Leveraging Symfony’s Templating Engine for Dynamic Web Pages
In the world of web development, creating dynamic web pages is essential for delivering personalized and engaging user experiences. Symfony, one of the most popular PHP frameworks, provides a robust templating engine called Twig that simplifies the process of building dynamic web pages. In this blog post, we will explore the power of Symfony’s templating engine and learn how to leverage it for developing dynamic web pages. We will delve into the features of Twig and provide code samples to illustrate its usage. So let’s dive in!
1. Understanding Symfony’s Templating Engine
Symfony’s templating engine, known as Twig, is a powerful and flexible tool for generating dynamic web pages. It separates the presentation layer from the business logic, making it easier to maintain and reuse code. Twig provides a clean and intuitive syntax, which resembles HTML, making it accessible to both developers and designers.
2. Getting Started with Twig
To use Twig in your Symfony project, you first need to install it. You can do this by adding the Twig package to your project’s dependencies using Composer:
bash composer require twig/twig
Once installed, you can start using Twig in your templates. To begin, create a new Twig template file with a .twig extension. Let’s create a simple template called hello.twig:
twig <!DOCTYPE html> <html> <head> <title>Hello, Twig!</title> </head> <body> <h1>Hello, {{ name }}!</h1> </body> </html>
In the above example, we have a basic HTML structure with a dynamic greeting. The {{ name }} syntax indicates a placeholder that will be replaced with the actual value when rendering the template.
3. Rendering Twig Templates
To render a Twig template in Symfony, you need to utilize the templating service. Here’s an example of how you can render the hello.twig template we created earlier:
php use Symfony\Component\Templating\EngineInterface; class HelloController { private $templating; public function __construct(EngineInterface $templating) { $this->templating = $templating; } public function helloAction($name) { return $this->templating->render('hello.twig', ['name' => $name]); } }
In the code snippet above, we inject the templating service into the controller constructor and use its render() method to render the hello.twig template. We pass an array of variables, including the name variable, which will be substituted into the template.
4. Templating Engine Features
Twig offers a wide range of features that enable you to build powerful and dynamic web pages. Let’s explore some of the most commonly used features:
4.1. Variables and Expressions
Twig allows you to define and manipulate variables within your templates. You can assign values to variables and perform operations on them. Here’s an example:
twig {% set username = 'John' %} {% set greeting = 'Hello, ' ~ username ~ '!' %} <h1>{{ greeting }}</h1>
In the above code, we set the username variable to ‘John’ and then concatenate it with the string ‘Hello, ‘ to form the greeting variable. Finally, we display the greeting in an <h1> tag.
4.2. Control Structures
Twig provides various control structures, such as if statements and loops, which allow you to conditionally render content or iterate over data. Here’s an example:
twig {% if user.isAdmin %} <h2>Welcome, admin!</h2> {% else %} <h2>Welcome, user!</h2> {% endif %} <ul> {% for item in items %} <li>{{ item }}</li> {% endfor %} </ul>
In this code snippet, we use an if statement to conditionally display a welcome message based on whether the user is an admin. We also utilize a for loop to iterate over a list of items and display them as list items.
4.3. Filters and Functions
Twig provides a variety of built-in filters and functions that allow you to transform and manipulate data within your templates. Filters modify the output, while functions perform specific actions. Here’s an example:
twig <p>{{ message | upper }}</p> <p>{{ 'Hello, Twig!' | length }}</p>
In the above code, the upper filter is used to convert the message variable to uppercase, and the length function calculates the length of the string ‘Hello, Twig!’.
Conclusion
Symfony’s templating engine, Twig, is a powerful tool for creating dynamic web pages. It simplifies the process of rendering templates and provides a wide range of features to enhance your web development projects. In this blog post, we explored the basics of Twig, including template rendering, variables, control structures, and filters/functions. By leveraging Symfony’s templating engine, you can deliver personalized and engaging web experiences to your users. So go ahead and start harnessing the power of Twig in your Symfony applications!
Table of Contents
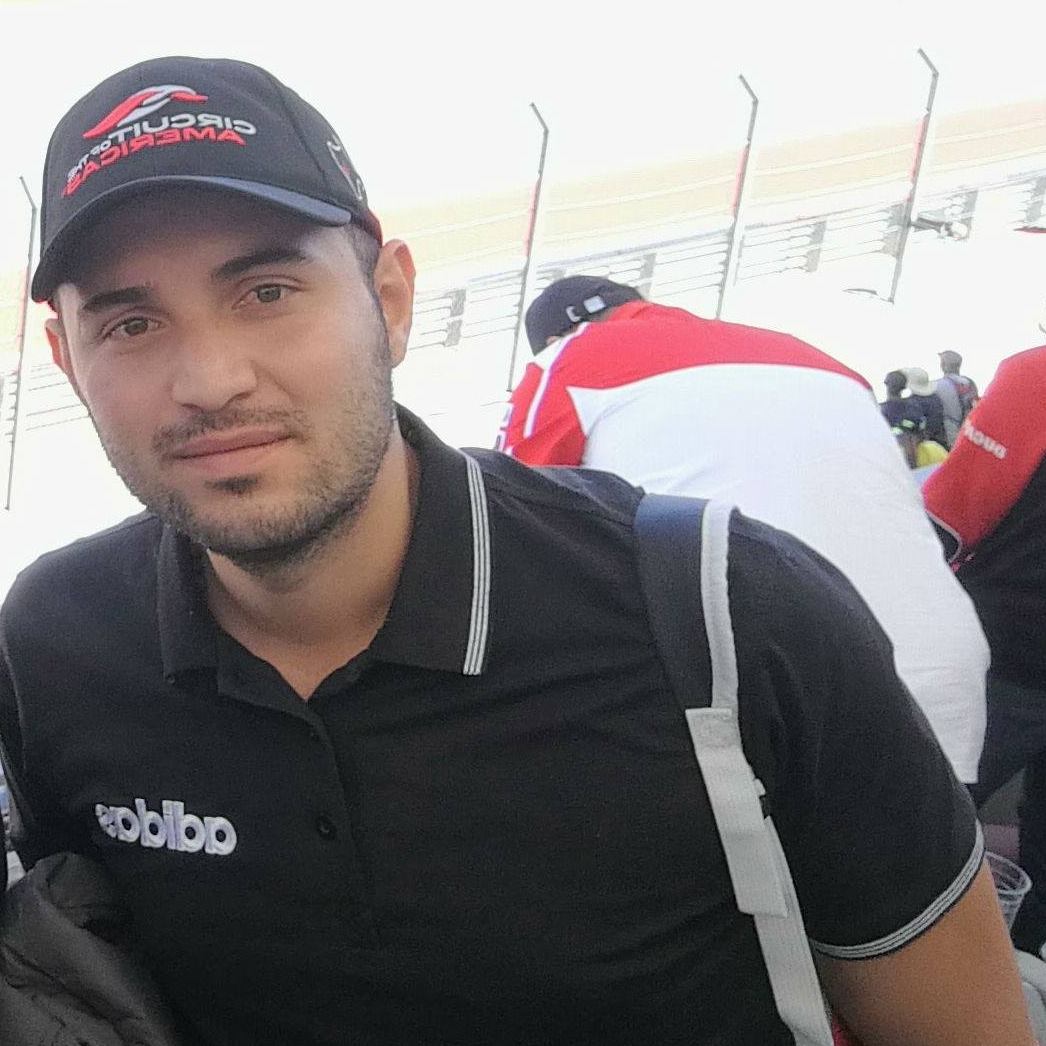
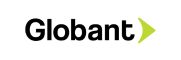