Creating Custom Directives in Vue.js: Extending the Framework’s Functionality
Vue.js is a popular JavaScript framework for building user interfaces and single-page applications. One of its powerful features is the ability to create custom directives, which allow developers to extend the framework’s functionality and add reusable behavior to their applications. In this article, we’ll explore the concept of custom directives in Vue.js and demonstrate how to create them with examples.
Understanding Directives in Vue.js
In Vue.js, directives are special tokens in the markup that tell the library to do something to a DOM element. They are prefixed with v-, followed by the directive name. Vue.js provides built-in directives like v-if, v-for, and v-bind for common tasks like conditional rendering, iterating over arrays, and binding data to attributes.
Creating Custom Directives
To create a custom directive in Vue.js, we use the Vue.directive() method. This method takes two arguments: the directive name and an object containing hook functions. These hook functions are called when the directive is bound to an element, inserted into the DOM, updated, or unbound.
Example 1: Custom Directive for Focus Management
Suppose we want to create a custom directive called v-focus that automatically focuses an input element when the page loads. Here’s how we can define this directive:
Vue.directive('focus', { inserted: function (el) { el.focus(); } });
Now, we can use this directive in our Vue templates like this:
<input type="text" v-focus>
Example 2: Custom Directive for Click Outside Detection
Another common use case for custom directives is detecting clicks outside of a specific element. Let’s create a directive called v-click-outside that triggers a callback when a click occurs outside of the element it’s bound to:
Vue.directive('click-outside', { bind: function (el, binding, vnode) { el.clickOutsideEvent = function (event) { if (!(el === event.target || el.contains(event.target))) { vnode.context[binding.expression](event); } }; document.body.addEventListener('click', el.clickOutsideEvent); }, unbind: function (el) { document.body.removeEventListener('click', el.clickOutsideEvent); } }); Now, we can use this directive in our Vue templates to handle click outside events: <div v-click-outside="handleClickOutside"> Click outside this div to trigger the event. </div>
Example 3: Custom Directive for Infinite Scrolling
Let’s create a custom directive called v-infinite-scroll that triggers a callback when the user scrolls to the bottom of a container. This is useful for implementing infinite scrolling functionality in our applications:
Vue.directive('infinite-scroll', { bind: function (el, binding) { el.addEventListener('scroll', function () { if (el.scrollHeight - el.scrollTop <= el.clientHeight) { binding.value(); } }); } });
Now, we can use this directive in our Vue templates to implement infinite scrolling:
<div v-infinite-scroll="loadMoreItems"> <!-- Content goes here --> </div>
Conclusion
Custom directives in Vue.js provide a powerful way to extend the framework’s functionality and add reusable behavior to our applications. By defining custom directives, developers can encapsulate complex interactions and make their code more modular and maintainable. Whether it’s managing focus, detecting clicks outside of elements, or implementing infinite scrolling, custom directives allow us to enhance the user experience and build more robust Vue.js applications.
External Resources
Table of Contents
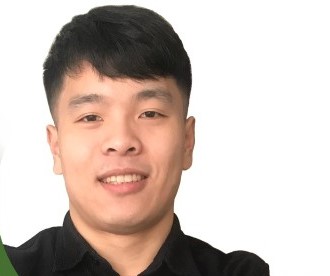
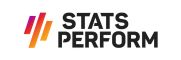