Mastering Symfony: A Comprehensive Guide for Beginners
Symfony is a powerful PHP framework that has gained immense popularity among developers worldwide. It provides a robust and flexible foundation for building web applications of any size and complexity. Whether you’re a beginner or an experienced developer, mastering Symfony can significantly enhance your skills and make you a sought-after professional in the industry.
In this comprehensive guide, we will take you through the fundamental concepts, best practices, and practical examples to help you become proficient in Symfony development. So, let’s dive into the world of Symfony and unlock its full potential!
1. Getting Started with Symfony
1.1 Installing Symfony
To begin your Symfony journey, you need to install the framework. Symfony provides a convenient installer that sets up everything you need for a new project. Open your terminal and run the following command:
shell $ composer create-project symfony/skeleton myproject
1.2 Symfony Directory Structure
Understanding the directory structure of a Symfony project is essential. Symfony follows a convention-over-configuration approach, providing a standardized layout. This structure makes it easier to organize your codebase and collaborate with other developers.
1.3 Creating Your First Symfony Project
Let’s create our first Symfony project. Use the following command to generate a new Symfony application:
shell $ symfony new myproject
1.4 Configuring Symfony
Symfony provides a flexible configuration system that allows you to customize various aspects of your application. You can configure services, routes, security, and much more using configuration files in YAML, XML, or PHP formats.
2. Understanding the MVC Architecture
Symfony follows the Model-View-Controller (MVC) architectural pattern. Understanding this pattern is crucial for building scalable and maintainable applications.
2.1 Model: Managing Data with Doctrine
Symfony integrates seamlessly with Doctrine, a powerful Object-Relational Mapping (ORM) tool. You will learn how to define database entities, perform database operations, and utilize Doctrine’s querying capabilities.
2.2 View: Templating with Twig
Twig is Symfony’s default templating engine, offering a clean and intuitive syntax. You will discover how to create dynamic views, use template inheritance, and leverage Twig’s powerful features.
2.3 Controller: Handling Requests and Responses
Controllers are responsible for processing requests, invoking the necessary logic, and generating responses. You will explore how to define controllers, handle routing, and manage different types of responses.
3. Routing and URL Generation
Routing is a fundamental concept in Symfony. It enables you to map incoming requests to specific controllers and actions. You will learn how to define routes, generate URLs, and work with route parameters and patterns.
3.1 Defining Routes in Symfony
Routes are defined using YAML, XML, or annotations. You will discover various techniques to define routes and handle dynamic segments in URLs.
3.2 Generating URLs in Symfony
Symfony provides powerful tools to generate URLs based on defined routes. You will explore how to generate URLs using different methods and handle query parameters.
3.3 Route Parameters and Patterns
Route parameters allow you to capture dynamic segments from URLs. You will learn how to define route patterns, constrain parameters, and create optional segments.
4. Working with Forms
Forms are a crucial part of web applications. Symfony offers a comprehensive Form component that simplifies form creation, validation, and handling form submissions.
4.1 Creating Forms in Symfony
You will learn how to create forms using Symfony’s Form component, including defining form fields, rendering forms, and handling form submissions.
4.2 Form Validation and Error Handling
Symfony provides powerful validation capabilities for ensuring data integrity. You will discover how to define validation rules, display validation errors, and handle form submission validation.
4.3 Handling Form Submissions
Handling form submissions involves processing data, performing additional operations, and redirecting users. You will explore how to handle form submissions, persist data, and display success messages.
5. Doctrine ORM and Database Management
Symfony integrates with Doctrine, a feature-rich ORM tool. You will learn how to define database entities, establish relationships between entities, and perform common database operations.
5.1 Introduction to Doctrine ORM
Gain a deep understanding of Doctrine’s core concepts, such as entities, repositories, and associations. You will explore how to use Doctrine annotations for entity mapping and generate database schemas.
5.2 Creating Database Entities
Define and configure entities that represent database tables. You will learn about different types of entity relationships and how to define them in Doctrine.
5.3 Querying the Database with Doctrine
Leverage Doctrine’s powerful query builder and DQL (Doctrine Query Language) to fetch and manipulate data from the database. You will learn how to perform basic and advanced database queries using Doctrine.
6. Services and Dependency Injection
Symfony follows the principles of dependency injection and provides a powerful service container to manage dependencies efficiently.
6.1 Understanding Services in Symfony
Discover the concept of services in Symfony and how they promote modular and reusable code. You will learn how to define services, inject dependencies, and leverage service autowiring.
6.2 Dependency Injection Container
Symfony’s Dependency Injection Container allows you to manage service dependencies and control their instantiation. You will explore how to configure services, use service tags, and benefit from autowiring.
6.3 Autowiring and Autoconfiguration
Symfony’s autowiring feature automatically resolves and injects dependencies without explicitly defining them. You will learn how to leverage autowiring and explore autoconfiguration options.
7. Security and Authentication
Learn how to secure your Symfony applications and implement user authentication and authorization.
7.1 Configuring Security in Symfony
Symfony provides a flexible security component that allows you to define access control rules, configure authentication providers, and manage firewalls. You will explore various security configuration options.
7.2 User Authentication and Authorization
Implement user authentication using Symfony’s built-in authentication providers, such as form-based login, token-based authentication, and OAuth. You will also learn how to define authorization rules to restrict access to certain resources.
7.3 Securing Routes and Resources
Secure specific routes and resources based on user roles and permissions. You will discover how to use access control rules to restrict access and implement custom authorization checks.
8. Testing and Debugging Symfony Applications
Testing and debugging are essential parts of the development process. Symfony provides powerful tools and techniques to ensure the quality and stability of your applications.
8.1 Unit Testing with PHPUnit
Learn how to write unit tests for your Symfony codebase using PHPUnit. You will explore different testing strategies, mocking dependencies, and asserting expected behaviors.
8.2 Functional Testing with Symfony’s WebTestCase
Symfony provides a functional testing framework that allows you to simulate HTTP requests and test your application’s behavior. You will learn how to write functional tests for controllers, forms, and security features.
8.3 Debugging Symfony Applications
Discover various debugging techniques and tools to identify and fix issues in your Symfony applications. You will explore the Symfony Profiler, logging, and using Xdebug for advanced debugging scenarios.
9. Advanced Symfony Concepts
Explore advanced concepts and techniques to take your Symfony skills to the next level.
9.1 Event Dispatching and Listeners
Symfony’s event system allows you to decouple components and handle specific actions based on events. You will learn how to create and dispatch events, as well as define event listeners and subscribers.
9.2 Services Tags and Compiler Passes
Discover how to use service tags and compiler passes to dynamically modify the behavior of services and extend Symfony’s core functionality. You will learn how to create and configure compiler passes and use service tags effectively.
9.3 Caching Strategies in Symfony
Caching can significantly improve the performance of your Symfony applications. You will explore various caching strategies, such as HTTP caching, database query caching, and using external cache systems like Redis or Memcached.
Conclusion
Congratulations! You have completed the comprehensive guide to mastering Symfony for beginners. Throughout this journey, you have gained a solid understanding of Symfony’s core concepts, best practices, and advanced techniques. Remember to practice and build real-world applications to solidify your skills. Symfony is a powerful framework that can unlock endless possibilities, and with dedication and continuous learning, you can become a proficient Symfony developer. Happy coding!
Table of Contents
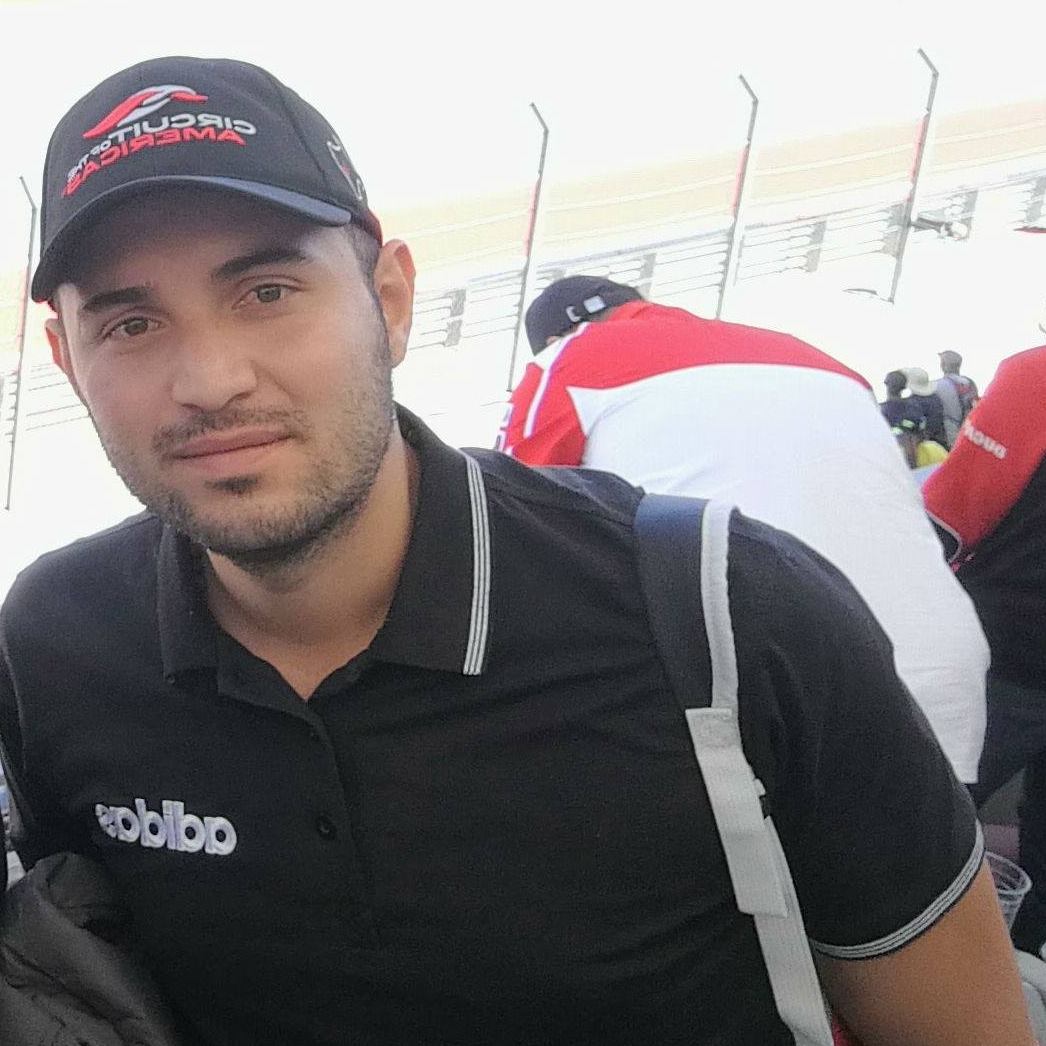
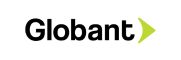