Using Symfony’s Event Dispatcher for Decoupled Architecture
In modern web development, creating applications with a decoupled architecture has become increasingly popular. Decoupled architecture separates components of a system, reducing interdependencies and improving maintainability, scalability, and flexibility. Symfony, a popular PHP framework, provides a powerful Event Dispatcher component that facilitates the implementation of decoupled architecture.
In this blog post, we will explore Symfony’s Event Dispatcher and its role in building a decoupled architecture. We’ll dive into the concept of event-driven programming, understand how Symfony’s Event Dispatcher works, and see practical examples of how it can be used to create a modular and flexible application.
Understanding Decoupled Architecture
Decoupled architecture, also known as a loosely coupled or event-driven architecture, involves designing a system where components can operate independently, communicating with each other through events and messages. Unlike a monolithic architecture, where components are tightly integrated, a decoupled architecture offers several advantages, such as:
- Modularity: Components can be developed and maintained independently, allowing for easy updates or replacements without affecting other parts of the system.
- Flexibility: The system can adapt to changing requirements more easily, as components are not tightly bound to each other.
- Scalability: Components can be distributed across different servers or microservices, enabling better resource utilization and improved performance.
- Testability: Isolated components can be tested independently, leading to more robust and comprehensive testing.
To implement decoupled architecture, we need a mechanism to manage the communication between components efficiently. This is where Symfony’s Event Dispatcher comes into play.
Introducing Symfony’s Event Dispatcher
Symfony’s Event Dispatcher is a powerful component that follows the Observer design pattern. It enables communication between different parts of an application without requiring them to know each other’s existence. The Event Dispatcher allows you to register event listeners (observers) to specific events, and when an event is dispatched, all registered listeners associated with that event are notified and can react accordingly.
Key Concepts of Symfony’s Event Dispatcher
Before we delve into practical examples, let’s understand some key concepts related to Symfony’s Event Dispatcher:
- Events: Events are simple PHP objects that represent something significant happening in the application. They can carry data relevant to the event and trigger actions based on that data.
- Event Listeners: Event listeners are PHP callables (usually, closures or methods) that respond to specific events. They are registered with the Event Dispatcher to listen for particular events and perform actions when those events are dispatched.
- Event Dispatcher: The Event Dispatcher is the central hub that manages the communication between events and event listeners. It holds a registry of event listeners and dispatches events to appropriate listeners when they occur.
Using Symfony’s Event Dispatcher in Practice
Now, let’s see how we can use Symfony’s Event Dispatcher to achieve a decoupled architecture in a practical example. Suppose we are building an e-commerce platform, and we want to send a notification to customers whenever a new product is added. Instead of coupling the notification logic directly with the product addition process, we can utilize the Event Dispatcher to handle this scenario.
Step 1: Set Up Symfony Event Dispatcher
First, make sure you have a Symfony project up and running. If you don’t have one, you can create it using Symfony’s installer or Composer.
Next, ensure that the Symfony Event Dispatcher component is installed. If it’s not already included in your project, you can add it via Composer:
bash composer require symfony/event-dispatcher
Step 2: Define the Events and Listeners
In this example, we will define two events: ProductAddedEvent and NotificationEvent.
Create the ProductAddedEvent class:
php // src/Event/ProductAddedEvent.php namespace App\Event; use Symfony\Contracts\EventDispatcher\Event; class ProductAddedEvent extends Event { public const NAME = 'product.added'; private $productId; public function __construct(int $productId) { $this->productId = $productId; } public function getProductId(): int { return $this->productId; } }
Create the NotificationEvent class:
php // src/Event/NotificationEvent.php namespace App\Event; use Symfony\Contracts\EventDispatcher\Event; class NotificationEvent extends Event { public const NAME = 'notification.send'; private $userId; private $message; public function __construct(int $userId, string $message) { $this->userId = $userId; $this->message = $message; } public function getUserId(): int { return $this->userId; } public function getMessage(): string { return $this->message; } }
Next, let’s create event listeners for these events. The listeners will handle the actual logic of sending notifications to users:
Create the ProductAddedListener class:
php // src/EventListener/ProductAddedListener.php namespace App\EventListener; use App\Event\ProductAddedEvent; use App\Event\NotificationEvent; class ProductAddedListener { public function onProductAdded(ProductAddedEvent $event) { $productId = $event->getProductId(); // Logic to handle product addition... // Send a notification about the new product $message = "A new product with ID $productId has been added."; $notificationEvent = new NotificationEvent(123, $message); return $notificationEvent; } }
Create the NotificationListener class:
php // src/EventListener/NotificationListener.php namespace App\EventListener; use App\Event\NotificationEvent; class NotificationListener { public function onNotificationSend(NotificationEvent $event) { $userId = $event->getUserId(); $message = $event->getMessage(); // Logic to send notification to the user... // For the sake of this example, we'll just print the notification message. echo "Sending notification to user $userId: $message\n"; } }
Step 3: Register the Event Listeners
After defining the events and their corresponding listeners, we need to register the listeners with the Event Dispatcher. Symfony provides a convenient way to do this through services and tags.
Configure the services in services.yaml:
yaml # config/services.yaml services: App\EventListener\ProductAddedListener: tags: - { name: kernel.event_listener, event: product.added, method: onProductAdded } App\EventListener\NotificationListener: tags: - { name: kernel.event_listener, event: notification.send, method: onNotificationSend }
In this configuration, the ProductAddedListener is tagged as a kernel.event_listener for the product.added event, and the NotificationListener is tagged for the notification.send event.
Step 4: Dispatch the Events
Finally, we can dispatch the events when appropriate. For instance, whenever a new product is added to the platform, we’ll dispatch the ProductAddedEvent:
php // src/Controller/ProductController.php namespace App\Controller; use App\Event\ProductAddedEvent; use Symfony\Component\EventDispatcher\EventDispatcherInterface; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route; class ProductController { /** * @Route("/product/add/{id}", name="add_product") */ public function addProduct(int $id, EventDispatcherInterface $eventDispatcher): Response { // Logic to add the product to the system... // For the sake of this example, we'll assume the product is successfully added. // Dispatch the ProductAddedEvent $event = new ProductAddedEvent($id); $eventDispatcher->dispatch($event, ProductAddedEvent::NAME); return new Response('Product added successfully!'); } }
When the ProductAddedEvent is dispatched, the ProductAddedListener will be triggered automatically, and it will, in turn, dispatch the NotificationEvent. The NotificationListener will handle this event, and, in our example, it will print the notification message.
Conclusion
Symfony’s Event Dispatcher is a robust tool that helps achieve a decoupled architecture by enabling efficient communication between different parts of an application. By utilizing events and event listeners, we can create modular, scalable, and flexible applications that are easy to maintain and test. This approach allows developers to build complex systems that can evolve with changing requirements without sacrificing stability.
In this blog post, we explored the basics of decoupled architecture, the key concepts of Symfony’s Event Dispatcher, and a practical example of using it to send notifications in an e-commerce platform. With Symfony’s Event Dispatcher in your toolbox, you can embrace the power of event-driven programming and take your application architecture to the next level of flexibility and extensibility. Happy coding!
Table of Contents
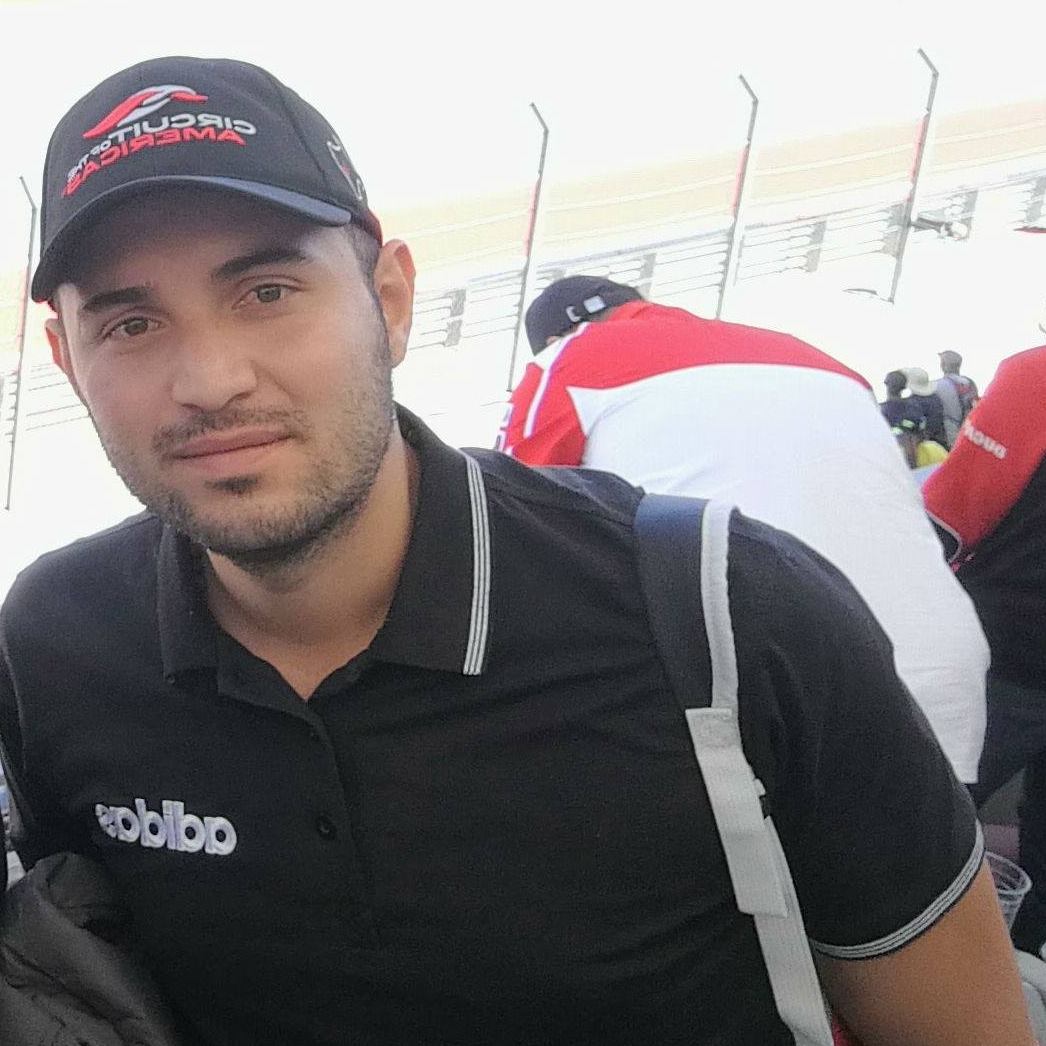
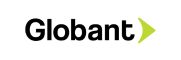