Vue.js and Firebase Realtime Database: Building Real-Time Applications
In today’s fast-paced digital landscape, real-time applications are essential for providing dynamic user experiences. Vue.js, a progressive JavaScript framework, paired with Firebase Realtime Database, offers a powerful combination for building such applications. This blog explores how to use Vue.js and Firebase Realtime Database together to create responsive and interactive applications.
Understanding Firebase Realtime Database
Firebase Realtime Database is a cloud-hosted NoSQL database that enables real-time data synchronization across all clients. It provides a simple way to store and sync data in real-time, making it ideal for applications that require instant updates.
Using Vue.js with Firebase Realtime Database
Vue.js provides an intuitive framework for building modern web applications, and when combined with Firebase Realtime Database, it allows for seamless real-time data updates. Below are some key aspects and code examples demonstrating how Vue.js can be used with Firebase Realtime Database.
1. Setting Up Firebase and Vue.js
Before you start building your application, you need to set up Firebase and integrate it with your Vue.js project. Begin by creating a Firebase project and configuring Firebase in your Vue.js application.
Example: Configuring Firebase in Vue.js
First, install the Firebase SDK:
```bash npm install firebase ```
Then, initialize Firebase in your Vue.js application:
```javascript // src/firebaseConfig.js import firebase from 'firebase/app'; import 'firebase/database'; const firebaseConfig = { apiKey: 'YOUR_API_KEY', authDomain: 'YOUR_PROJECT_ID.firebaseapp.com', databaseURL: 'https://YOUR_PROJECT_ID.firebaseio.com', projectId: 'YOUR_PROJECT_ID', storageBucket: 'YOUR_PROJECT_ID.appspot.com', messagingSenderId: 'YOUR_MESSAGING_SENDER_ID', appId: 'YOUR_APP_ID', }; firebase.initializeApp(firebaseConfig); export const db = firebase.database(); ```
2. Real-Time Data Binding
Vue.js makes it easy to bind real-time data from Firebase to your components. By using Firebase Realtime Database’s on value listener, you can automatically update your Vue components when data changes.
Example: Displaying Real-Time Data in a Vue Component
```javascript // src/components/RealTimeData.vue <template> <div> <h1>Real-Time Data</h1> <ul> <li v-for="item in data" :key="item.id">{{ item.name }}</li> </ul> </div> </template> <script> import { db } from '../firebaseConfig'; export default { data() { return { data: [] }; }, created() { db.ref('items').on('value', snapshot => { const items = []; snapshot.forEach(childSnapshot => { const item = childSnapshot.val(); items.push({ id: childSnapshot.key, ...item }); }); this.data = items; }); } }; </script> ```
3. Adding Data to Firebase
You can also use Firebase Realtime Database to add new data from your Vue.js application. This is useful for applications where users can input and submit data in real-time.
Example: Adding Data with Vue.js
```javascript // src/components/AddData.vue <template> <div> <input v-model="newItem" placeholder="Add new item" /> <button @click="addItem">Add Item</button> </div> </template> <script> import { db } from '../firebaseConfig'; export default { data() { return { newItem: '' }; }, methods: { addItem() { if (this.newItem.trim() !== '') { db.ref('items').push({ name: this.newItem }); this.newItem = ''; } } } }; </script> ```
4. Handling Data Updates and Deletes
Firebase Realtime Database also allows you to handle data updates and deletions, ensuring your application remains in sync with the latest data changes.
Example: Updating and Deleting Data
```javascript // src/components/UpdateDeleteData.vue <template> <div> <button @click="updateItem">Update First Item</button> <button @click="deleteItem">Delete First Item</button> </div> </template> <script> import { db } from '../firebaseConfig'; export default { methods: { updateItem() { db.ref('items').limitToFirst(1).once('value', snapshot => { const firstKey = Object.keys(snapshot.val())[0]; db.ref(`items/${firstKey}`).update({ name: 'Updated Name' }); }); }, deleteItem() { db.ref('items').limitToFirst(1).once('value', snapshot => { const firstKey = Object.keys(snapshot.val())[0]; db.ref(`items/${firstKey}`).remove(); }); } } }; </script> ```
Conclusion
Combining Vue.js with Firebase Realtime Database provides a robust solution for building real-time applications. Whether you’re handling data binding, adding new data, or managing updates and deletions, Vue.js and Firebase offer a seamless experience for creating dynamic, interactive applications. By leveraging these tools effectively, you can build responsive applications that provide a superior user experience.
Further Reading:
Table of Contents
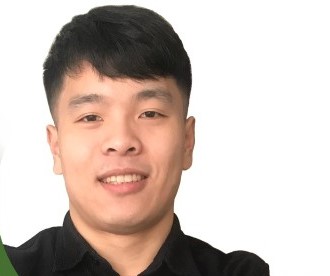
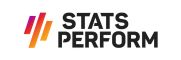