Vue.js Authentication with OAuth: Integrating Third-Party Login Providers
In modern web applications, OAuth is a popular framework for enabling secure authorization and authentication. By integrating third-party login providers, you can streamline user sign-ins and enhance security. Vue.js, with its flexible architecture and robust ecosystem, makes it easy to implement OAuth authentication. This guide explores how to use Vue.js for integrating various third-party login providers using OAuth.
Understanding OAuth Authentication
OAuth (Open Authorization) is a protocol that allows applications to access user data without exposing user credentials. It enables users to log in using their accounts from services like Google, Facebook, or GitHub, providing a secure and seamless authentication process.
Integrating OAuth in Vue.js
Vue.js provides a reactive and component-based framework that can be effectively utilized to manage OAuth authentication. Here’s how you can set up OAuth integration in a Vue.js application.
1. Setting Up OAuth Providers
Before integrating OAuth into your Vue.js app, you need to configure your OAuth providers. Register your application with the provider (e.g., Google, Facebook) to obtain the necessary client ID and client secret.
2. Installing Required Libraries
To handle OAuth in Vue.js, you can use libraries like `vue-authenticate` and `axios`. Install these libraries via npm:
```bash npm install vue-authenticate axios ```
3. Configuring OAuth in Vue.js
Create a Vue plugin to configure OAuth providers. For this example, we’ll set up Google authentication.
Example: Configuring OAuth Plugin
```javascript // src/plugins/auth.js import Vue from 'vue'; import VueAuthenticate from 'vue-authenticate'; import axios from 'axios'; Vue.use(VueAuthenticate, { baseUrl: 'http://your-backend-api.com', // Your API endpoint providers: { google: { clientId: 'YOUR_GOOGLE_CLIENT_ID', redirectUri: 'http://localhost:8080/auth/callback', }, }, }); ```
4. Handling Authentication Flow
Use Vue Router to handle authentication callbacks and manage the login process.
Example: Adding Authentication Routes
```javascript // src/router/index.js import Vue from 'vue'; import Router from 'vue-router'; import Home from '@/components/Home'; import AuthCallback from '@/components/AuthCallback'; Vue.use(Router); export default new Router({ routes: [ { path: '/', name: 'Home', component: Home, }, { path: '/auth/callback', name: 'AuthCallback', component: AuthCallback, }, ], }); ```
Example: AuthCallback Component
```javascript // src/components/AuthCallback.vue <template> <div> <h1>Authenticating...</h1> </div> </template> <script> export default { mounted() { this.$auth .getAuthResponse() .then(response => { // Handle successful authentication console.log(response); this.$router.push('/'); }) .catch(error => { // Handle authentication error console.error(error); }); }, }; </script> ```
5. Protecting Routes with Authentication
Ensure that only authenticated users can access certain routes by using navigation guards in Vue Router.
Example: Adding Route Guards
```javascript // src/router/index.js import Vue from 'vue'; import Router from 'vue-router'; import Home from '@/components/Home'; import AuthCallback from '@/components/AuthCallback'; Vue.use(Router); const router = new Router({ routes: [ { path: '/', name: 'Home', component: Home, meta: { requiresAuth: true }, }, { path: '/auth/callback', name: 'AuthCallback', component: AuthCallback, }, ], }); router.beforeEach((to, from, next) => { if (to.matched.some(record => record.meta.requiresAuth)) { if (!Vue.prototype.$auth.isAuthenticated()) { next({ name: 'AuthCallback' }); } else { next(); } } else { next(); } }); export default router; ```
6. Handling User Data
Once authenticated, you can fetch user information from the provider and use it within your application.
Example: Fetching User Data
```javascript // src/components/UserProfile.vue <template> <div> <h1>User Profile</h1> <p>Name: {{ user.name }}</p> <p>Email: {{ user.email }}</p> </div> </template> <script> export default { data() { return { user: {}, }; }, mounted() { this.$auth .getUserInfo() .then(user => { this.user = user; }) .catch(error => { console.error(error); }); }, }; </script> ```
Conclusion
Integrating OAuth authentication into your Vue.js application enhances both security and user experience. By using OAuth providers like Google or Facebook, you can streamline the authentication process and offer users a seamless login experience. With the tools and examples provided, you should be able to implement OAuth authentication effectively in your Vue.js projects.
Further Reading:
Table of Contents
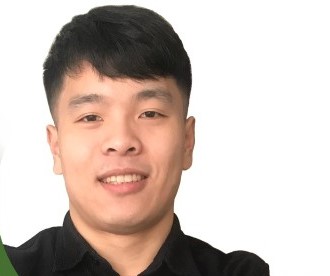
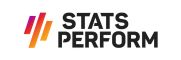