Exploring Symfony’s Powerful Routing System
Symfony is a widely adopted PHP framework known for its robustness and flexibility in building web applications. One of the key features that make Symfony a preferred choice among developers is its powerful routing system. The routing component of Symfony allows you to define and manage URL patterns, making it easier to handle requests and generate dynamic URLs. In this blog, we will dive deep into Symfony’s routing system, exploring its various components and showcasing code samples to demonstrate its versatility.
1. Understanding Symfony’s Routing System:
1.1 Routing in Web Applications:
Routing plays a crucial role in web development by mapping incoming HTTP requests to specific actions or resources within an application. It enables developers to define clean, human-readable URLs and handle dynamic parameters in a structured manner.
1.2 Symfony’s Routing Component:
Symfony’s routing component provides a comprehensive set of tools to handle routing in PHP web applications. It offers a flexible and powerful system for defining routes, managing parameters, and generating URLs.
2. Defining Routes:
2.1 Route Configuration:
Routes in Symfony are defined in a centralized routing configuration file, typically located in the config/routes.yaml or config/routes.php file. This configuration file maps URLs to specific controllers or actions.
Here’s an example of a basic route configuration in YAML format:
yaml # config/routes.yaml homepage: path: / controller: App\Controller\HomeController::index
In this example, the homepage route is defined with a path of /, which corresponds to the root URL of the application. The route is associated with the index method of the HomeController class.
2.2 Route Parameters:
Symfony allows you to define dynamic route parameters that can be extracted from the URL and passed to controllers or actions. Parameters are defined using curly braces {} within the route’s path.
Consider the following example:
yaml # config/routes.yaml product_show: path: /product/{id} controller: App\Controller\ProductController::show
In this case, the product_show route has a dynamic parameter {id} that can match any value within the URL. The value of the id parameter will be passed to the show method of the ProductController class.
2.3 Route Requirements:
Symfony’s routing system allows you to define requirements for route parameters using regular expressions. This ensures that the parameter values match specific patterns.
For instance:
yaml # config/routes.yaml product_show: path: /product/{id} controller: App\Controller\ProductController::show requirements: id: '\d+'
In this example, the id parameter is constrained to match only numeric values using the regular expression \d+. If a non-numeric value is provided in the URL, Symfony will return a 404 Not Found response.
3. Generating URLs:
3.1 Named Routes:
Symfony’s routing system allows you to assign names to routes, making it easier to generate URLs in a consistent and maintainable way. A named route can be referenced throughout the application to generate URLs dynamically.
Here’s an example:
yaml # config/routes.yaml product_show: path: /product/{id} controller: App\Controller\ProductController::show name: product_show_route
In this case, the product_show route is given the name product_show_route. We can now reference this name when generating URLs.
3.2 Route Generation with Parameters:
Symfony provides a convenient way to generate URLs by automatically substituting route parameters. By passing the necessary parameters, you can easily create dynamic URLs.
Consider the following example in a Twig template:
twig <a href="{{ path('product_show_route', {'id': product.id}) }}">View Product</a>
In this code snippet, the path function is used to generate the URL for the product_show_route route, substituting the id parameter with the corresponding value from the product object.
4. Advanced Routing Techniques:
4.1 Route Prefixes:
Symfony allows you to define route prefixes to group related routes together. This can be useful when you have a set of routes that share a common URL prefix.
yaml # config/routes.yaml admin_dashboard: path: /admin/dashboard controller: App\Controller\Admin\DashboardController::index admin_users: path: /admin/users controller: App\Controller\Admin\UserController::index
In this example, both the admin_dashboard and admin_users routes share the /admin prefix. This helps in organizing routes and improving readability.
4.2 Route Collections:
Symfony’s routing system supports route collections, which allow you to organize routes into logical groups. Route collections make it easier to manage routes across different areas of your application.
php // config/routes.php use Symfony\Component\Routing\RouteCollection; use Symfony\Component\Routing\Route; $routes = new RouteCollection(); $adminRoutes = new RouteCollection(); $adminRoutes->add('admin_dashboard', new Route('/admin/dashboard', ['_controller' => 'App\Controller\Admin\DashboardController::index'])); $adminRoutes->add('admin_users', new Route('/admin/users', ['_controller' => 'App\Controller\Admin\UserController::index'])); $routes->addCollection($adminRoutes); return $routes;
In this PHP example, we create a main route collection and an adminRoutes collection. The adminRoutes collection is then added to the main route collection, allowing us to group related routes together.
4.3 Route Redirection:
Symfony’s routing system provides the ability to define route redirections. This can be useful when you want to redirect specific URLs to different locations within your application.
yaml # config/routes.yaml old_about_us: path: /aboutus redirect: /about-us
In this example, the old_about_us route is defined with a redirection to the /about-us URL. When a request is made to /aboutus, Symfony will automatically redirect it to /about-us.
5. Customizing Routing Behavior:
5.1 Route Middleware:
Symfony allows you to apply middleware to routes, enabling you to modify the request and response before and after the route is executed. This allows for additional processing or validation to be performed.
php use Symfony\Component\HttpFoundation\Request; use Symfony\Component\HttpFoundation\Response; $routes->add('secured_route', new Route('/secured', [ '_controller' => 'App\Controller\SecuredController::index', ])->setMethods(Request::METHOD_GET)->before( function (Request $request) { // Perform authentication or other middleware actions } )->after( function (Request $request, Response $response) { // Modify the response or perform post-processing actions } );
In this example, middleware functions are defined using the before and after methods. These functions have access to the Request and Response objects, allowing you to customize the behavior as needed.
5.2 Route Controllers:
Symfony’s routing system allows you to map routes directly to controllers. This approach provides a clean separation of concerns and allows for better code organization.
yaml # config/routes.yaml homepage: path: / controller: App\Controller\HomeController action: index
In this example, the homepage route is mapped to the index action of the HomeController class. Symfony will automatically instantiate the controller and call the appropriate action when the route is matched.
Conclusion:
Symfony’s powerful routing system is a fundamental part of building flexible and maintainable web applications. Its features, such as route definition, parameter handling, URL generation, and advanced techniques like route prefixes and collections, provide developers with the tools needed for efficient and effective routing management. By leveraging Symfony’s routing capabilities, developers can create clean and robust applications while ensuring a seamless user experience.
Table of Contents
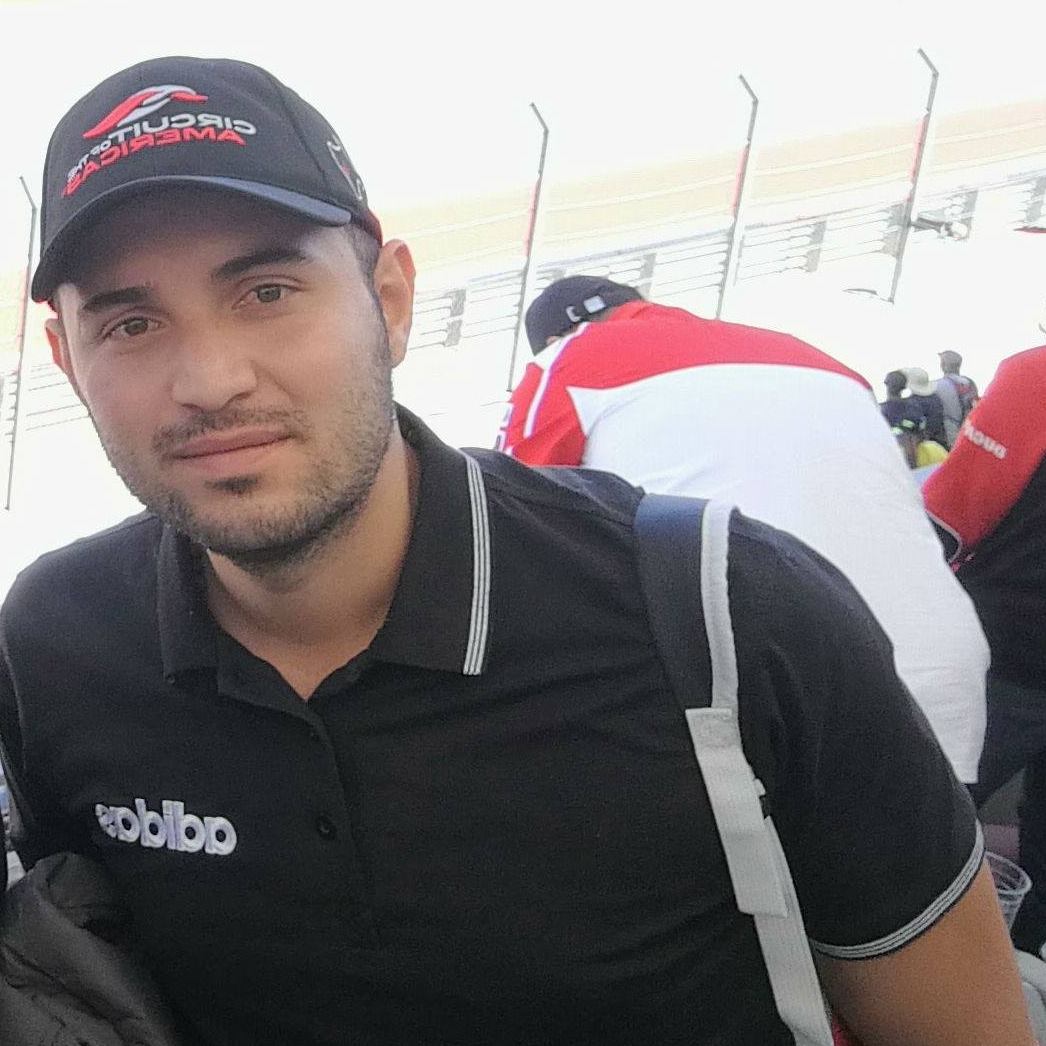
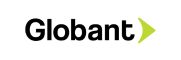