.price > :price' )->setParameter('price', 100); $products = $query->getResult();
2.3. Associations and Relationships:
One of the most significant advantages of using an ORM like Doctrine is the ability to define and manage associations between entities easily. Doctrine supports various types of relationships, such as OneToOne, OneToMany, ManyToOne, and ManyToMany. These associations are defined using annotations, allowing you to build complex database schemas while maintaining the integrity of the data.
Code Sample:
php // AppBundle/Entity/Order.php namespace AppBundle\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="orders") */ class Order { // ... /** * @ORM\ManyToOne(targetEntity="AppBundle\Entity\Customer", inversedBy="orders") * @ORM\JoinColumn(name="customer_id", referencedColumnName="id") */ private $customer; // Other properties and methods... }
2.4. Lifecycle Events:
Doctrine provides lifecycle callbacks that allow you to hook into specific events that occur during the lifecycle of an entity, such as “prePersist,” “postLoad,” “preUpdate,” and more. This feature is handy for implementing business logic or performing additional operations on entities when certain events are triggered.
Code Sample:
php // AppBundle/Entity/Product.php namespace AppBundle\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="products") * @ORM\HasLifecycleCallbacks */ class Product { // ... /** * @ORM\PrePersist */ public function setCreatedAtValue() { $this->createdAt = new \DateTime(); } // Other properties and methods... }
2.5. Migrations:
As your application evolves, so will your database schema. With Doctrine Migrations, you can keep your database in sync with your entity classes by generating and executing migration scripts. Migrations provide a version control system for your database, allowing you to update your schema incrementally and efficiently.
Code Sample:
bash # Generate a new migration class php bin/console doctrine:migrations:diff # Execute pending migrations php bin/console doctrine:migrations:migrate
3. Setting up Doctrine in Symfony:
Before diving into using Doctrine, you need to ensure that it’s set up correctly in your Symfony project. Symfony’s standard edition comes with Doctrine pre-configured, but if you’re starting from scratch or using a different edition, you might need to configure it yourself.
Step 1: Install Doctrine via Composer
Open your terminal and navigate to your Symfony project’s root directory. Run the following command to install Doctrine and its dependencies:
bash composer require doctrine symfony/orm-pack
This command installs Doctrine along with the necessary Symfony bridge to make them work seamlessly together.
Step 2: Configure Doctrine
Symfony provides a configuration file (doctrine.yaml) where you can define your database connection and other settings. Locate the file in the config/packages directory and make sure it looks like this:
yaml # config/packages/doctrine.yaml doctrine: dbal: # configure your database connection here url: '%env(resolve:DATABASE_URL)%' orm: auto_generate_proxy_classes: true naming_strategy: doctrine.orm.naming_strategy.underscore auto_mapping: true
Here, we’re using environment variables to configure the database connection, making it easy to switch between different environments (e.g., development, staging, production) without modifying the configuration.
Step 3: Create the Database
After configuring Doctrine, you can create the database by running the following command:
bash php bin/console doctrine:database:create
4. Creating Entities and Entity Mapping:
Entities are the heart of Doctrine ORM. They represent the data in your application and are used to interact with the database. To create an entity, you simply create a PHP class and annotate it with the necessary metadata to map it to a database table.
Step 1: Creating the Entity Class
For instance, let’s create an entity class to represent a “Product” in our application. In the Entity directory, create a new file called Product.php with the following content:
php // src/Entity/Product.php namespace App\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="products") */ class Product { /** * @ORM\Id * @ORM\GeneratedValue(strategy="AUTO") * @ORM\Column(type="integer") */ private $id; /** * @ORM\Column(type="string", length=255) */ private $name; /** * @ORM\Column(type="float") */ private $price; // Getters and setters... }
5. Querying with DQL:
DQL is a powerful query language that allows you to perform complex database queries using an object-oriented syntax. Instead of writing raw SQL, you can write queries using the names of your entity classes and properties.
Step 1: Fetching Data with DQL
To fetch data from the database using DQL, you need to obtain the EntityManager instance and create a QueryBuilder. Then, you can specify the entity and properties to select, apply filters, and define any other criteria.
php // Fetch products whose price is greater than $100 $query = $entityManager->createQuery( 'SELECT p FROM App\Entity\Product p WHERE p.price > :price' )->setParameter('price', 100); $products = $query->getResult();
6. Working with Associations and Relationships:
One of the strengths of Doctrine is its support for defining and managing associations between entities. These associations can be OneToOne, OneToMany, ManyToOne, and ManyToMany, depending on the cardinality of the relationship.
Step 1: Defining Relationships
Let’s assume we have two entities, Order and Customer. An order belongs to a single customer (ManyToOne), and a customer can have multiple orders (OneToMany). Here’s how you define these relationships:
php // src/Entity/Order.php namespace App\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="orders") */ class Order { // ... /** * @ORM\ManyToOne(targetEntity="App\Entity\Customer", inversedBy="orders") * @ORM\JoinColumn(name="customer_id", referencedColumnName="id") */ private $customer; // Other properties and methods... } php // src/Entity/Customer.php namespace App\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="customers") */ class Customer { // ... /** * @ORM\OneToMany(targetEntity="App\Entity\Order", mappedBy="customer") */ private $orders; // Other properties and methods... }
7. Utilizing Doctrine’s Lifecycle Events:
Doctrine provides lifecycle callbacks that allow you to hook into specific events that occur during the lifecycle of an entity, such as “prePersist,” “postLoad,” “preUpdate,” and more. This feature is handy for implementing business logic or performing additional operations on entities when certain events are triggered.
Step 1: Defining Lifecycle Callbacks
Let’s say you want to set the creation date of a Product entity automatically when it’s persisted for the first time. You can achieve this using a prePersist lifecycle callback:
php // src/Entity/Product.php namespace App\Entity; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="products") * @ORM\HasLifecycleCallbacks */ class Product { // ... /** * @ORM\Column(type="datetime") */ private $createdAt; /** * @ORM\PrePersist */ public function setCreatedAtValue() { $this->createdAt = new \DateTime(); } // Other properties and methods... }
8. Managing Database Migrations:
As your application evolves, so will your database schema. With Doctrine Migrations, you can keep your database in sync with your entity classes by generating and executing migration scripts. Migrations provide a version control system for your database, allowing you to update your schema incrementally and efficiently.
Step 1: Generating Migrations
To generate a new migration, run the following command:
bash php bin/console doctrine:migrations:diff
This command compares the current state of your entity classes with the current state of the database and generates a migration file with the necessary SQL statements to update the schema.
Step 2: Executing Migrations
Once the migration file is generated, you can apply the changes to the database using the following command:
bash php bin/console doctrine:migrations:migrate
This command executes all pending migrations and updates the database schema accordingly.
9. Advantages of Symfony’s ORM: Doctrine:
Symfony’s ORM, Doctrine, provides numerous advantages that make it an excellent choice for managing database interactions in Symfony applications:
- Abstraction of Database Operations: Doctrine abstracts the low-level database operations, allowing developers to focus on the business logic and work with PHP objects instead of writing raw SQL queries.
- Portability: With DQL, you can write database queries using an object-oriented syntax, making them portable across different database systems.
- Maintainable Codebase: The separation of concerns provided by Doctrine’s Data Mapper pattern results in a clean and maintainable codebase.
- Associations and Relationships: Doctrine provides straightforward ways to define and manage relationships between entities, simplifying complex database schemas.
- Lifecycle Events: The lifecycle events in Doctrine offer the flexibility to execute custom logic during different stages of an entity’s life.
- Database Migrations: With Doctrine Migrations, you can manage your database schema efficiently and keep it in sync with your entity classes.
10. Best Practices for Using Doctrine Effectively:
- Keep Entities Clean and Focused: Entities should be clean representations of your application’s data. Avoid adding business logic or application-specific methods directly into the entity classes.
- Use Lazy Loading: Doctrine supports lazy loading, which means related entities are loaded only when accessed. Utilize this feature wisely to avoid unnecessary database queries.
- Optimize Queries: Be mindful of the queries generated by Doctrine, especially in performance-critical sections of your application. Use DQL efficiently to fetch only the necessary data.
- Stay Updated: Keep your Symfony and Doctrine versions up to date to benefit from the latest features and security updates.
- Logging and Debugging: Enable logging and debugging in your development environment to understand the generated queries and optimize them if needed.
Conclusion
Symfony’s ORM, Doctrine, is a powerful and flexible tool for managing database interactions in Symfony applications. By abstracting away the complexities of database operations, Doctrine allows developers to work with PHP objects and focus on building robust and maintainable applications. In this blog, we explored the core features of Doctrine, including entity mapping, DQL, associations, lifecycle events, and migrations. Armed with this knowledge, you can now leverage Doctrine effectively to enhance your Symfony projects, making them more scalable and efficient. Happy coding!
Table of Contents
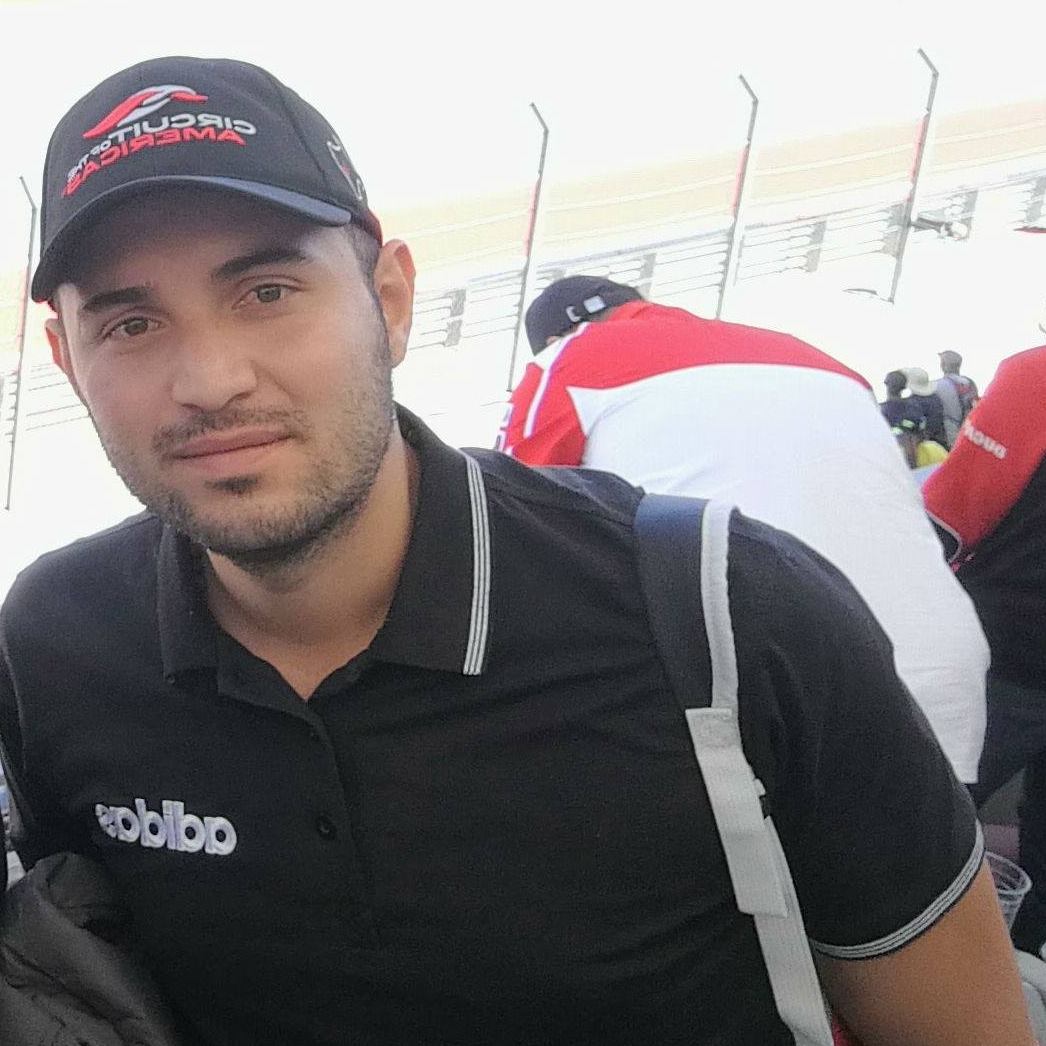
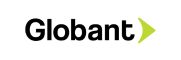