Developing Voice Applications with TypeScript and Alexa Skills
Voice technology has rapidly become an integral part of our daily lives, with virtual assistants like Amazon Alexa leading the way. These virtual assistants are more than just voice-activated speakers; they represent a new frontier of user interaction and engagement. If you’re a developer looking to tap into the potential of voice applications, this guide will walk you through the process of building Alexa Skills using TypeScript. We’ll cover everything from setting up your development environment to creating captivating voice experiences that will delight your users.
Table of Contents
1. Introduction
1.1. The Rise of Voice Technology
Voice technology has evolved beyond being a mere novelty. With devices like Amazon Echo and Alexa becoming household names, voice assistants are reshaping the way we interact with technology. From controlling smart home devices to providing weather updates and playing music, voice-enabled devices have become integral to our daily routines.
1.2. Why TypeScript for Voice Applications?
TypeScript, a superset of JavaScript, has gained popularity for its ability to add static typing to JavaScript development. This feature helps catch errors early, leading to more reliable and maintainable code. When developing voice applications, especially for platforms like Alexa, the combination of TypeScript’s strong typing and enhanced tooling makes it an ideal choice for a smooth development experience.
2. Getting Started
2.1. Setting Up Your Development Environment
Before diving into voice application development, you need to set up your development environment. Make sure you have Node.js and npm (Node Package Manager) installed. You’ll also need a code editor; Visual Studio Code is a popular choice due to its TypeScript support and rich extensions for Alexa Skills development.
2.2. Creating an Amazon Developer Account
To develop and publish Alexa Skills, you’ll need an Amazon Developer Account. Go to the Amazon Developer Console, sign in with your Amazon account, and create a new developer account if you don’t have one. This account will be used to manage your Alexa Skills and interact with Amazon’s developer tools.
3. Building Your First Alexa Skill
3.1. Designing the User Interaction
Before writing a single line of code, it’s essential to design your skill’s user interaction. Decide on the skill’s purpose, the intents it will handle, and the overall flow of the conversation. Amazon provides tools like the Alexa Skill Design Guide to help you design compelling voice user interfaces (VUI).
3.2. Creating the Skill Using TypeScript
Let’s start by creating a simple “Hello World” Alexa Skill using TypeScript. Begin by initializing a new Node.js project:
bash mkdir MyAlexaSkill cd MyAlexaSkill npm init
Install the necessary packages:
bash npm install ask-sdk-core axios npm install --save-dev @types/node @types/axios
Now, let’s create a TypeScript file named index.ts:
typescript import { HandlerInput, SkillBuilders } from 'ask-sdk-core'; import axios from 'axios'; const LaunchRequestHandler = { canHandle(handlerInput: HandlerInput) { return handlerInput.requestEnvelope.request.type === 'LaunchRequest'; }, handle(handlerInput: HandlerInput) { const speechText = 'Welcome to the Hello World skill!'; return handlerInput.responseBuilder .speak(speechText) .getResponse(); }, }; const HelloWorldIntentHandler = { canHandle(handlerInput: HandlerInput) { return handlerInput.requestEnvelope.request.type === 'IntentRequest' && handlerInput.requestEnvelope.request.intent.name === 'HelloWorldIntent'; }, async handle(handlerInput: HandlerInput) { const response = await axios.get('https://api.example.com/greeting'); const greeting = response.data.greeting; return handlerInput.responseBuilder .speak(`The server says: ${greeting}`) .getResponse(); }, }; export const handler = SkillBuilders.custom() .addRequestHandlers( LaunchRequestHandler, HelloWorldIntentHandler ) .lambda();
This example demonstrates a simple skill with a Launch Request handler and an Intent handler. The Intent handler sends a request to an external API and responds with the received greeting.
4. Voice Interaction Design
4.1. Designing Voice User Interfaces (VUI)
Voice interaction design requires a different approach compared to traditional graphical interfaces. You need to consider the natural flow of a conversation, the variety of ways users might express the same intent, and how to provide clear and concise responses. Use Amazon’s VUI Design Guide to create an intuitive and engaging experience.
4.2. Handling User Intents and Slots
Intents represent the actions a user wants to perform, while slots capture specific pieces of information within those intents. For instance, in a weather app, a “GetWeatherIntent” might have a “City” slot. Define your intents and slots in your skill’s interaction model, often represented using JSON. With TypeScript, you can create models for these intents and slots to enhance code readability and reliability.
5. TypeScript and Alexa Skill Development
5.1. Leveraging TypeScript for Reliable Development
TypeScript brings static typing to your Alexa Skill development, catching errors before runtime and improving code quality. You can define interfaces for request and response objects, ensuring proper structure and reducing the likelihood of bugs.
5.2. Defining Skill Models and Handlers
Create separate TypeScript files for your skill’s models and handlers. Define interfaces for request and response objects, keeping your codebase organized and maintainable. Utilize TypeScript’s inheritance and composition features to build modular and extensible handlers for different intents.
6. Enhancing User Experience
6.1. Adding Speech Output and Text-to-Speech
Incorporate speech output to interact with users effectively. You can use text-to-speech (TTS) to convert text responses into natural-sounding speech. Amazon provides built-in SSML (Speech Synthesis Markup Language) support, allowing you to control aspects like pitch, rate, and emphasis for a lifelike conversation.
6.2. Utilizing Cards for Visual Responses
While voice is the primary mode of interaction, you can also enhance user experience by using visual responses called cards. Cards display additional information on devices with screens, complementing the voice interaction. TypeScript enables you to generate card content dynamically based on the skill’s responses.
7. Testing and Debugging
7.1. Testing Your Skill Using Simulators
Amazon provides simulators that allow you to test your skill without deploying it to a physical device. The Alexa Simulator and Echo Show Simulator help you verify the skill’s responses, speech output, and card content.
7.2. Debugging Voice Applications
Debugging voice applications might seem challenging due to the lack of traditional visual feedback. However, you can log interactions, intents, and responses to the console during testing. Leverage TypeScript’s strong typing to catch potential errors early and ensure a smooth debugging process.
8. Deployment and Certification
8.1. Preparing Your Skill for Deployment
Before deploying your skill, thoroughly test it on simulators and real devices. Ensure that the skill’s interaction is intuitive, and responses are accurate and engaging. Double-check any external API integrations for reliability.
8.2. Submitting for Amazon Certification
To make your skill available to users, you need to submit it for certification by Amazon. Ensure your skill adheres to Amazon’s certification guidelines and passes the necessary tests. Once certified, your skill can be published and accessed by millions of Alexa users.
9. Advanced Concepts
9.1. Multi-Modal Voice Experiences
Alexa-enabled devices with screens support multi-modal interactions, combining both voice and visual elements. Take advantage of TypeScript’s flexibility to create immersive experiences that seamlessly transition between voice and visual components.
9.2. Integrating APIs and External Services
Extend the functionality of your Alexa Skill by integrating with external APIs and services. Whether it’s fetching real-time data or connecting to third-party platforms, TypeScript’s robustness ensures a reliable integration process.
10. Best Practices
10.1. Writing Maintainable Voice Code
Adhere to software engineering best practices when developing Alexa Skills. Keep your code modular, with well-defined interfaces and separation of concerns. Leverage TypeScript’s features like interfaces and enums to enhance code readability and maintainability.
10.2. Ensuring Accessibility and Inclusivity
Consider accessibility and inclusivity when designing your voice application. Provide alternatives for users with different abilities and ensure that your skill is easy to use for everyone.
Conclusion
Developing voice applications using TypeScript and Alexa Skills opens up exciting possibilities for engaging user interactions. With the power of TypeScript’s static typing and Amazon’s extensive developer tools, you can create voice experiences that captivate and delight users. Whether you’re building simple utilities or complex multi-modal experiences, TypeScript empowers you to craft reliable, maintainable, and accessible voice applications that shape the future of technology. Start your journey into the world of voice application development and create experiences that leave a lasting impact on users around the globe.
Table of Contents
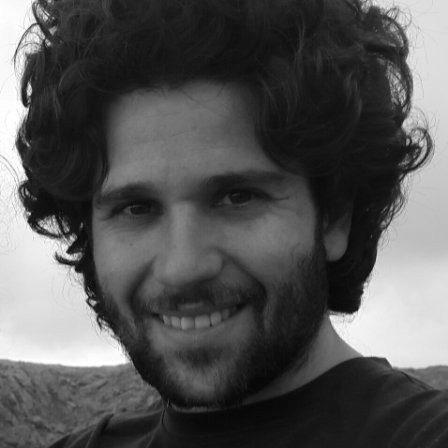
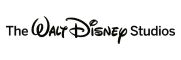