Vue.js File Uploads: Handling User File Input in Your App
File uploads are a common feature in web applications, allowing users to submit documents, images, and other types of files. In Vue.js, handling file uploads involves managing user input, processing files, and integrating with backend services. This guide will explore how to implement and manage file uploads in a Vue.js application, complete with practical examples.
Understanding File Uploads in Vue.js
Handling file uploads involves creating a user interface that allows file selection and then processing and sending these files to a server. Vue.js provides reactive data handling and component-based architecture, making it a good choice for implementing file upload functionality.
Using Vue.js for File Uploads
Vue.js offers a straightforward way to handle file inputs and upload files to a server. Below are some key aspects and code examples demonstrating how to manage file uploads in Vue.js.
1. Creating a File Upload Component
The first step is to create a component that includes a file input element. This component will handle file selection and trigger the upload process.
Example: File Upload Component
```html <template> <div> <input type="file" @change="handleFileUpload" /> <button @click="uploadFile">Upload</button> </div> </template> <script> export default { data() { return { selectedFile: null }; }, methods: { handleFileUpload(event) { this.selectedFile = event.target.files[0]; }, async uploadFile() { if (this.selectedFile) { const formData = new FormData(); formData.append('file', this.selectedFile); try { const response = await fetch('https://your-backend-api/upload', { method: 'POST', body: formData }); const result = await response.json(); console.log('File uploaded successfully:', result); } catch (error) { console.error('Error uploading file:', error); } } else { console.error('No file selected'); } } } }; </script> ```
2. Handling File Uploads on the Backend
Once the file is uploaded from the client-side, you need to handle it on the server. This usually involves saving the file to a specific directory and processing it as needed.
Example: Express.js Backend for File Uploads
```javascript const express = require('express'); const multer = require('multer'); const path = require('path'); const app = express(); const upload = multer({ dest: 'uploads/' }); app.post('/upload', upload.single('file'), (req, res) => { console.log('File received:', req.file); res.json({ message: 'File uploaded successfully' }); }); app.listen(3000, () => { console.log('Server running on port 3000'); }); ```
3. Displaying Uploaded Files
After uploading files, you might want to display them in your Vue.js app. You can achieve this by showing previews of images or listing uploaded documents.
Example: Displaying an Image Preview
```html <template> <div> <input type="file" @change="handleFileUpload" /> <img v-if="imageSrc" :src="imageSrc" alt="Image Preview" /> </div> </template> <script> export default { data() { return { imageSrc: null }; }, methods: { handleFileUpload(event) { const file = event.target.files[0]; if (file) { this.imageSrc = URL.createObjectURL(file); } } } }; </script> ```
4. Adding Progress Indicators
To improve user experience, you can add progress indicators to show the status of the file upload process.
Example: File Upload Progress
```html <template> <div> <input type="file" @change="handleFileUpload" /> <button @click="uploadFile">Upload</button> <div v-if="uploadProgress !== null">Upload Progress: {{ uploadProgress }}%</div> </div> </template> <script> export default { data() { return { selectedFile: null, uploadProgress: null }; }, methods: { handleFileUpload(event) { this.selectedFile = event.target.files[0]; }, async uploadFile() { if (this.selectedFile) { const formData = new FormData(); formData.append('file', this.selectedFile); const xhr = new XMLHttpRequest(); xhr.open('POST', 'https://your-backend-api/upload', true); xhr.upload.onprogress = (event) => { if (event.lengthComputable) { this.uploadProgress = Math.round((event.loaded / event.total) * 100); } }; xhr.onload = () => { if (xhr.status === 200) { console.log('File uploaded successfully'); this.uploadProgress = null; } else { console.error('Error uploading file'); } }; xhr.send(formData); } else { console.error('No file selected'); } } } }; </script> ```
Conclusion
Handling file uploads in Vue.js involves creating user-friendly interfaces for file selection, processing uploads, and displaying results. By utilizing Vue.js’s reactive data handling and integrating with backend services, you can efficiently manage file uploads and enhance user experiences in your web applications.
Further Reading
Table of Contents
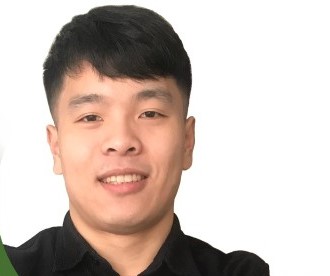
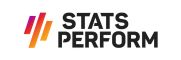