Symfony Forms: Simplifying User Input Handling
User input handling is a critical aspect of web development, and Symfony Forms is a powerful tool that simplifies this process. With its intuitive features and extensive customization options, Symfony Forms streamlines the creation and handling of user input forms, reducing development time and enhancing the overall user experience. In this blog post, we will explore the key benefits of Symfony Forms and delve into its various features to demonstrate how it can simplify user input handling.
1. Understanding Symfony Forms
Symfony Forms is a component of the Symfony PHP framework that provides a robust and flexible way to handle user input. It simplifies the creation, rendering, and processing of forms while offering advanced features such as data validation, CSRF protection, and data transformation. Symfony Forms adheres to the Model-View-Controller (MVC) architectural pattern, making it seamlessly integrate with Symfony’s ecosystem.
2. Benefits of Symfony Forms
2.1. Rapid Form Creation
Symfony Forms offers a high level of abstraction, allowing developers to create complex forms with ease. It provides a range of pre-built form field types (e.g., text, checkbox, select), making it simple to generate form elements and handle their rendering automatically. This speeds up development time and reduces the need for writing repetitive HTML and validation code manually.
2.2. Automatic Data Validation
Validating user input is crucial to ensure data integrity and security. Symfony Forms simplifies this process by providing a comprehensive validation system. It offers a wide range of validators (e.g., required, length, email) that can be applied to form fields, automatically validating user input. Validation errors are automatically associated with the corresponding fields, allowing for easy error handling and feedback to the user.
2.3. Templating and Rendering
Symfony Forms seamlessly integrates with Symfony’s templating system, enabling developers to render forms using templates. This provides flexibility in terms of form layout, styling, and customization. Developers can utilize Symfony’s powerful templating engine to create visually appealing forms that align with the application’s design and branding guidelines.
2.4. CSRF Protection
Cross-Site Request Forgery (CSRF) attacks can be detrimental to the security of an application. Symfony Forms includes built-in CSRF protection, mitigating the risk of such attacks. It generates CSRF tokens for each form, validates them on form submission, and ensures that only legitimate requests are processed. This helps prevent unauthorized access and maintains the integrity of user data.
2.5. Easy Data Transformation
Symfony Forms allows for seamless data transformation between PHP objects and form fields. It simplifies the process of mapping data from the form to the corresponding model object and vice versa. This is especially useful when dealing with complex data structures or when the form data needs to be transformed before being persisted or displayed.
3. Working with Symfony Forms
3.1. Installation and Setup
To start working with Symfony Forms, ensure that Symfony is installed on your system. If not, you can install it using Composer:
bash composer create-project symfony/skeleton my-project
Next, navigate to your project’s directory and install the Form component:
bash composer require symfony/form
3.2. Creating a Simple Form
To create a simple form, define a form class that extends Symfony’s AbstractType:
php use Symfony\Component\Form\AbstractType; use Symfony\Component\Form\FormBuilderInterface; use Symfony\Component\OptionsResolver\OptionsResolver; class ContactFormType extends AbstractType { public function buildForm(FormBuilderInterface $builder, array $options) { $builder ->add('name') ->add('email') ->add('message', TextareaType::class); } public function configureOptions(OptionsResolver $resolver) { $resolver->setDefaults([ 'data_class' => Contact::class, ]); } }
In the above example, we define a ContactFormType class that builds a form with three fields: name, email, and message. The configureOptions method specifies the data class that the form will bind to.
3.3. Handling Form Submission
Once the form is created, we need to handle its submission. In a Symfony controller, we can use the createForm method to instantiate the form and process the submission:
php public function contactForm(Request $request) { $contact = new Contact(); // Assuming the Contact class exists $form = $this->createForm(ContactFormType::class, $contact); $form->handleRequest($request); if ($form->isSubmitted() && $form->isValid()) { // Handle the form submission, e.g., save data to the database } // Render the form template with the form instance return $this->render('contact/form.html.twig', [ 'form' => $form->createView(), ]); }
In the above example, we instantiate the form using the ContactFormType class and pass in a new instance of the Contact class. The handleRequest method processes the form submission, and the isSubmitted and isValid methods validate the form data.
3.4. Form Rendering and Templating
Symfony Forms integrates seamlessly with Symfony’s templating system. To render a form, we can use the form’s view and pass it to the template:
twig {# contact/form.html.twig #} {{ form_start(form) }} {{ form_row(form.name) }} {{ form_row(form.email) }} {{ form_row(form.message) }} {{ form_end(form) }}
In the above Twig template, form_start, form_row, and form_end are used to render the form, its fields, and the form closing tag, respectively. This provides complete control over form rendering and allows for easy customization.
4. Customization and Advanced Features
4.1. Form Fields and Types
Symfony Forms provides a wide range of form field types out-of-the-box, including text fields, checkboxes, select fields, file uploads, and more. Additionally, developers can create custom form field types to handle specific requirements. These custom types can encapsulate complex functionality and provide reusable components throughout the application.
4.2. Data Transformation and Validation
Symfony Forms supports various data transformers that allow developers to transform form data before it is mapped to the model object. This is particularly useful when dealing with data that requires conversion or normalization. Furthermore, Symfony Forms’ validation system allows developers to create custom validators and apply them to form fields, ensuring data integrity and consistency.
4.3. Handling File Uploads
Uploading files is a common task in web applications. Symfony Forms simplifies file handling by providing a FileType that handles file uploads and manages file validation. Developers can easily configure the file type to define allowed file types, maximum file size, and other file-related constraints.
4.4. Form Theming
Symfony Forms’ theming system allows developers to customize the appearance of forms and their fields. By applying custom CSS classes, changing HTML structure, or overriding default form rendering blocks, developers can achieve a consistent and visually appealing form design that aligns with the application’s branding and user interface guidelines.
Conclusion
Symfony Forms is a powerful component that simplifies user input handling in web applications. By leveraging its features, developers can create complex forms rapidly, automate data validation, enhance user experience, and protect against security threats. With its seamless integration into Symfony’s ecosystem, Symfony Forms provides a flexible and customizable solution for handling user input efficiently. Whether you are building a simple contact form or a complex data entry system, Symfony Forms is an invaluable tool that streamlines the development process and ensures a robust user input handling experience.
Table of Contents
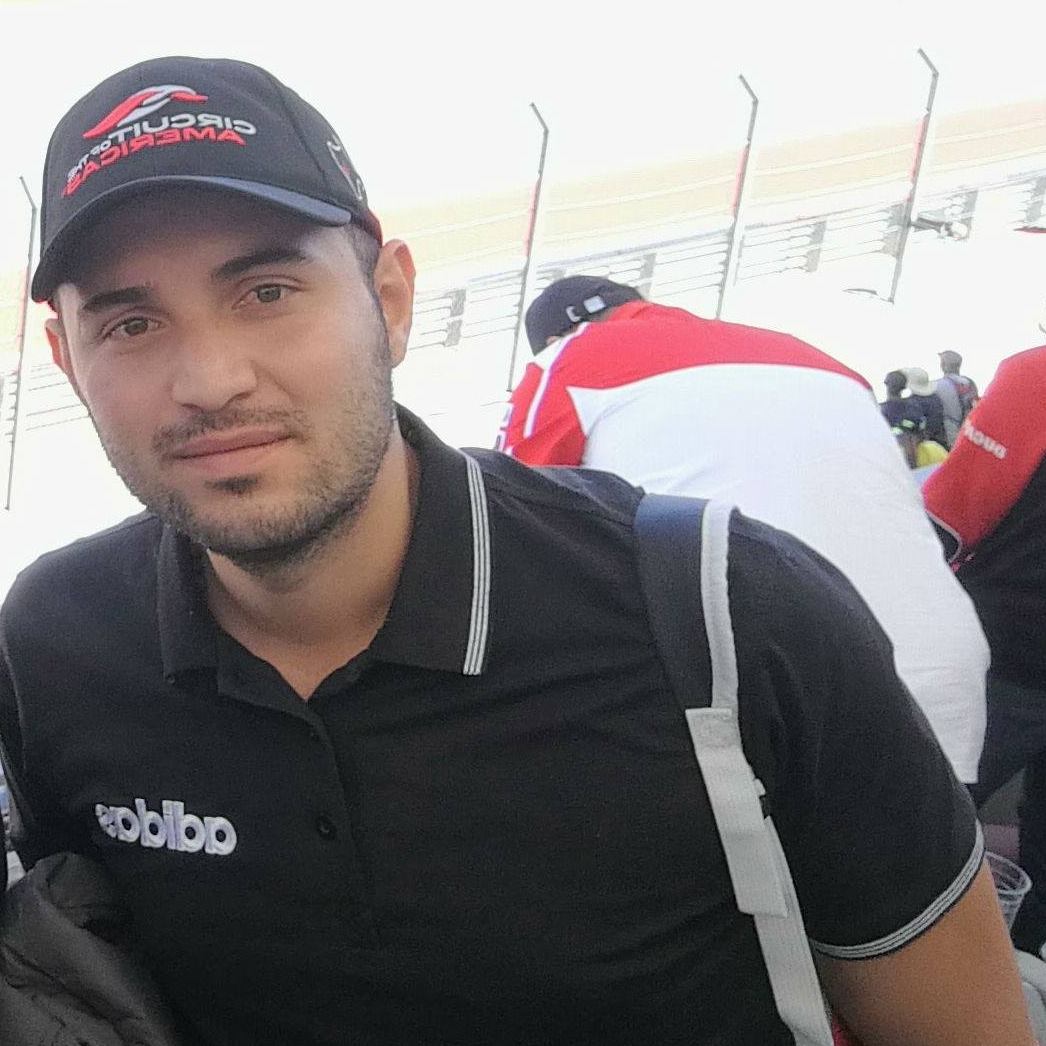
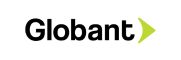