Vue.js and D3.js Integration: Visualizing Data in Your Web App
In the realm of web development, harnessing the power of data visualization can transform a mundane user experience into an engaging and insightful journey. As developers, we’re often faced with the challenge of effectively presenting complex data in a visually appealing manner. This is where the integration of Vue.js and D3.js comes into play, offering a potent combination for creating stunning data visualizations within your web applications.
Why Vue.js and D3.js?
Vue.js: Vue.js has gained widespread popularity among developers due to its simplicity, flexibility, and performance. It’s a progressive JavaScript framework that excels in building user interfaces and single-page applications. With its reactive data binding and component-based architecture, Vue.js provides an intuitive way to manage the state of your application.
D3.js: D3.js, short for Data-Driven Documents, is a powerful JavaScript library for manipulating documents based on data. It allows you to bind data to the DOM (Document Object Model) and apply data-driven transformations to create dynamic and interactive visualizations. From simple bar charts to complex network diagrams, D3.js empowers you to bring your data to life in the browser.
Integration Guide
1. Setting Up Your Project
To begin, make sure you have Vue.js and D3.js installed in your project. You can include them via CDN links in your HTML file or use package managers like npm or yarn to install them directly into your project directory.
<!-- Vue.js CDN --> <script src="https://cdn.jsdelivr.net/npm/vue@2"></script> <!-- D3.js CDN --> <script src="https://d3js.org/d3.v7.min.js"></script>
2. Creating a Vue Component
Next, let’s create a Vue component where we’ll integrate D3.js for data visualization. Here’s a basic example of a Vue component structure:
<template> <div class="data-visualization"> <!-- SVG container for D3.js --> <svg id="chart"></svg> </div> </template> <script> export default { name: 'DataVisualization', mounted() { // D3.js code for creating visualizations // Example: D3.js bar chart const data = [10, 20, 30, 40, 50]; const svg = d3.select("#chart") .attr("width", 400) .attr("height", 200); svg.selectAll("rect") .data(data) .enter() .append("rect") .attr("x", (d, i) => i * 70) .attr("y", (d) => 200 - d) .attr("width", 65) .attr("height", (d) => d) .attr("fill", "skyblue"); } } </script> <style scoped> .data-visualization { margin: 20px; } </style>
3. Integrating D3.js in Vue Component
In the mounted lifecycle hook of our Vue component, we can leverage D3.js to create various types of visualizations. In the example above, we’ve created a simple bar chart using D3.js. You can customize this code to generate different types of charts such as line charts, pie charts, or scatter plots based on your data and requirements.
4. Styling and Interactivity
Once you’ve integrated D3.js within your Vue component, you can enhance the visualization by adding CSS styles for better aesthetics and implementing interactivity using D3.js event listeners. This allows users to interact with the visualization, such as hovering over data points for additional information or clicking to trigger actions.
Conclusion
By integrating Vue.js and D3.js, you can unlock endless possibilities for visualizing data in your web applications. Whether you’re building a dashboard, analytics tool, or data-driven storytelling platform, this powerful combination empowers you to create compelling and meaningful visualizations that captivate your audience.
External Resources
Start exploring the fusion of Vue.js and D3.js today, and elevate your web app’s data visualization game to new heights!
Table of Contents
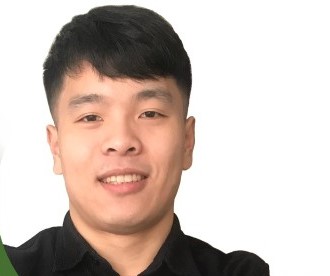
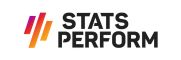