Vue.js and Firebase Authentication: Securely Managing User Accounts
User authentication is a critical aspect of modern web applications, ensuring that only authorized users have access to certain features and data. Firebase Authentication, combined with Vue.js, offers a powerful solution for managing user accounts securely. This blog will delve into how to leverage Vue.js with Firebase Authentication to build a robust and secure authentication system.
Understanding Firebase Authentication
Firebase Authentication provides backend services to help authenticate users in web and mobile apps. It supports various authentication methods, including email/password, phone numbers, and third-party providers like Google and Facebook. Firebase simplifies the implementation of user authentication, providing ready-made UI components and secure token management.
Using Vue.js with Firebase Authentication
Vue.js is a popular JavaScript framework for building user interfaces and single-page applications. Its reactive data-binding and component-based architecture make it an excellent choice for integrating with Firebase Authentication. Here’s how you can set up and use Firebase Authentication in a Vue.js application.
1. Setting Up Firebase
First, you need to set up a Firebase project and configure authentication methods. Follow these steps:
1. Create a Firebase Project: Go to the [Firebase Console](https://console.firebase.google.com/) and create a new project.
2. Enable Authentication Providers: Navigate to the Authentication section and enable the desired sign-in methods (e.g., Email/Password, Google).
3. Obtain Firebase Configuration: In the project settings, find your Firebase configuration object. You will need this to initialize Firebase in your Vue.js app.
2. Installing Firebase and VueFire
Add Firebase and VueFire (a library for integrating Firebase with Vue.js) to your Vue.js project.
```bash npm install firebase vuefire ```
3. Configuring Firebase in Vue.js
Create a `firebase.js` file to initialize Firebase and set up authentication.
```javascript // firebase.js import firebase from 'firebase/app'; import 'firebase/auth'; // Your web app's Firebase configuration const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" }; // Initialize Firebase firebase.initializeApp(firebaseConfig); export const auth = firebase.auth(); export default firebase; ```
4. Implementing Authentication in Vue.js
Here’s how to implement user registration and login features in your Vue.js application.
Example: User Registration
```vue <template> <div> <h1>Register</h1> <form @submit.prevent="register"> <input v-model="email" type="email" placeholder="Email" required /> <input v-model="password" type="password" placeholder="Password" required /> <button type="submit">Register</button> </form> </div> </template> <script> import { auth } from './firebase'; export default { data() { return { email: '', password: '' }; }, methods: { async register() { try { await auth.createUserWithEmailAndPassword(this.email, this.password); alert('User registered successfully!'); } catch (error) { console.error('Error registering user:', error); alert('Registration failed.'); } } } }; </script> ```
Example: User Login
```vue <template> <div> <h1>Login</h1> <form @submit.prevent="login"> <input v-model="email" type="email" placeholder="Email" required /> <input v-model="password" type="password" placeholder="Password" required /> <button type="submit">Login</button> </form> </div> </template> <script> import { auth } from './firebase'; export default { data() { return { email: '', password: '' }; }, methods: { async login() { try { await auth.signInWithEmailAndPassword(this.email, this.password); alert('User logged in successfully!'); } catch (error) { console.error('Error logging in user:', error); alert('Login failed.'); } } } }; </script> ```
5. Handling Authentication State
To manage user authentication state across your application, you can use Vue’s reactive properties and watch for changes in the authentication state.
Example: Authentication State Management
```vue <template> <div> <h1 v-if="user">Welcome, {{ user.email }}!</h1> <h1 v-else>Please log in.</h1> </div> </template> <script> import { auth } from './firebase'; export default { data() { return { user: null }; }, created() { auth.onAuthStateChanged(user => { this.user = user; }); } }; </script> ```
Conclusion
Integrating Firebase Authentication with Vue.js provides a streamlined and secure approach to managing user accounts. By leveraging Firebase’s robust authentication features and Vue.js’s reactive capabilities, you can create a secure and user-friendly authentication system for your application.
Further Reading:
Table of Contents
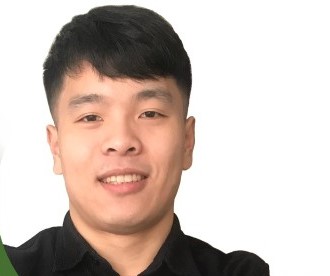
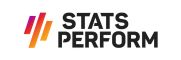