Exploring Reactive Programming with RxJS and TypeScript
In the rapidly evolving landscape of modern web development, creating responsive and interactive applications has become a necessity rather than a luxury. Traditional programming paradigms often struggle to keep up with the demand for real-time updates and dynamic user experiences. This is where Reactive Programming comes into play, offering a paradigm shift that empowers developers to efficiently handle asynchronous data streams and build highly responsive applications. In this blog post, we will delve into the world of Reactive Programming using RxJS and TypeScript, exploring its concepts, benefits, and providing code samples to showcase its power.
Table of Contents
1. Understanding Reactive Programming
1.1. What is Reactive Programming?
At its core, Reactive Programming is a programming paradigm that focuses on asynchronous data streams and the propagation of changes. Instead of dealing with data updates imperatively, Reactive Programming allows developers to define how the data should react to changes and events over time. This results in a more declarative approach to handling asynchronous operations, making the codebase more readable and maintainable.
1.2. Why Choose Reactive Programming?
Reactive Programming offers several benefits that are particularly valuable in the context of modern web development:
- Responsiveness: Reactive Programming enables the creation of applications that respond to changes and events in real-time, delivering a seamless and dynamic user experience.
- Modularity: By breaking down complex logic into smaller, composable units, Reactive Programming promotes modularity and code reusability.
- Asynchronous Simplicity: Managing asynchronous operations, such as data fetching or user interactions, becomes simpler and more manageable with Reactive Programming.
- Error Handling: Reactive streams provide elegant error handling mechanisms, making it easier to handle exceptions and unexpected behaviors in a systematic manner.
2. Getting Started with RxJS and TypeScript
2.1. Installing RxJS
Before diving into the world of Reactive Programming with RxJS and TypeScript, you need to install the RxJS library. You can install it using npm or yarn:
bash npm install rxjs # or yarn add rxjs
2.2. Creating Observables
In RxJS, Observables are the building blocks of Reactive Programming. An Observable represents a sequence of values that can be observed over time. Here’s how you can create a simple Observable:
typescript import { Observable } from 'rxjs'; const myObservable = new Observable((observer) => { observer.next('Hello'); observer.next('World'); observer.complete(); });
2.3. Subscribing to Observables
To observe the values emitted by an Observable, you need to subscribe to it. Subscribing creates a connection between the Observable and an Observer, allowing you to receive and react to emitted values:
typescript myObservable.subscribe({ next: (value) => console.log(value), complete: () => console.log('Observable completed'), });
3. Core Concepts of RxJS
3.1. Observers and Observables
In RxJS, Observers are objects that react to the emissions from Observables. Observables emit values over time, and Observers define how to handle these emissions. The subscribe method establishes the connection between Observables and Observers.
3.2. Operators
Operators are the heart of RxJS, enabling you to transform, filter, and manipulate data emitted by Observables. They allow you to perform operations like mapping, filtering, and reducing without the need for complex nested callbacks.
typescript import { of } from 'rxjs'; import { map, filter } from 'rxjs/operators'; const numbersObservable = of(1, 2, 3, 4, 5); numbersObservable .pipe( filter((num) => num % 2 === 0), map((num) => num * 2) ) .subscribe((result) => console.log(result)); // Output: 4, 8
3.3. Subjects
Subjects act as both Observables and Observers. They allow multicasting, which means multiple Observers can subscribe to a single Subject, receiving the same sequence of emissions.
typescript import { Subject } from 'rxjs'; const subject = new Subject<number>(); subject.subscribe({ next: (value) => console.log(`Observer 1: ${value}`), }); subject.next(1); // Output: Observer 1: 1 subject.subscribe({ next: (value) => console.log(`Observer 2: ${value}`), }); subject.next(2); // Output: Observer 1: 2, Observer 2: 2
3.4. Streams and Pipelines
Reactive Programming involves creating data pipelines using Observables and Operators. Data flows through these pipelines, undergoing transformations at each stage. This approach provides a clear and maintainable way to handle asynchronous operations.
4. Building Responsive Applications
4.1. Event Handling the Reactive Way
In traditional event-driven programming, handling UI events often involves managing callbacks and synchronization. With RxJS, you can create Observables from UI events and process them in a more declarative manner:
typescript import { fromEvent } from 'rxjs'; const button = document.getElementById('myButton'); const buttonClicks = fromEvent(button, 'click'); buttonClicks.subscribe(() => console.log('Button clicked'));
4.2. Real-time Data Fetching
Reactive Programming shines when dealing with real-time data. You can easily create Observables that fetch data from APIs and update your UI reactively:
typescript import { Observable } from 'rxjs'; function fetchData(): Observable<string> { return new Observable((observer) => { fetch('https://api.example.com/data') .then((response) => response.json()) .then((data) => { observer.next(data); observer.complete(); }) .catch((error) => observer.error(error)); }); } fetchData().subscribe({ next: (data) => console.log(data), error: (error) => console.error(error), });
4.3. Managing UI State Reactively
UI state management can become complex as applications grow. Reactive Programming simplifies this by representing UI state as Observables that emit updates:
typescript import { BehaviorSubject } from 'rxjs'; const appState = new BehaviorSubject<string>('initial state'); appState.subscribe((state) => console.log(`Current state: ${state}`)); appState.next('updated state'); // Output: Current state: updated state
5. Handling Asynchronous Operations
5.1. Dealing with Callback Hell
Callback Hell, also known as the Pyramid of Doom, occurs when multiple levels of nested callbacks make the code difficult to read and maintain. Reactive Programming addresses this issue by providing a cleaner way to handle asynchronous operations.
5.2. Promises vs. Observables
Promises and Observables both handle asynchronous operations, but Observables offer more features and flexibility. Observables can emit multiple values over time and have powerful operators for transforming data streams.
5.3. Error Handling and Retry Strategies
RxJS provides elegant error handling mechanisms, allowing you to catch and handle errors gracefully. You can also implement retry strategies to automatically attempt an operation again in case of failure.
6. Combining and Transforming Data Streams
6.1. Using Operators for Transformation
RxJS Operators provide a rich set of functions for transforming data streams. You can use these operators to modify, filter, and combine Observables to achieve complex data processing tasks.
6.2. Merging and Concatenating Streams
Merging and concatenating streams allow you to combine multiple Observables into a single stream. This is useful for scenarios where you need to handle data from different sources.
6.3. Filtering Data with RxJS Operators
Operators like filter, map, and reduce enable you to manipulate the data emitted by Observables. These operators promote a more functional and declarative approach to data processing.
7. Handling Memory and Resource Management
7.1. Unsubscribing and Memory Leaks
When subscribing to Observables, it’s crucial to unsubscribe when you’re done to prevent memory leaks. RxJS provides mechanisms to automatically manage subscriptions, ensuring resources are released properly.
7.2. Strategies for Resource Cleanup
RxJS offers strategies like the takeUntil operator and the unsubscribe lifecycle hook to manage subscriptions effectively. These strategies help prevent lingering subscriptions and resource leaks.
8. Testing and Debugging Reactive Code
8.1. Writing Unit Tests for Observables
RxJS Observables can be easily tested using tools like Jasmine or Jest. You can mock Observables and test how they react to different inputs and scenarios.
8.2. Debugging Techniques with RxJS
Debugging reactive code can be challenging due to its asynchronous nature. RxJS offers debugging tools like tap and do operators, as well as browser extensions for visualizing data flows.
8.3. Using Marble Diagrams for Visualization
Marble diagrams are a powerful way to visualize the behavior of Observables and their operators over time. They help you understand and communicate complex data transformations.
Conclusion
In conclusion, Reactive Programming with RxJS and TypeScript offers a robust and elegant solution for handling asynchronous data streams and building responsive applications. By embracing its concepts and harnessing the power of Observables and Operators, developers can create codebases that are more maintainable, modular, and efficient. Whether you’re building real-time applications, managing UI state, or processing complex data streams, RxJS empowers you to take control of asynchronous operations in a more declarative and organized manner. So dive into the world of Reactive Programming, and unlock the potential of building truly dynamic and responsive web applications.
Table of Contents
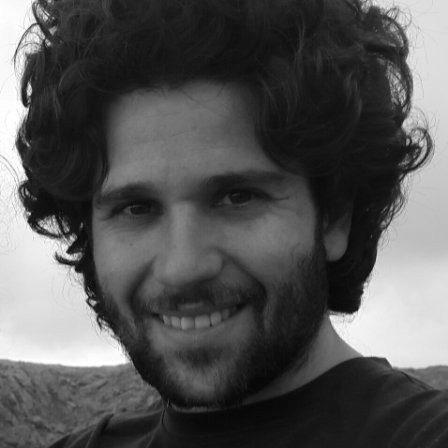
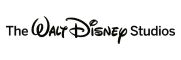