Optimizing Performance in Symfony Applications
Symfony is a powerful PHP framework known for its flexibility and scalability. However, as your Symfony application grows in complexity and user base, ensuring optimal performance becomes crucial. Slow-loading pages and high resource consumption can negatively impact user experience and deter potential customers.
In this blog, we will explore essential strategies to optimize the performance of Symfony applications. From writing efficient database queries to implementing caching mechanisms, these techniques will help you deliver a blazing-fast and responsive application.
1. Profiling and Benchmarking
Before diving into optimizations, it’s crucial to identify performance bottlenecks. Symfony provides robust tools like the Symfony Profiler and Blackfire.io for profiling and benchmarking. The profiler gives you insights into the time and resources consumed by different parts of your application.
2. Optimize Database Queries
1. Use Indexes: Ensure that your database tables are properly indexed to improve query performance. Analyze your application’s most frequent and slowest queries and add indexes on the columns used in the WHERE clause or JOIN conditions.
2. Avoid N+1 Problem: The N+1 problem occurs when you retrieve a collection of entities along with their related entities, resulting in a large number of database queries. Use Symfony’s Doctrine’s “eager loading” or “fetch-join” to fetch all required data in a single query, mitigating this issue.
Code Sample: Eager Loading
php // Before optimization $posts = $this->getDoctrine()->getRepository(Post::class)->findAll(); foreach ($posts as $post) { $authorName = $post->getAuthor()->getName(); } // After optimization $posts = $this->getDoctrine()->getRepository(Post::class)->findAllWithAuthorData();
3. Query Optimization: Ensure your queries are optimized and avoid unnecessary selects and joins. Use Symfony’s query builder wisely and leverage features like “select” only the required columns and “where” conditions to filter results effectively.
3. Caching Strategies
1. Symfony Cache Component: Utilize Symfony’s built-in Cache component to cache various parts of your application. Caching expensive operations like database queries or complex computations can significantly boost performance.
Code Sample: Caching in Symfony
php use Symfony\Component\Cache\Adapter\FilesystemAdapter; // Retrieve from cache $cache = new FilesystemAdapter(); $key = 'my_unique_key'; if ($cache->hasItem($key)) { $result = $cache->getItem($key)->get(); } else { // If not in cache, compute and store in cache $result = // perform expensive operation $cache->getItem($key)->set($result); $cache->save(); }
2. HTTP Caching: Leverage HTTP caching by setting proper cache headers (e.g., Last-Modified, ETag) for responses. This allows the client to cache the response, reducing unnecessary requests to the server.
4. Optimize Autoloading
1. Classmap Autoloading: In Symfony, you can use classmap autoloading to preload all the classes in your application into a single file, reducing the number of file inclusions during runtime.
Code Sample: Classmap Autoloading
Modify composer.json:
json { "autoload": { "classmap": ["src/"] } }
Then run: composer dump-autoload –optimize
2. PSR-4 Autoloading: Utilize PSR-4 autoloading efficiently. Avoid placing too many classes in a single namespace directory to prevent performance degradation.
5. Optimize Configuration
Remove Unused Services: Review your Symfony services configuration and remove any unused or unnecessary services. Keeping your service container lightweight will lead to faster boot times.
Use Environment Variables: Avoid hardcoding configuration values and use environment variables. Symfony’s parameter handling allows you to define environment-specific values.
6. HTTP/2 and CDN Usage
Upgrade to HTTP/2: If your server and client support HTTP/2, upgrade from HTTP/1.1 to take advantage of its improvements in multiplexing and parallelism, resulting in faster page loading times.
Content Delivery Network (CDN): Implement a CDN to serve static assets (e.g., images, stylesheets, and scripts) from servers closer to the user, reducing latency and download times.
7. Optimize Twig Templates
1. Cache Twig Templates: Enable Twig template caching in production to avoid the overhead of parsing templates on every request.
Code Sample: Enable Twig Caching
yaml # config/packages/twig.yaml twig: cache: '%kernel.cache_dir%/twig'
2. Reduce Template Complexity: Keep your Twig templates simple and avoid heavy logic or database queries within templates. Instead, pass the required data from the controller.
8. Optimize Asset Management
1. Asset Versioning: Add a version number to your assets (e.g., CSS, JS files) to leverage browser caching and ensure users receive the latest versions when changes are made.
Code Sample: Asset Versioning
twig <link rel="stylesheet" href="{{ asset('build/style.css') ~ '?v=' ~ asset_version('build/style.css') }}">
2. Minification and Compression: Minify your CSS and JavaScript files to remove unnecessary whitespace and comments. Additionally, enable gzip compression on the server to reduce the size of assets during transmission.
IX. Use OPCache
OPCache is a PHP extension that caches precompiled script bytecode in shared memory, reducing the time taken to parse and compile PHP files on each request.
Code Sample: Enable OPCache
Modify your php.ini or php.ini file:
ini [opcache] opcache.enable=1 opcache.enable_cli=1
Conclusion
Optimizing performance is an ongoing process, especially as your Symfony application evolves. By employing the strategies discussed in this blog, you can significantly enhance the speed and responsiveness of your Symfony application. Profiling, caching, database query optimization, and other techniques together can lead to a smooth user experience and improved overall application performance. Continuously monitor your application’s performance and iterate on the optimizations to ensure your Symfony application runs efficiently even as it scales to meet user demands.
Table of Contents
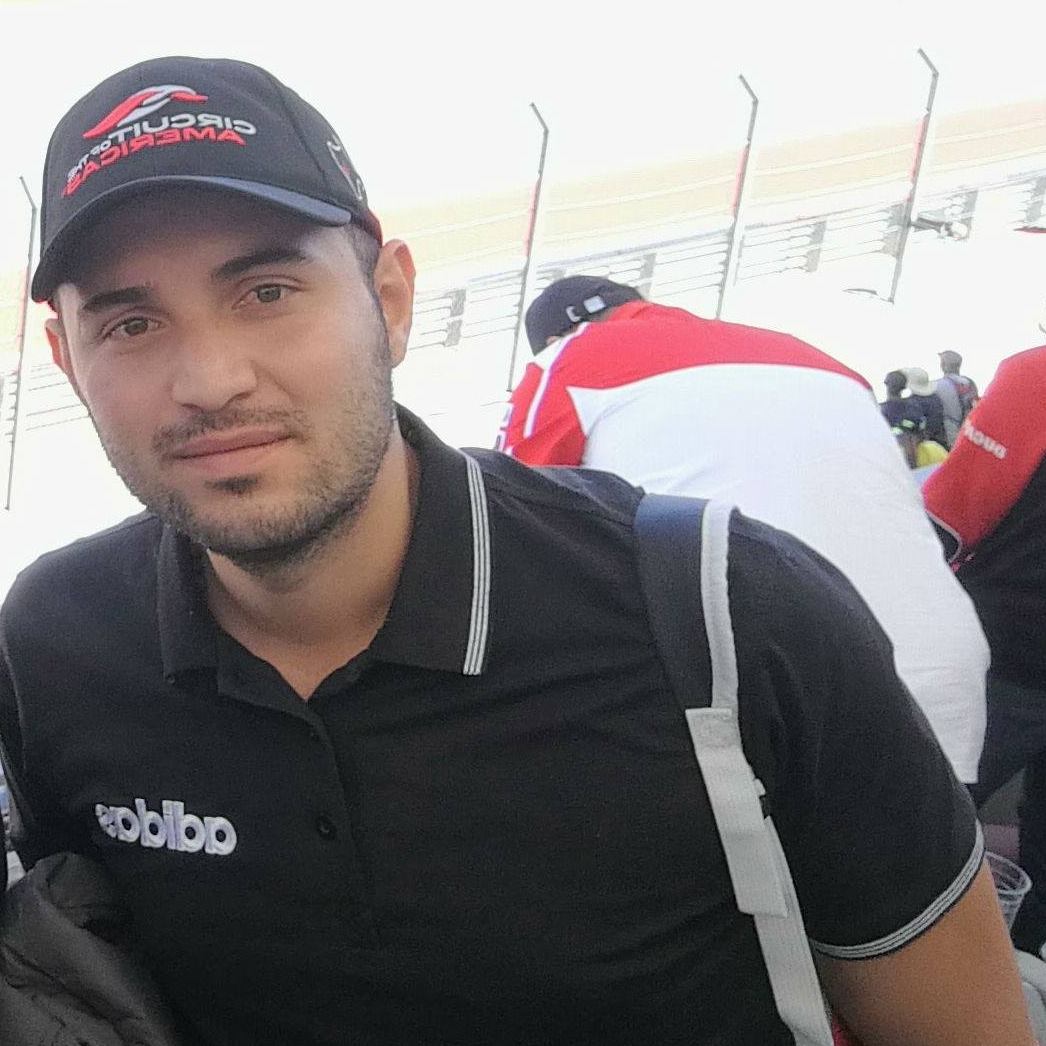
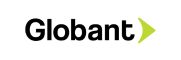