Best Practices for Testing Symfony Applications
Symfony is a popular PHP framework known for its flexibility and extensibility. Testing plays a vital role in ensuring the robustness and reliability of Symfony applications. It allows developers to catch bugs early, maintain code quality, and confidently make changes without breaking existing functionality. In this blog, we will explore the best practices for testing Symfony applications, covering various types of tests, testing tools, and techniques.
1. Unit Testing:
Unit testing is the foundation of a well-tested Symfony application. It focuses on testing individual units of code, such as functions, classes, and methods, in isolation. PHPUnit is the de facto testing framework for PHP, and Symfony provides excellent integration with it.
1.1. Setting Up PHPUnit:
To begin unit testing in Symfony, first, make sure PHPUnit is installed as a development dependency. Use Composer to add it:
bash composer require --dev phpunit/phpunit
Next, create a tests/ directory at the root of your Symfony project. This directory will house all your PHPUnit tests.
1.2. Writing PHPUnit Tests:
Let’s assume we have a Calculator class with an add method that adds two numbers. To test this method, create a new file CalculatorTest.php inside the tests/ directory:
php use PHPUnit\Framework\TestCase; class CalculatorTest extends TestCase { public function testAdd() { $calculator = new Calculator(); $result = $calculator->add(2, 3); $this->assertEquals(5, $result); } }
1.3. Test Coverage and Code Quality:
To ensure comprehensive test coverage, aim to write tests for all critical code paths. PHPUnit can generate code coverage reports that highlight areas lacking tests. Integrating tools like PHPUnit with quality analysis tools such as CodeClimate or SonarQube can further enhance code quality.
2. Functional Testing:
Functional testing focuses on testing the interaction between various components of an application. Symfony provides powerful tools for functional testing out of the box, making it easy to simulate HTTP requests and analyze responses.
2.1. Symfony’s Built-in Functional Testing Tools:
Symfony’s testing tools are based on the Symfony HttpKernel component. This allows you to create functional tests that mimic HTTP requests to your application, making it possible to test controllers, routes, and middleware.
2.2. Writing Functional Tests:
To write functional tests, create a new file CalculatorControllerTest.php inside the tests/ directory:
php use Symfony\Bundle\FrameworkBundle\Test\WebTestCase; class CalculatorControllerTest extends WebTestCase { public function testAdd() { $client = static::createClient(); $client->request('GET', '/calculator/add/2/3'); $this->assertEquals(200, $client->getResponse()->getStatusCode()); $this->assertEquals(5, $client->getResponse()->getContent()); } }
2.3. Managing Test Fixtures:
When testing interactions with a database, it’s crucial to ensure the test database is in a consistent state. Symfony provides fixtures to help manage test data and maintain a clean database state before each test. Use tools like DoctrineFixturesBundle or AliceBundle to handle fixture management.
Integration Testing:
Integration testing verifies the behavior and interaction between different parts of an application. It involves testing the integration of databases, external services, and dependencies.
3.1. Database Testing:
Symfony provides a powerful tool called Doctrine DBAL that simplifies database testing. Use a separate test database and transactions to ensure each test runs on a clean database and rolls back changes after the test.
3.2. External Service Integration:
When integrating with external services, avoid making actual API calls during testing. Instead, use libraries like Guzzle MockHandler to simulate API responses and control the behavior of external services.
3.3. Mocking Dependencies:
To isolate code units during integration testing, mock dependencies using tools like PHPUnit’s mocking capabilities or dedicated libraries like Mockery or Prophecy. Mocking allows you to control the behavior of dependencies and focus solely on testing the unit under consideration.
4. Continuous Integration and Testing:
Continuous Integration (CI) is a crucial part of modern software development. It ensures that code changes are continuously tested and integrated into the project. Symfony applications can benefit from various CI tools and services.
4.1. Automating Tests with Continuous Integration:
Use CI tools like Jenkins, Travis CI, or GitLab CI/CD to automate test execution on every code commit. Configure your CI environment to install dependencies, run tests, and generate test reports.
4.2. Test Execution and Reporting:
Ensure your CI configuration runs tests in different environments, such as different PHP versions and databases. Generate test reports in a readable format, highlighting test results, coverage, and potential issues.
4.3. Ensuring Test Environment Consistency:
Maintaining a consistent test environment is vital. Use tools like Docker or Vagrant to create reproducible test environments. Automate the setup and teardown of test databases, external services, and other dependencies to ensure consistent test results.
Conclusion:
Testing Symfony applications is essential for ensuring code quality, catching bugs early, and maintaining a robust codebase. By following the best practices outlined in this blog, you can establish a comprehensive testing strategy for your Symfony projects. Remember to prioritize unit testing, utilize functional and integration testing, and automate tests through continuous integration. With these practices in place, you can confidently build high-quality Symfony applications that meet the expectations of your users.
Table of Contents
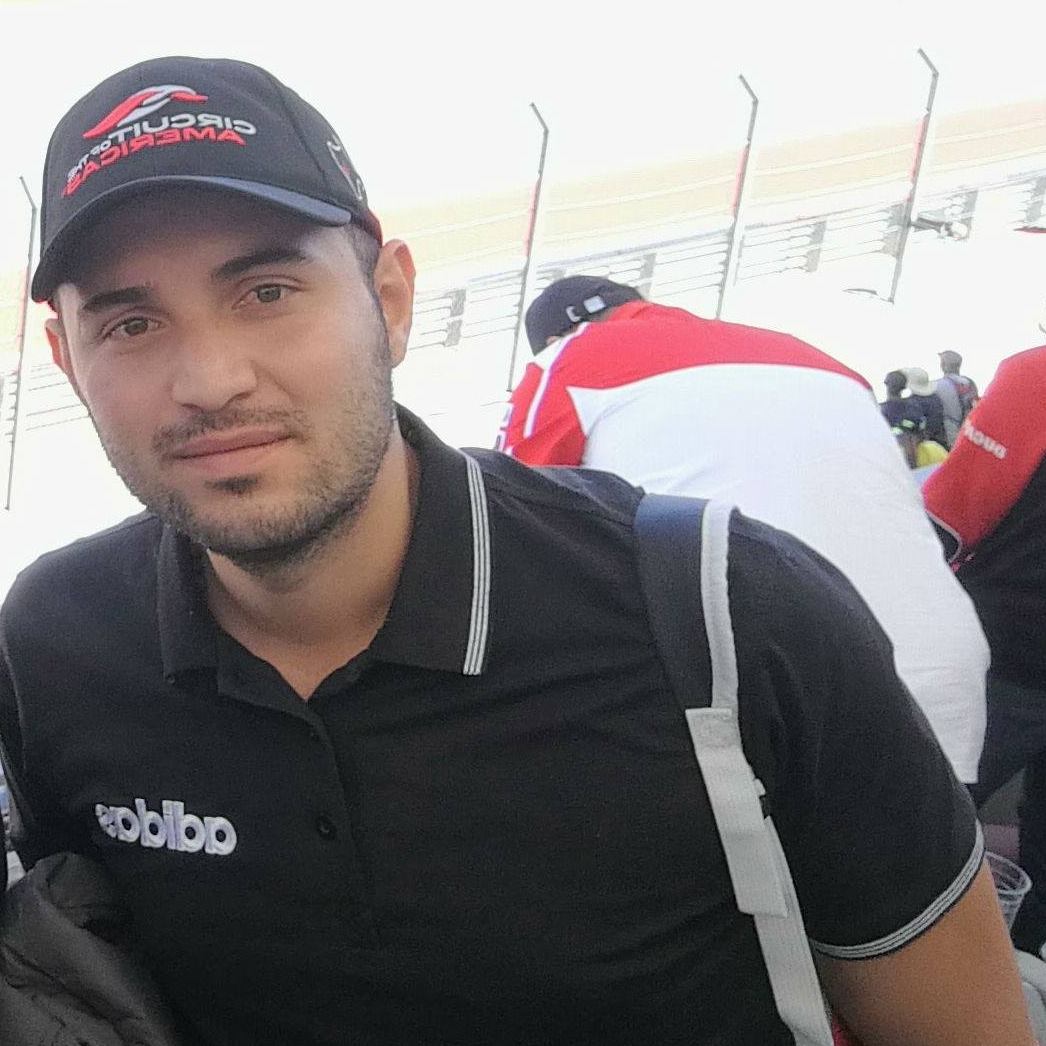
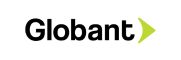