Vue.js Authentication with JWT: Implementing Secure User Sessions
In the world of web development, ensuring secure user authentication and session management is paramount. With the rise of single-page applications (SPAs) and client-side frameworks like Vue.js, implementing robust authentication mechanisms becomes both crucial and challenging. One popular method for securing user sessions in Vue.js applications is through JSON Web Tokens (JWT). In this guide, we’ll explore how to implement secure user sessions in Vue.js using JWT.
Understanding JWT Authentication
JSON Web Tokens (JWT) have become a standard for token-based authentication on the web. JWTs consist of three parts: a header, a payload, and a signature. They are cryptographically signed to verify their authenticity, making them a secure method for transmitting information between parties.
Benefits of JWT Authentication
- Stateless: JWTs are stateless, meaning the server does not need to store session data for authenticated users. This scalability is especially beneficial for applications with high traffic.
- Secure: JWTs are signed using a secret key, ensuring that the token has not been tampered with. This provides a high level of security for user authentication.
- Versatile: JWTs can contain any amount of data in their payload, allowing developers to include custom user information or permissions.
Implementing JWT Authentication in Vue.js
To implement JWT authentication in a Vue.js application, we’ll need to perform the following steps:
- User Authentication: When a user logs in to the application, the server generates a JWT containing the user’s information and sends it back to the client.
- Token Storage: The client stores the JWT securely, typically in local storage or a cookie.
- Protected Routes: Certain routes in the application may require authentication. We’ll need to check for a valid JWT before allowing access to these routes.
- Token Expiration: JWTs can have an expiration time to mitigate security risks. When a JWT expires, the user will need to log in again to obtain a new token.
Let’s delve into each step with examples:
1. User Authentication
// Example Vue.js code for user login
async login() { try { const response = await axios.post('/api/login', { email, password }); const token = response.data.token; localStorage.setItem('jwt', token); // Redirect or perform additional actions upon successful login } catch (error) { console.error('Login failed:', error); } }
2. Token Storage
// Storing JWT in local storage localStorage.setItem('jwt', token); // Retrieving JWT from local storage const token = localStorage.getItem('jwt');
3. Protected Routes
// Example Vue Router configuration for protected routes
import Vue from 'vue'; import Router from 'vue-router'; Vue.use(Router); const router = new Router({ routes: [ { path: '/dashboard', component: Dashboard, meta: { requiresAuth: true } // Mark route as requiring authentication }, // Other routes ] }); router.beforeEach((to, from, next) => { const token = localStorage.getItem('jwt'); if (to.matched.some(route => route.meta.requiresAuth) && !token) { next('/login'); // Redirect to login page if token is missing for protected route } else { next(); } }); export default router;
4. Token Expiration
// Example code to check token expiration const token = localStorage.getItem('jwt'); const decodedToken = jwt_decode(token); const currentTime = Date.now() / 1000; if (decodedToken.exp < currentTime) { // Token has expired, user needs to log in again }
Conclusion
Implementing JWT authentication in Vue.js applications provides a secure and efficient way to manage user sessions. By following best practices and leveraging the versatility of JWTs, developers can create robust authentication systems that enhance the security and usability of their applications.
Remember to handle JWTs with care, implement proper error handling, and regularly audit your authentication system for potential vulnerabilities.
External Resources
- JWT.io – Official website for JSON Web Tokens with comprehensive documentation and tools.
- Vue Router – Official documentation for Vue Router, essential for managing routes in Vue.js applications.
- Axios – Axios is a promise-based HTTP client for JavaScript that can be used in both browsers and Node.js environments.
With these tools and techniques, you can confidently implement secure user sessions in your Vue.js applications using JWT authentication. Happy coding!
Table of Contents
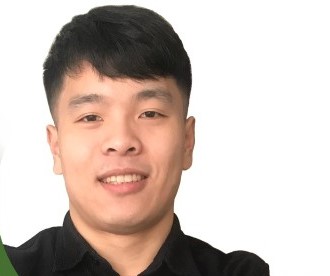
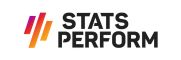