Exploring Advanced Types in TypeScript
TypeScript has become a favorite among developers due to its ability to enhance JavaScript code with strong typing and compile-time error checking. While basic types like strings, numbers, and arrays are crucial, TypeScript’s true potential shines when leveraging its advanced type system. In this blog, we’ll delve into the world of Advanced Types, understanding their applications and how they elevate the developer experience.
1. Introduction to Advanced Types
1.1 What are Advanced Types?
In TypeScript, advanced types refer to a set of powerful features that allow developers to express complex relationships between different types. These features go beyond simple primitives and enable the creation of sophisticated type definitions, providing increased type safety and code correctness.
1.2 Why use Advanced Types?
While basic types are suitable for most scenarios, they might not fully capture the intricacies of modern JavaScript applications. Advanced types offer developers the following benefits:
- Type Safety: Advanced types help catch more errors at compile-time, reducing the likelihood of runtime errors.
- Refactoring Support: Precise type definitions make refactoring easier and less error-prone.
- Better IDE Support: IDEs can provide more accurate code suggestions and autocompletions based on advanced type information.
- Enhanced Readability: Well-defined types make the codebase more understandable and self-documenting.
- Reusable Abstractions: Advanced types encourage creating reusable and composable abstractions for complex scenarios.
Let’s now explore some of the most powerful advanced types TypeScript has to offer.
2. Union Types and Intersection Types
2.1 Combining multiple types
Union types allow us to define a variable that can hold values of different types. This is achieved by using the | operator to separate the types. For example:
typescript type Status = "success" | "error" | "loading"; let currentStatus: Status; currentStatus = "success"; // Valid currentStatus = "error"; // Valid currentStatus = "done"; // Error: 'done' is not assignable to type 'Status'
Intersection types, on the other hand, allow us to combine multiple types into a single type. This is done using the & operator. An object of an intersection type will have properties from all the intersected types. For example:
typescript interface Shape { color: string; } interface Size { width: number; } type ColoredShape = Shape & Size; const coloredRectangle: ColoredShape = { color: "blue", width: 100, };
2.2 Union types in action
Consider a scenario where we want a function to accept either a string or an array of strings as an argument:
typescript function printText(text: string | string[]) { if (typeof text === "string") { console.log(text); } else { text.forEach((item) => console.log(item)); } } printText("Hello, TypeScript!"); // Output: Hello, TypeScript! printText(["Hello", "TypeScript"]); // Output: Hello TypeScript // …
Union types provide a concise way to handle different argument types without resorting to complex type checks.
2.3 Intersection types in action
In a UI library, we might need to merge the props of different components. With intersection types, we can easily achieve this:
typescript interface ButtonProps { text: string; onClick: () => void; } interface IconProps { icon: string; onClick: () => void; } type IconButtonProps = ButtonProps & IconProps; const shareButton: IconButtonProps = { text: "Share", icon: "share-icon", onClick: () => { // Share functionality }, };
Here, IconButtonProps combines the properties of both ButtonProps and IconProps, allowing us to create buttons with icons.
3. Conditional Types
3.1 Introduction to conditional types
Conditional types in TypeScript enable us to create type definitions that depend on a condition. The syntax for a conditional type is as follows:
typescript type ConditionalType<T> = T extends U ? X : Y;
The type ConditionalType checks if type T is assignable to type U. If true, it evaluates to type X, otherwise to type Y.
3.2 Using conditional types with generics
Conditional types are commonly used with generics to provide more precise typing based on specific conditions. For instance, let’s define a utility type that extracts the property names of an object whose values are of type string:
typescript type StringPropertyNames<T> = { [K in keyof T]: T[K] extends string ? K : never; }[keyof T]; interface Person { name: string; age: number; email: string; } type StringPropsOfPerson = StringPropertyNames<Person>; // "name" | "email"
In this example, StringPropertyNames iterates over the keys of T and checks if each value is of type string. If true, the key is included in the resulting type; otherwise, it is mapped to never and omitted from the final type.
3.3 Practical use case: Dynamic mapping of object properties
Conditional types are valuable when dealing with data transformation. Suppose we have an API response with inconsistent property names and want to convert it to a more structured format:
typescript interface ApiResponse { usr_n: string; usr_yrs: number; usr_em: string; } type CamelCaseKeys<T> = { [K in keyof T as Uncapitalize<string & K>]: T[K]; }; const apiResponse: ApiResponse = { usr_n: "John Doe", usr_yrs: 30, usr_em: "john@example.com", }; const formattedData = apiResponse as CamelCaseKeys<ApiResponse>; /* formattedData is now: { usr_n: "John Doe", usr_yrs: 30, usr_em: "john@example.com", } */
In this example, the CamelCaseKeys conditional type maps each key of ApiResponse to its camel-cased version, resulting in a more consistent and readable format.
4. Mapped Types
4.1 Simplify object transformations
Mapped types allow us to create new types based on the properties of an existing type. The general syntax for mapped types is as follows:
typescript type MappedType<T> = { [P in keyof T]: /* new type definition based on T[P] */; };
This iterates over each property P in T and defines a new type based on T[P].
4.2 Read-only and optional properties
Common use cases for mapped types include creating read-only or optional versions of an interface. Let’s see some examples:
typescript interface Product { name: string; price: number; } type ReadonlyProduct = { readonly [P in keyof Product]: Product[P]; }; type OptionalProduct = { [P in keyof Product]?: Product[P]; }; const product: ReadonlyProduct = { name: "Laptop", price: 999, }; product.name = "Desktop"; // Error: Cannot assign to 'name' because it is a read-only property const partialProduct: OptionalProduct = { name: "Tablet", }; // Partially constructed product: { name: "Tablet" }
4.3 Mapping over tuples and arrays
Mapped types also work with tuples and arrays. For example, we can create a utility type that transforms each element of a tuple to a nullable version:
typescript type Nullable<T> = { [K in keyof T]: T[K] | null; }; type StringNumberBoolean = [string, number, boolean]; type NullableStringNumberBoolean = Nullable<StringNumberBoolean>; // NullableStringNumberBoolean: [string | null, number | null, boolean | null]
In this case, the Nullable mapped type makes each element of the tuple nullable.
5. Type Guards and User-Defined Type Guards
5.1 Narrowing down types in conditionals
TypeScript’s type system relies on the concept of “type guards” to narrow down the type of a variable based on runtime checks. Type guards are conditions that return a boolean and are used in if statements or ternary expressions.
For instance, consider a function that takes an argument of type string | number and performs a different action based on the type:
typescript function processInput(input: string | number) { if (typeof input === "string") { // Input is of type 'string' console.log(input.toUpperCase()); } else { // Input is of type 'number' console.log(input.toFixed(2)); } } processInput("hello"); // Output: "HELLO" processInput(3.14159); // Output: "3.14"
The typeof type guard checks whether input is a string, allowing us to safely use string-specific methods without triggering a type error.
5.2 Implementing custom type guards
TypeScript also allows us to define custom type guards using user-defined type predicates. A user-defined type guard is a function that returns a type predicate. A type predicate is a special type that informs the TypeScript compiler about the narrowed-down type inside an if statement.
For example, let’s create a custom type guard to check if an object has a property called “length”:
typescript function hasLengthProperty(obj: any): obj is { length: number } { return typeof obj.length === "number"; } function printLength(obj: any) { if (hasLengthProperty(obj)) { console.log(`Length: ${obj.length}`); } else { console.log("Object does not have a length property."); } } const arr = [1, 2, 3]; const str = "Hello"; printLength(arr); // Output: "Length: 3" printLength(str); // Output: "Length: 5"
In this example, the hasLengthProperty function acts as a type guard and narrows down the type of obj to { length: number } inside the if statement. This allows us to safely access the length property without causing type errors.
5.3 Advanced type guarding techniques
TypeScript supports a wide range of type guards, including:
- typeof type guards, as shown in previous examples.
- instanceof type guards to check if an object is an instance of a specific class.
- Literal type guards to check for specific literal values (e.g., typeof input === “string”).
- in type guards to check if a property exists on an object.
- Custom type guards using user-defined type predicates, as demonstrated earlier.
By using these type guarding techniques, you can ensure more precise and safe type checking in your TypeScript code.
6. Exhaustive Type Checking
6.1 Leveraging discriminated unions
Discriminated unions are a powerful pattern in TypeScript that allows you to model a type that can have different variants, each identified by a shared property called a “discriminant.” The discriminant property is usually a literal type, such as a string or a number.
Consider the following example, where we have a type representing different shapes:
typescript interface Circle { kind: "circle"; radius: number; } interface Square { kind: "square"; sideLength: number; } type Shape = Circle | Square;
Here, the kind property acts as the discriminant for the Shape union type.
6.2 Achieving exhaustive type checking
With discriminated unions, TypeScript can perform exhaustive type checking in switch statements. This means that if you cover all the possible variants of the union type in the switch statement, TypeScript will ensure that you handle all cases, eliminating the possibility of having unhandled cases.
typescript function area(shape: Shape): number { switch (shape.kind) { case "circle": return Math.PI * shape.radius ** 2; case "square": return shape.sideLength ** 2; default: // TypeScript will warn if there are missing cases. throw new Error("Unhandled shape."); } }
By handling all possible cases, TypeScript guarantees that any new variants added to the Shape union in the future will be flagged as errors until properly addressed, ensuring better code robustness.
7. Recursive Types
7.1 Defining self-referential types
Recursive types in TypeScript are types that reference themselves within their definition. These types are particularly useful when working with data structures that contain nested or hierarchical elements.
For instance, let’s define a simple binary tree using a recursive type:
typescript type TreeNode<T> = { value: T; left?: TreeNode<T>; right?: TreeNode<T>; }; const tree: TreeNode<number> = { value: 10, left: { value: 5, left: { value: 2 }, right: { value: 7 }, }, right: { value: 15, left: { value: 12 }, right: { value: 20 }, }, };
Here, the TreeNode type is self-referential, as both the left and right properties can hold another TreeNode<T>.
7.2 Recursive type limitations
While recursive types can be very helpful in certain situations, it’s essential to be cautious about infinite type expansions. In TypeScript, recursive types must be well-founded, meaning they must have a base case where the recursion stops.
For example, the following type definition would lead to an infinite expansion:
typescript type InfiniteType = { next: InfiniteType; }; // This will cause a TypeScript error due to infinite type expansion.
To prevent infinite expansions, recursive types must have a well-defined stopping condition, like the optional left and right properties in our binary tree example.
7.3 Recursive types in data structures
Recursive types are especially valuable when dealing with nested data structures like linked lists, trees, or graphs. By leveraging self-referential types, you can model complex data hierarchies while ensuring type safety and readability.
8. Utility Types
8.1 Built-in utility types
TypeScript comes with a set of built-in utility types that simplify common type transformations and manipulations. These utility types are generic and operate on existing types to create new types.
Some commonly used utility types include:
- Partial<T>: Makes all properties of T optional.
- Required<T>: Makes all properties of T required.
- Readonly<T>: Makes all properties of T read-only.
- Pick<T, K>: Creates a type with only the selected properties K from T.
- Omit<T, K>: Creates a type without the selected properties K from T.
- Record<K, T>: Creates an object type with properties of type T indexed by K.
- Exclude<T, U>: Creates a type by excluding all elements of U from T.
For example:
typescript interface User { id: number; name: string; age: number; } type PartialUser = Partial<User>; type UserWithoutID = Omit<User, "id">;
8.2 Custom utility types
In addition to built-in utility types, you can create your custom utility types using mapped types, conditional types, and type inference.
For instance, let’s create a custom utility type to remove all null and undefined properties from an object:
typescript type NonNullableProps<T> = { [K in keyof T]: NonNullable<T[K]>; }; interface Example { a: string | null; b: number | undefined; c: boolean; } type CleanExample = NonNullableProps<Example>; // CleanExample: { a: string; b: number; c: boolean }
Here, NonNullableProps uses the NonNullable built-in utility type to remove null and undefined from each property of the object.
8.3 Composing utility types for powerful abstractions
By combining built-in and custom utility types, you can create powerful abstractions that simplify complex type transformations and ensure type safety throughout your codebase. These utility types play a significant role in making TypeScript code more concise and maintainable.
9. Conditional Module Loading with Type Predicates
9.1 Introduction to type predicates
TypeScript’s type system is not limited to just static analysis but also offers runtime capabilities through type predicates. A type predicate is a function that returns a type predicate annotation to inform the compiler about the narrowed-down type within a specific code block.
Type predicates can be utilized in conditional module loading to load modules based on specific conditions. This technique can be particularly useful in scenarios where you want to reduce the size of your bundled JavaScript by dynamically importing modules only when needed.
9.2 Conditional loading of modules
Consider a scenario where you have an application with multiple themes, and you want to load the appropriate theme module based on the user’s preference.
First, you can create a base theme interface and a couple of theme implementations:
typescript interface Theme { name: string; apply(): void; } const darkTheme: Theme = { name: "Dark", apply() { // Apply dark theme styles }, }; const lightTheme: Theme = { name: "Light", apply() { // Apply light theme styles }, };
Next, create a type predicate function to check if a given theme is the dark theme:
typescript function isDarkTheme(theme: Theme): theme is typeof darkTheme { return theme.name === darkTheme.name; }
Now, you can conditionally load the theme module based on the user’s preference:
typescript let userPreference: Theme = darkTheme; // User preference from settings or user agent if (isDarkTheme(userPreference)) { import("./darkThemeModule").then((module) => { module.applyTheme(userPreference); }); } else { import("./lightThemeModule").then((module) => { module.applyTheme(userPreference); }); }
In this example, if the user’s preference is the dark theme, the “./darkThemeModule” is dynamically imported and applied; otherwise, the “./lightThemeModule” is imported and applied.
9.3 Improving performance and reducing bundle size
By conditionally loading modules based on type predicates, you can significantly reduce the initial bundle size of your application. Themes that are not in use won’t be included in the initial bundle, leading to faster load times and improved performance.
Additionally, type predicates provide type safety during the conditional module loading process, ensuring that the correct modules are imported and applied based on the narrowed-down type.
Conclusion
In this blog post, we’ve explored the world of Advanced Types in TypeScript. From union and intersection types to conditional types and user-defined type guards, we’ve seen how these powerful features enhance type safety, code readability, and maintainability.
We’ve also delved into mapped types, recursive types, utility types, and conditional module loading with type predicates, showcasing how they help us build robust and flexible applications.
As TypeScript continues to evolve, it’s essential to stay updated with the latest advancements in the language to make the most of its capabilities and build better JavaScript applications with confidence.
Happy typing in TypeScript!
Table of Contents
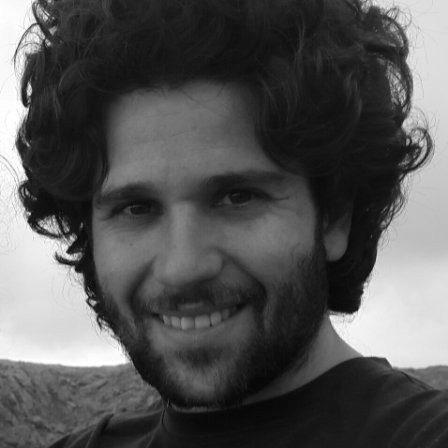
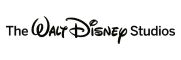