Interfaces in TypeScript: Defining Contracts
As software developers, we often find ourselves working on projects that involve complex data structures and interactions between various components. Maintaining a clear understanding of how these components communicate with each other is crucial for building robust, scalable, and maintainable applications. This is where TypeScript interfaces come to the rescue!
In this blog post, we’ll dive deep into the world of TypeScript interfaces and explore how they help us define contracts between different parts of our code. We’ll cover the basics, advanced usage, and practical examples to demonstrate the power of interfaces in ensuring type safety and improving the overall development experience.
1. Introduction to TypeScript Interfaces
1.1 What are Interfaces?
In TypeScript, an interface is a way to define a contract or a shape that other parts of the code must adhere to. It acts as a blueprint for objects, describing their structure and types of properties or methods they should have. By using interfaces, you can achieve static typing, making it easier to catch errors during development and improve the maintainability of your code.
1.2 Why use Interfaces?
Interfaces play a significant role in ensuring clear communication and consistency in a TypeScript project. They allow you to define a clear contract between different parts of the code, making it easier for developers to understand how various components interact with each other. Additionally, interfaces enable better IDE support, as editors can provide auto-completions and suggestions based on the defined interface.
2. Defining Interfaces
2.1 Syntax of Interface
In TypeScript, you define an interface using the interface keyword, followed by the name of the interface and the curly braces containing its members. Here’s a simple example of defining an interface for a Person object:
typescript interface Person { name: string; age: number; }
In this example, we have defined an interface called Person, which requires objects to have both name and age properties, where name must be of type string, and age must be of type number.
2.2 Properties in Interfaces
Interfaces can have properties with different data types, including primitive types, objects, functions, and even other interfaces. For instance:
typescript interface Book { title: string; author: { name: string; nationality: string; }; pages: number; isAvailable: boolean; }
Here, the Book interface defines properties such as title of type string, author of type { name: string, nationality: string }, pages of type number, and isAvailable of type boolean.
2.3 Optional Properties
In some cases, you might have properties that are not mandatory for an object to conform to the interface. You can make these properties optional by adding a ? after the property name.
typescript interface Song { title: string; artist: string; durationInSeconds?: number; album?: string; }
The durationInSeconds and album properties in the Song interface are optional, meaning they may or may not be present in objects that implement this interface.
2.4 Readonly Properties
In certain scenarios, you might want to ensure that the properties of an object remain constant once set. TypeScript allows you to define readonly properties in an interface:
typescript interface Circle { readonly radius: number; }
Once you set the radius property of an object, you won’t be able to modify it later.
2.5 Function Signatures in Interfaces
Interfaces can also describe the shape of functions. This is particularly useful when you want to enforce that an object must have specific functions.
typescript interface MathOperations { add(x: number, y: number): number; subtract(x: number, y: number): number; }
The MathOperations interface requires objects to implement the add and subtract functions, taking two arguments of type number and returning a number.
3. Extending Interfaces
Interfaces can inherit from other interfaces, allowing you to compose and extend functionality.
3.1 Inheritance with Interfaces
You can use the extends keyword to create a new interface that inherits from another interface:
typescript interface Animal { species: string; makeSound(): void; } interface Dog extends Animal { breed: string; }
Here, the Dog interface extends the Animal interface, adding a breed property. Any object that implements Dog must also include the properties and methods defined in the Animal interface.
3.2 Combining Multiple Interfaces
In TypeScript, you can implement multiple interfaces in a single class or object:
typescript interface Printable { print(): void; } interface Readable { read(): void; } class Document implements Printable, Readable { // Implement print and read methods here }
The Document class implements both the Printable and Readable interfaces, requiring it to provide implementations for both print() and read() methods.
3.3 Extending Built-in Types
You can extend not only other interfaces but also built-in types in TypeScript:
typescript interface EnhancedArray extends Array<number> { sum(): number; } const numbers: EnhancedArray = [1, 2, 3, 4, 5]; const totalSum = numbers.sum(); // Calling custom method sum()
In this example, we extend the native Array<number> type with a custom method sum(), allowing arrays of numbers to access this new functionality.
4. Implementing Interfaces
Interfaces are not just for defining shapes; they are also useful for enforcing contracts on classes.
4.1 Implementing Interfaces in Classes
To implement an interface in a class, you use the implements keyword and provide the required members:
typescript interface Shape { area(): number; } class Circle implements Shape { constructor(private radius: number) {} area(): number { return Math.PI * this.radius * this.radius; } }
The Circle class implements the Shape interface, which requires the class to have an area() method.
4.2 Ensuring Class Compliance
When a class implements an interface, TypeScript ensures that the class adheres to the contract defined by the interface. If the class doesn’t implement all the required members, TypeScript will raise an error.
4.3 Using Multiple Interfaces in Classes
As mentioned earlier, a class can implement multiple interfaces:
typescript interface Flyable { fly(): void; } interface Swimmable { swim(): void; } class Duck implements Flyable, Swimmable { // Implement fly and swim methods here }
The Duck class implements both the Flyable and Swimmable interfaces, requiring it to provide implementations for both fly() and swim() methods.
5. Interfaces for Function Types
Interfaces can also describe the structure of function types, allowing you to define contracts for functions.
5.1 Typing Function Parameters
You can specify the types of parameters a function should take in the interface:
typescript interface Calculator { (x: number, y: number): number; } const add: Calculator = (a, b) => a + b; const subtract: Calculator = (a, b) => a - b;
Here, the Calculator interface enforces that any function assigned to variables add and subtract must take two arguments of type number and return a number.
5.2 Defining Function Signatures
Interfaces can also describe function signatures with named properties:
typescript interface MathOperations { add(x: number, y: number): number; subtract(x: number, y: number): number; }
The MathOperations interface requires objects to implement the add and subtract functions, taking two arguments of type number and returning a number.
5.3 Implementing Function Interfaces
You can enforce a function to adhere to an interface by using the interface as a type annotation for the function:
typescript interface Printer { (content: string): void; } function printToConsole(content: string): void { console.log(content); } const printer: Printer = printToConsole;
The printer variable enforces that the function assigned to it must take a string argument and return void.
6. Interfaces for Arrays and Index Signatures
6.1 Typed Arrays
Interfaces can describe the structure of arrays, ensuring that elements adhere to specific types:
typescript interface Point { x: number; y: number; } interface PointArray { [index: number]: Point; } const points: PointArray = [ { x: 0, y: 0 }, { x: 1, y: 1 }, { x: 2, y: 3 }, ];
In this example, the PointArray interface enforces that the points array contains objects with x and y properties of type number.
6.2 Index Signatures for Dynamic Properties
You can also use index signatures to define dynamic property names in an interface:
typescript interface Config { [key: string]: any; } const appConfig: Config = { theme: "dark", maxRetries: 3, analyticsEnabled: true, };
The Config interface allows objects to have any number of properties with dynamic keys and any value type.
7. Interfaces vs. Type Aliases
TypeScript offers two primary ways of defining contracts: interfaces and type aliases. Understanding the differences between them is essential for making the right choice.
7.1 Understanding the Differences
Interfaces are mainly used for defining object shapes and function contracts. They can be extended and implemented by classes and objects. You can also merge multiple interface declarations with the same name.
Type Aliases are more versatile and can represent any type, not just object shapes. They are useful for creating unions, intersections, and providing more complex type structures. Type aliases are also easier to create for certain types, such as tuple types.
7.2 When to Use Interfaces or Type Aliases
Choose interfaces when:
- You need to define an object shape.
- You want to implement an object’s contract in a class or multiple classes.
- You intend to extend an existing interface.
Choose type aliases when:
- You need to define complex types that are not just object shapes.
- You want to create unions or intersections of types.
- You have specific use cases like tuple types.
8. Practical Examples
Let’s see how we can use interfaces in practical scenarios.
8.1 Building a Geometric Shapes Library
Consider a library that handles various geometric shapes. We can define interfaces for different shapes:
typescript interface Shape { area(): number; } interface Rectangle extends Shape { width: number; height: number; } interface Circle extends Shape { radius: number; } class CircleShape implements Circle { constructor(public radius: number) {} area(): number { return Math.PI * this.radius * this.radius; } } class RectangleShape implements Rectangle { constructor(public width: number, public height: number) {} area(): number { return this.width * this.height; } }
By using interfaces, the library enforces that all shape objects have the area() method, promoting consistency and preventing errors.
8.2 Defining a Todo App Data Structure
For a todo app, we can define an interface to represent a single todo item:
typescript interface Todo { id: number; title: string; description?: string; completed: boolean; }
The Todo interface ensures that all todo items have an id, title, completed, and an optional description.
8.3 Creating a Configurable Logger
In a logging library, we can define an interface to represent different loggers:
typescript interface Logger { log(message: string): void; } class ConsoleLogger implements Logger { log(message: string): void { console.log(message); } } class FileLogger implements Logger { log(message: string): void { // Implement file logging logic } }
By using the Logger interface, the application can switch between different logging mechanisms easily.
Conclusion
TypeScript interfaces provide a powerful tool for defining contracts between different parts of your code. By using interfaces, you can ensure clear communication, improve type safety, and enhance the maintainability of your projects. We explored the basics of defining interfaces, extending them, implementing them in classes, and practical examples that demonstrate their real-world usage. So, the next time you start a TypeScript project, consider making good use of interfaces to build a more robust and error-free application!
Table of Contents
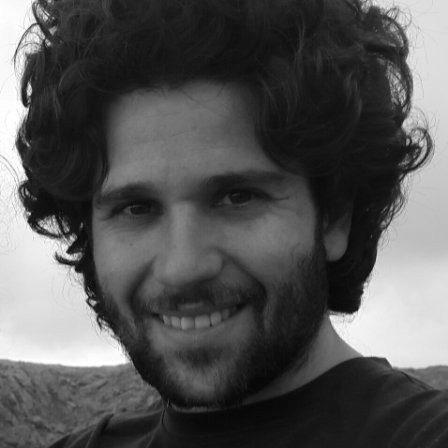
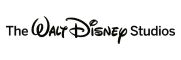