TypeScript and Design Patterns: Building Better Software
In the world of software development, the quest for building efficient, maintainable, and scalable applications is ongoing. One of the ways to achieve this goal is by utilizing TypeScript and design patterns in tandem. TypeScript, a superset of JavaScript, adds static typing and advanced features to the language. Design patterns, on the other hand, are proven solutions to recurring software design problems. Combining these two elements can lead to cleaner code, improved architecture, and streamlined development. In this article, we’ll delve into the synergy between TypeScript and design patterns, exploring their benefits and providing illustrative examples.
Table of Contents
1. Understanding TypeScript: A Brief Overview
TypeScript has rapidly gained popularity in the web development community due to its ability to address many of JavaScript’s inherent issues. By introducing static typing, TypeScript allows developers to catch errors early in the development process, reducing runtime bugs and enhancing code quality. This typing also facilitates better tooling, as IDEs can provide more accurate suggestions and autocomplete options.
TypeScript extends JavaScript’s capabilities by offering features like classes, interfaces, and modules. This makes it more suitable for building large-scale applications with well-organized codebases. Additionally, TypeScript can be compiled into plain JavaScript, making it compatible with virtually any browser and runtime.
2. Exploring Design Patterns
Design patterns are reusable solutions to common problems encountered during software design. They provide a structured approach to solving challenges, promoting code reusability, maintainability, and scalability. Integrating design patterns in your development process can lead to a more organized codebase and a better software architecture.
Let’s explore a few essential design patterns and see how they can be effectively implemented using TypeScript.
2.1. Singleton Pattern
The Singleton Pattern ensures that a class has only one instance and provides a global point of access to that instance. This is particularly useful when you want to limit the number of instances of a class and maintain a single point of control.
Here’s an example of implementing the Singleton Pattern in TypeScript:
typescript class Singleton { private static instance: Singleton; private constructor() { // Private constructor to prevent instantiation } static getInstance(): Singleton { if (!Singleton.instance) { Singleton.instance = new Singleton(); } return Singleton.instance; } } const instance1 = Singleton.getInstance(); const instance2 = Singleton.getInstance(); console.log(instance1 === instance2); // Output: true
2.2. Factory Pattern
The Factory Pattern provides an interface for creating objects in a super class, but allows subclasses to alter the type of objects that will be created. This promotes loose coupling and enhances flexibility in object creation.
Consider the following TypeScript implementation of the Factory Pattern:
typescript interface Product { operation(): string; } class ConcreteProduct1 implements Product { operation(): string { return 'Product 1'; } } class ConcreteProduct2 implements Product { operation(): string { return 'Product 2'; } } class Creator { createProduct(): Product { throw new Error('Method not implemented.'); } } class ConcreteCreator1 extends Creator { createProduct(): Product { return new ConcreteProduct1(); } } class ConcreteCreator2 extends Creator { createProduct(): Product { return new ConcreteProduct2(); } } const creator1 = new ConcreteCreator1(); const product1 = creator1.createProduct(); const creator2 = new ConcreteCreator2(); const product2 = creator2.createProduct(); console.log(product1.operation()); // Output: Product 1 console.log(product2.operation()); // Output: Product 2
2.3. Observer Pattern
The Observer Pattern defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically. This is especially useful in scenarios where multiple parts of an application need to stay in sync.
Let’s implement the Observer Pattern using TypeScript, focusing on a basic example in a React context:
typescript interface Observer { update(message: string): void; } class ConcreteObserver implements Observer { update(message: string): void { console.log(`Received message: ${message}`); } } class Subject { private observers: Observer[] = []; attach(observer: Observer): void { this.observers.push(observer); } notify(message: string): void { for (const observer of this.observers) { observer.update(message); } } } const subject = new Subject(); const observer1 = new ConcreteObserver(); const observer2 = new ConcreteObserver(); subject.attach(observer1); subject.attach(observer2); subject.notify('Hello, observers!');
3. TypeScript and Design Patterns in Action
Now, let’s put our knowledge to the test by applying TypeScript and design patterns to practical examples.
3.1. Implementing the Singleton Pattern in TypeScript
Consider a scenario where you need to manage a configuration object throughout your application’s lifecycle. The Singleton Pattern can be an ideal choice for this:
typescript class ConfigurationManager { private static instance: ConfigurationManager; private config: any = {}; private constructor() { // Load configuration from a file or other source this.config = { apiKey: 'your-api-key', apiUrl: 'https://api.example.com' }; } static getInstance(): ConfigurationManager { if (!ConfigurationManager.instance) { ConfigurationManager.instance = new ConfigurationManager(); } return ConfigurationManager.instance; } getConfig(key: string): any { return this.config[key]; } } const configManager = ConfigurationManager.getInstance(); console.log(configManager.getConfig('apiKey')); // Output: your-api-key
3.2. Utilizing the Factory Pattern for Object Creation
Imagine you’re building a game with various types of characters. The Factory Pattern can help you create these characters dynamically:
typescript interface Character { attack(): string; } class Warrior implements Character { attack(): string { return 'Warrior attacks with a sword.'; } } class Mage implements Character { attack(): string { return 'Mage casts a fireball spell.'; } } class CharacterFactory { createCharacter(type: string): Character { switch (type) { case 'warrior': return new Warrior(); case 'mage': return new Mage(); default: throw new Error(`Character type ${type} not supported.`); } } } const characterFactory = new CharacterFactory(); const warrior = characterFactory.createCharacter('warrior'); console.log(warrior.attack()); // Output: Warrior attacks with a sword. const mage = characterFactory.createCharacter('mage'); console.log(mage.attack()); // Output: Mage casts a fireball spell.
3.3. Applying the Observer Pattern to React State Changes
In a React application, the Observer Pattern can be useful for handling state changes and ensuring that different components stay in sync:
typescript import React, { useState, useEffect } from 'react'; interface Observer { update(newState: any): void; } function useSubject(initialState: any) { const [state, setState] = useState(initialState); const observers: Observer[] = []; const attach = (observer: Observer) => { observers.push(observer); }; const notify = (newState: any) => { for (const observer of observers) { observer.update(newState); } }; const updateState = (newState: any) => { setState(newState); notify(newState); }; return { state, updateState, attach }; } function ComponentA() { const { state, attach } = useSubject(''); useEffect(() => { const observer: Observer = { update(newState: any) { console.log(`Component A received new state: ${newState}`); } }; attach(observer); }, [attach]); return <div>Component A: {state}</div>; } function ComponentB() { const { state, updateState } = useSubject(''); useEffect(() => { const interval = setInterval(() => { updateState(new Date().toLocaleTimeString()); }, 1000); return () => clearInterval(interval); }, [updateState]); return <div>Component B: {state}</div>; } function App() { return ( <div> <ComponentA /> <ComponentB /> </div> ); } export default App;
4. Benefits of Using TypeScript and Design Patterns Together
The marriage of TypeScript and design patterns brings forth a multitude of advantages for software development:
- Code Clarity and Maintainability: TypeScript’s static typing and design patterns encourage well-structured code, making it easier to understand and maintain.
- Bug Prevention: Static typing in TypeScript helps catch type-related errors before runtime, reducing the occurrence of bugs.
- Scalability: Design patterns provide blueprints for solving problems, making it easier to scale and extend applications.
- Reusability: Design patterns promote the reuse of proven solutions, leading to more efficient development.
- Collaboration: The combination of TypeScript’s type annotations and clear design patterns facilitates collaboration among team members.
- Adaptability: Design patterns allow for flexible modifications and enhancements to software without affecting the entire codebase.
Conclusion
TypeScript and design patterns are powerful tools that, when used in conjunction, can greatly improve the quality and maintainability of your software. TypeScript’s static typing enhances code robustness, while design patterns offer elegant solutions to common problems. By adopting these practices, you can build software that is not only efficient and scalable but also easier to understand and maintain over time. As you continue your journey in software development, consider harnessing the synergy between TypeScript and design patterns to build better software for the future.
Table of Contents
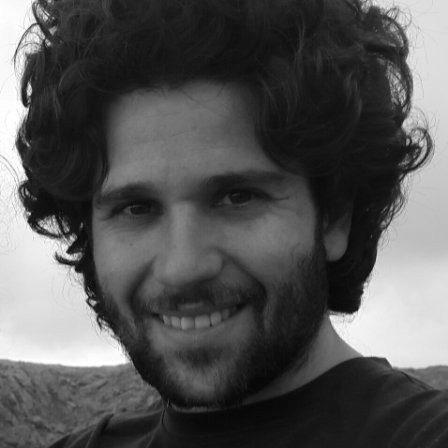
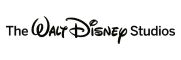