Hire Symfony Developers Interview Questions Guide
Symfony, a robust PHP framework, is widely used for building scalable and maintainable web applications. When aiming to recruit Symfony developers, it’s essential to assess their proficiency in Symfony, PHP, and related technologies. This guide equips you with the tools to navigate the hiring process, allowing you to evaluate candidates effectively and select the best fit for your team.
Table of Contents
1. How to Hire Symfony Developers
Embark on your journey to hire Symfony developers with these strategic steps:
1.1 Job Requirements
Define clear job prerequisites, outlining the specific Symfony versions, PHP versions, and other related technologies relevant to your project.
1.2 Search Channels
Utilize various channels like job boards, professional networks, and Symfony-related communities to discover potential candidates with the right skills and experience.
1.3 Screening
Scrutinize candidates’ Symfony expertise, examining their experience with Symfony components, bundles, and best practices.
1.4 Technical Assessment
Develop a comprehensive technical assessment, including coding challenges and problem-solving scenarios to gauge candidates’ Symfony development skills.
2. Core Skills of Symfony Developers to Look For
When evaluating Symfony developers, focus on these core skills:
- Symfony Framework Proficiency: A deep understanding of Symfony components, bundles, and the overall framework structure.
- PHP Expertise: Solid knowledge of PHP, including object-oriented programming and understanding PHP versions compatible with Symfony.
- Database Integration: Experience with Symfony Doctrine for seamless integration with databases.
- Twig Templating Engine: Proficiency in using the Twig templating engine for efficient and maintainable view layer code.
- API Development: Familiarity with building and consuming APIs using Symfony for creating robust and flexible applications.
- Testing: Experience with Symfony’s testing tools for unit and functional testing.
3. Overview of the Symfony Developer Hiring Process
Navigate the Symfony developer hiring process with these key steps:
3.1 Defining Job Requirements and Skillsets
Clearly define job requirements, specifying Symfony versions, PHP versions, and other essential skills needed for your project.
3.2 Crafting Compelling Job Descriptions
Create job descriptions that accurately convey the role, emphasizing the Symfony-specific skills required.
3.3 Crafting Symfony Developer Interview Questions
Develop a comprehensive set of interview questions covering Symfony intricacies, best practices, and real-world problem-solving scenarios.
4. Sample Symfony Developer Interview Questions and Answers
Explore these sample questions to assess candidates’ Symfony skills:
Q1. Explain the Symfony Event Dispatcher. How does it facilitate communication between components?
A: The Symfony Event Dispatcher is a component that allows decoupled components to communicate. It dispatches events and allows listeners to respond to those events. For example:
// Dispatching an event $event = new SomeEvent(); $dispatcher->dispatch(SomeEvent::NAME, $event); // Listening to an event $dispatcher->addListener(SomeEvent::NAME, [$this, 'onSomeEvent']);
Q2. Describe the Symfony service container. How does dependency injection work in Symfony?
A: The Symfony service container is a centralized place to manage services in a Symfony application. Dependency injection involves injecting a service into another, facilitating loose coupling. For example:
class SomeService { private $dependency; public function __construct(Dependency $dependency) { $this->dependency = $dependency; } }
Q3. How does Symfony handle routing, and what is the role of the routing configuration file?
A: Symfony uses a routing configuration file (usually routes.yaml or annotations) to define URL patterns and map them to controllers. For example:
# routes.yaml home: path: / controller: App\Controller\HomeController::index
Q4. Explain Symfony security components and their role in web application development.
A: Symfony security components provide tools for authentication, authorization, and other security-related tasks. The firewall, for instance, is configured to control access to parts of the application based on user roles.
Q5. Implement a Symfony form that collects user data, validates it, and persists it to the database.
A:
// In a controller action public function createUser(Request $request, EntityManagerInterface $entityManager) { $user = new User(); $form = $this->createForm(UserType::class, $user); $form->handleRequest($request); if ($form->isSubmitted() && $form->isValid()) { $entityManager->persist($user); $entityManager->flush(); } // ... }
Q6: Implement a Symfony Controller action that retrieves data from a Doctrine entity and returns it as a JSON response.
A:
// Controller action use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; use Symfony\Component\HttpFoundation\JsonResponse; use Symfony\Component\Routing\Annotation\Route; class ApiController extends AbstractController { /** * @Route("/api/data", name="api_data") */ public function getData() { $entityManager = $this->getDoctrine()->getManager(); $dataRepository = $entityManager->getRepository(Data::class); $data = $dataRepository->findAll(); $serializedData = $this->get('serializer')->serialize($data, 'json'); return new JsonResponse($serializedData, 200, [], true); } }
Q7: Create a Symfony Form Type that handles the submission of a user registration form with fields for username, email, and password.
A:
// Form Type use Symfony\Component\Form\AbstractType; use Symfony\Component\Form\FormBuilderInterface; use Symfony\Component\OptionsResolver\OptionsResolver; use Symfony\Component\Form\Extension\Core\Type\TextType; use Symfony\Component\Form\Extension\Core\Type\EmailType; use Symfony\Component\Form\Extension\Core\Type\PasswordType; class RegistrationFormType extends AbstractType { public function buildForm(FormBuilderInterface $builder, array $options) { $builder ->add('username', TextType::class) ->add('email', EmailType::class) ->add('password', PasswordType::class); } public function configureOptions(OptionsResolver $resolver) { $resolver->setDefaults([ // Configure your form options here ]); } }
Q8: Implement a Symfony Console Command that interacts with a service to perform a specific task, e.g., sending notification emails.
A:
// Console Command use Symfony\Component\Console\Command\Command; use Symfony\Component\Console\Input\InputInterface; use Symfony\Component\Console\Output\OutputInterface; class SendNotificationCommand extends Command { protected static $defaultName = 'app:send-notification'; protected function configure() { // Command configuration } protected function execute(InputInterface $input, OutputInterface $output) { $notificationService = $this->getContainer()->get('app.notification_service'); $notificationService->sendNotifications(); $output->writeln('Notifications sent successfully.'); return Command::SUCCESS; } }
Q9: Write a Symfony Validator constraint that ensures a field value is unique in the database.
A:
// Validator Constraint use Symfony\Component\Validator\Constraint; /** * @Annotation */ class UniqueField extends Constraint { public $message = 'The value "{{ value }}" is already taken.'; }
Q10: Create a Symfony Event Listener that logs an event when a user registers in the system.
A:
// Event Listener use Symfony\Component\EventDispatcher\EventSubscriberInterface; use Symfony\Component\Security\Http\Event\InteractiveLoginEvent; use Symfony\Component\Security\Http\SecurityEvents; use Psr\Log\LoggerInterface; class UserRegistrationListener implements EventSubscriberInterface { private $logger; public function __construct(LoggerInterface $logger) { $this->logger = $logger; } public static function getSubscribedEvents() { return [ SecurityEvents::INTERACTIVE_LOGIN => 'onInteractiveLogin', ]; } public function onInteractiveLogin(InteractiveLoginEvent $event) { $user = $event->getAuthenticationToken()->getUser(); $this->logger->info('User registered: ' . $user->getUsername()); } }
These questions and sample answers aim to assess a candidate’s practical skills in Symfony development. Candidates should be encouraged to explain their code choices, handle edge cases, and discuss potential improvements or optimizations.
5. Hiring Symfony Developers through CloudDevs
Step 1: Connect with CloudDevs
Initiate a dialogue with a CloudDevs consultant to delve into your project requirements, preferred skills, and desired experience. CloudDevs focuses on understanding your unique needs to streamline the Symfony developer selection process.
Step 2: Discover Your Ideal Match
In a swift turnaround, CloudDevs presents a curated selection of Symfony developers from their meticulously vetted pool of professionals. Dive into their profiles, assessing expertise and experience, and choose the candidate whose skills align seamlessly with your project’s vision.
Step 3: Embark on a Risk-Free Trial
Engage in meaningful conversations with your chosen Symfony developer to ensure a harmonious onboarding process. Once confidence is established, formalize the collaboration and kickstart a week-long risk-free trial. CloudDevs facilitates this trial period, allowing you to witness firsthand how their Symfony developers contribute to your project’s success.
By tapping into the capabilities of CloudDevs, you effortlessly pinpoint and onboard exceptional Symfony developers, guaranteeing your team possesses the skills necessary to craft outstanding web applications.
6. Conclusion
With these interview questions and insights, you’re now well-prepared to assess Symfony developers comprehensively. Whether you’re building web applications or APIs, securing the right Symfony developers for your team is crucial to the success of your projects.
Table of Contents
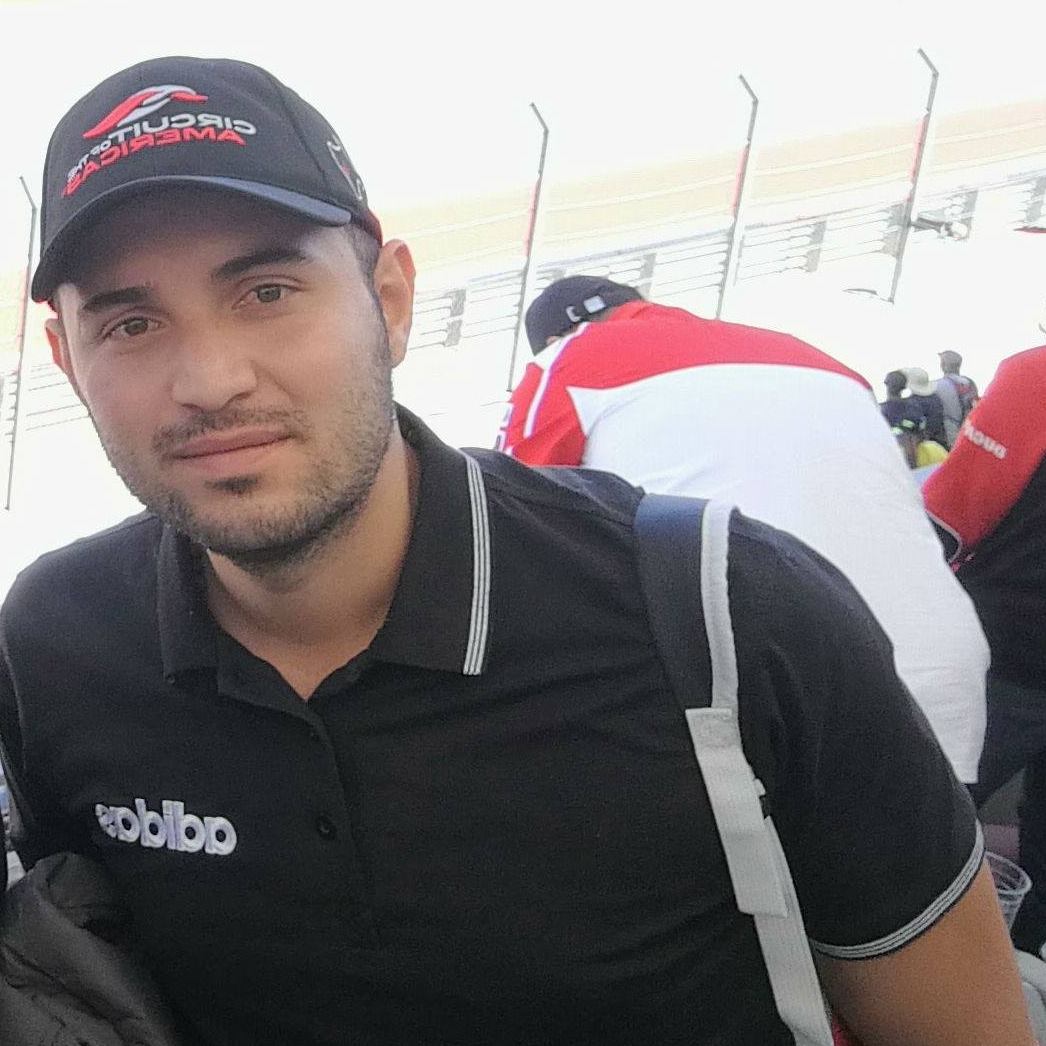
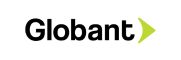