Symfony Security: Protecting Your Web App from Vulnerabilities
In today’s digital world, security is paramount. With the increasing number of cyber threats and attacks, it is crucial to ensure the safety of your web applications. Symfony, a popular PHP framework, provides a robust security component that allows you to protect your web app from vulnerabilities. In this blog post, we will explore Symfony’s security features, discuss common vulnerabilities, and provide code samples and best practices for securing your Symfony web application.
Web application security is a critical aspect of software development. An insecure application exposes sensitive user data and can lead to severe consequences, including financial losses and damage to a company’s reputation. Symfony, known for its stability and security, offers a comprehensive security component that enables developers to implement robust security measures in their web applications.
1. Understanding Symfony Security Component
Symfony’s security component provides a wide range of features for securing your web application. It offers a flexible and modular approach to authentication, authorization, and access control.
1.1 Authentication
Authentication is the process of verifying the identity of a user or system. Symfony supports various authentication methods, including form-based authentication, token-based authentication (using JSON Web Tokens), and OAuth.
php // Example of form-based authentication in Symfony public function login(AuthenticationUtils $authenticationUtils): Response { $error = $authenticationUtils->getLastAuthenticationError(); $lastUsername = $authenticationUtils->getLastUsername(); return $this->render('security/login.html.twig', [ 'last_username' => $lastUsername, 'error' => $error, ]); }
1.2 Authorization
Authorization determines whether a user has the necessary permissions to perform a specific action. Symfony’s security component provides a flexible role-based access control system. You can define roles and grant permissions to specific routes or actions based on those roles.
yaml # Example of defining access control rules in Symfony's security.yaml access_control: - { path: ^/admin, roles: ROLE_ADMIN } - { path: ^/profile, roles: ROLE_USER }
1.3 Firewall
Symfony’s firewall acts as a protective barrier around your web application. It intercepts incoming requests and applies security rules based on the configuration you define. The firewall ensures that only authenticated and authorized users can access protected resources.
yaml # Example of firewall configuration in Symfony's security.yaml firewalls: main: pattern: ^/ anonymous: true provider: app_user_provider form_login: login_path: login check_path: login default_target_path: home
2. Common Web Application Vulnerabilities
Before diving into securing your Symfony web application, it’s essential to understand the common vulnerabilities you may encounter. By being aware of these vulnerabilities, you can better protect your application against potential threats.
2.1 Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) occurs when an attacker injects malicious scripts into web pages viewed by other users. This vulnerability allows attackers to steal sensitive information, such as login credentials or session cookies. To prevent XSS attacks, Symfony provides built-in protection by escaping user-generated content and applying output encoding.
twig <!-- Example of output encoding in Twig templates --> {{ user.name|e }}
2.2 Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) involves tricking authenticated users into performing actions without their knowledge or consent. To protect against CSRF attacks, Symfony generates and validates unique tokens for each form submission. These tokens ensure that the form submission originated from your application and not from a malicious source.
twig <!-- Example of CSRF protection in Twig templates --> {{ csrf_token('comment_form') }}
2.3 SQL Injection
SQL Injection occurs when an attacker manipulates SQL queries to gain unauthorized access to your database. Symfony’s security component provides prepared statements and query builders, which help prevent SQL Injection by automatically sanitizing user input.
php // Example of using prepared statements in Symfony's Doctrine ORM $query = $entityManager->createQuery('SELECT u FROM User u WHERE u.username = :username'); $query->setParameter('username', $username); $users = $query->getResult();
2.4 Session Hijacking
Session Hijacking involves an attacker stealing or impersonating a user’s session to gain unauthorized access. Symfony provides built-in mechanisms to mitigate session hijacking, such as regenerating session IDs after successful authentication and setting secure session cookies.
yaml # Example of configuring session security in Symfony's security.yaml session: cookie_secure: auto cookie_samesite: strict cookie_lifetime: 3600
3. Securing Your Symfony Web App
Now that we have covered the common vulnerabilities, let’s explore how to secure your Symfony web application using Symfony’s security component.
3.1 Password Hashing
Storing user passwords securely is essential to prevent unauthorized access. Symfony provides password hashing algorithms, such as bcrypt and Argon2, to securely hash and store passwords in your application’s database.
php // Example of password hashing in Symfony $encodedPassword = $passwordEncoder->encodePassword($user, $plainPassword); $user->setPassword($encodedPassword);
3.2 User Authentication
Implementing user authentication is a fundamental aspect of web application security. Symfony’s security component simplifies the authentication process by providing pre-built authentication providers for common use cases.
yaml # Example of configuring a form login authentication provider in Symfony's security.yaml security: providers: app_user_provider: entity: class: App\Entity\User property: email
3.3 Role-Based Access Control
Symfony’s security component supports role-based access control, allowing you to control access to specific routes or actions based on user roles. By defining roles and associating them with users, you can restrict certain parts of your application to authorized individuals.
php // Example of restricting access to a route based on user role in Symfony's controller /** * @Route("/admin/dashboard", name="admin_dashboard") * @IsGranted("ROLE_ADMIN") */ public function adminDashboard() { // Only accessible by users with the "ROLE_ADMIN" role }
3.4 CSRF Protection
To protect against CSRF attacks, Symfony provides a built-in mechanism that automatically generates and validates CSRF tokens for form submissions. By including the generated token in your forms, you ensure that the request originated from your application.
wig <!-- Example of CSRF protection in Symfony forms --> {{ form_start(form) }} {{ form_widget(form) }} <input type="hidden" name="_token" value="{{ csrf_token('comment_form') }}"> <button type="submit">Submit</button> {{ form_end(form) }}
3.5 SQL Injection Prevention
Symfony’s security component helps prevent SQL Injection attacks by providing query builders and prepared statements. By using these features, you can ensure that user input is properly sanitized and prevent attackers from manipulating SQL queries.
php // Example of using query builders in Symfony's Doctrine ORM $queryBuilder = $entityManager->createQueryBuilder(); $queryBuilder ->select('u') ->from(User::class, 'u') ->where('u.email = :email') ->setParameter('email', $email); $users = $queryBuilder->getQuery()->getResult();
3.6 Session Security
Securing sessions is vital to prevent session hijacking. Symfony provides features like session ID regeneration after successful authentication and secure session cookies. By configuring session security options, you can enhance the protection of user sessions.
4. Best Practices for Symfony Security
In addition to implementing the security measures mentioned above, it is important to follow best practices to maintain a secure Symfony web application. Here are some best practices to consider:
- Regular Updates and Patching: Keep your Symfony installation and dependencies up to date to benefit from the latest security patches and fixes.
- Strong Password Policies: Enforce strong password policies, such as minimum length, complexity requirements, and regular password rotation.
- Principle of Least Privilege: Assign users the minimum necessary permissions to perform their tasks to reduce the potential impact of compromised accounts.
- Input Validation and Sanitization: Validate and sanitize all user input to prevent common vulnerabilities, such as SQL Injection and XSS attacks.
- Security Audits: Regularly conduct security audits and vulnerability assessments to identify potential weaknesses and address them promptly.
Conclusion
Securing your web application is of utmost importance to protect sensitive data and maintain the trust of your users. Symfony’s security component offers a comprehensive set of features to help you build secure applications. By following best practices, understanding common vulnerabilities, and implementing the provided code samples, you can ensure the security of your Symfony web application and mitigate the risk of potential attacks. Remember, security is an ongoing process, so stay vigilant and keep up to date with the latest security practices in the ever-evolving landscape of web application security.
Table of Contents
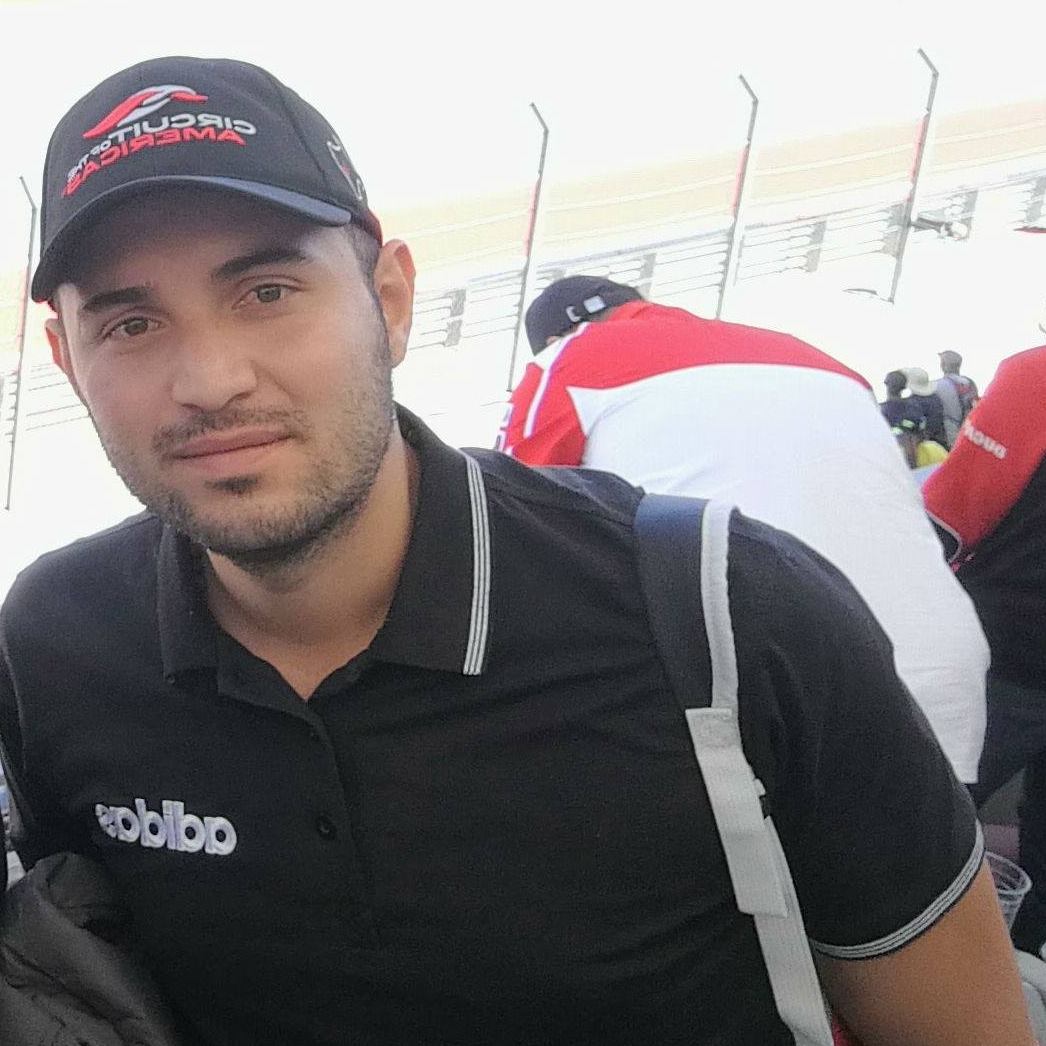
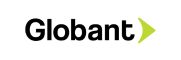