Vue.js Fundamentals: A Comprehensive Guide to Getting Started
In the ever-evolving landscape of modern web development, Vue.js has firmly established itself as one of the prime choices for developers worldwide, making the demand to hire Vue.js developers increasingly significant. Vue.js is a popular JavaScript framework used for building user interfaces. Its simplicity, reusability, and adaptability are some of the reasons why it has been adopted by both small startups and large tech giants like Alibaba, Xiaomi, and Adobe.
In this blog post, we’ll delve into Vue.js’s essential concepts, a valuable resource for those who aim to hire Vue.js developers or for developers wanting to add this skill to their toolkit. By the end of the post, you’ll have a good understanding of Vue.js and will be ready to start your first Vue project or competently engage with and hire Vue.js developers for your team.
Introduction to Vue.js
Vue.js is a progressive framework, meaning you can opt to build an entire single-page application with Vue.js or just use it for a portion of your project where you need a more reactive user interface. It’s straightforward to integrate Vue.js with existing projects, which makes it an excellent choice for developers aiming to incrementally improve their apps.
Vue’s core library focuses solely on the view layer, enabling easy integration with other libraries or existing projects. However, when used in combination with modern tooling and supporting libraries, Vue.js also becomes a powerful option for building sophisticated Single-Page Applications (SPAs).
Setting Up Vue.js
Before we proceed with coding, let’s install Vue.js. The easiest way to start a Vue.js project is by using Vue CLI, a full system for rapid Vue.js development. This toolset is a staple in the toolkit of any Vue.js developer, and knowing how to utilize it is vital for those looking to hire Vue.js developers. You need to have Node.js (version 8.9 or above) installed on your machine. This step is a cornerstone of any Vue.js project setup, and itβs knowledge like this that differentiates novice developers from the seasoned Vue.js developers you might be looking to hire.
First, install Vue CLI globally by running the following command in your terminal:
```shell npm install -g @vue/cli ```
Once Vue CLI is installed, you can create a new project using:
```shell vue create my-vue-app ```
You’ll be prompted to pick a preset. You can either choose the default preset which comes with a basic Babel + ESLint setup or manually select features for a more tailored setup.
Vue.js Project Structure
Once your project is created, navigate to the project directory. Your directory structure should look like this:
```shell my-vue-app |-- node_modules |-- public |-- src | |-- assets | |-- components | |-- App.vue | |-- main.js |-- package.json |-- README.md ```
The `src` folder is where you’ll spend most of your time. It includes `main.js`, the entry point of your application, and `App.vue`, your main Vue component. The `components` folder is where you’ll place all your Vue components.
Vue.js Components and Templates
One of the significant advantages of Vue.js is the ability to create components, reusable pieces of code that can help you structure your application. A Vue component has a template, a script, and (optionally) styles.
Here is a simple Vue component:
```vue <template> <div> <h1>{{ message }}</h1> </div> </template> <script> export default { data() { return { message: 'Hello Vue!' } } } </script> <style scoped> h1 { color: blue; } </style> ```
In this component, we have a simple template with a single data property `message`. In Vue.js, you use double curly braces `{{ }}` to display data in your templates. The `scoped` attribute in the style tag ensures the CSS rules apply only to this component.
Vue.js Directives
Vue.js uses directives – special attributes prefixed with `v-` – to apply special reactive behavior to the DOM. Let’s take a look at some commonly used
directives:
– `v-bind`: The `v-bind` directive is used to bind a property or an attribute to an expression. This is commonly used to bind the `src` attribute of an image tag to a data property, as shown below:
```vue <template> <img v-bind:src="imageUrl" alt="Vue logo"> </template> <script> export default { data() { return { imageUrl: 'https://vuejs.org/images/logo.png' } } } </script> ```
– `v-model`: The `v-model` directive creates two-way data bindings on form input, textarea, and select elements. It automatically updates the element’s value whenever the data property changes and vice versa.
```vue <template> <input v-model="message" placeholder="Enter a message"> <p>Your message: {{ message }}</p> </template> <script> export default { data() { return { message: '' } } } </script> ```
– `v-if`, `v-else-if`, `v-else`: These directives are used to conditionally render elements. An element is only rendered if the directive’s expression returns a truthy value.
```vue <template> <div v-if="loading">Loading...</div> <div v-else-if="error">An error occurred</div> <div v-else>Data loaded successfully</div> </template> <script> export default { data() { return { loading: true, error: false } } } </script> ```
– `v-for`: The `v-for` directive is used to render a list of items based on an array.
```vue <template> <ul> <li v-for="item in items" :key="item.id"> {{ item.name }} </li> </ul> </template> <script> export default { data() { return { items: [ { id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }, { id: 3, name: 'Item 3' } ] } } } </script> ```
Vue.js Lifecycle Hooks
Lifecycle hooks are a crucial part of any Vue.js application. They allow you to know when your component is created, added to the DOM, updated, or destroyed. Vue has several lifecycle hooks, but the most commonly used are:
– `created()`: This hook runs after the instance is created. At this stage, the instance has finished processing the options which means the data is reactive, computed properties are available, and methods, watcher, and event callbacks are set up. However, the mount phase has not been started, and the `$el` property is not available yet.
– `mounted()`: This hook is called after the instance has been mounted. When this hook is invoked, all direct `v-node` children of the Vue instance have also been mounted.
– `updated()`: This hook is called after a data change causes the virtual DOM to be re-rendered and patched. The component’s DOM will have been updated when this hook is called, so you can perform DOM-dependent operations here.
– `destroyed()`: This hook is called after a Vue instance has been destroyed. When this hook is called, all directives of the Vue instance have been unbound, all event listeners have been removed, and all child Vue instances have also been destroyed.
Here’s an example of a Vue component with lifecycle hooks:
```vue <template> <div>{{ count }}</div> </template> <script> export default { data() { return { count: 0 } }, created() { console.log('Component created'); }, mounted() { console.log('Component mounted'); setInterval(() => { this.count++; }, 1000); }, updated() { console.log('Component updated'); }, destroyed() { console.log('Component destroyed'); } } </script> ```
In the example above, we have a counter that increases every second. We’ve used each lifecycle hook to log a message to the console so you can see when each hook is triggered.
Vue Router
Vue Router is the official router for Vue.js. It deeply integrates with Vue.js core to make building Single Page Applications a breeze. To get started with Vue Router, we need to install it first:
```shell npm install vue-router ```
After installing, you can set up routes in your Vue application. Here’s an example:
```js import { createRouter, createWebHistory } from 'vue-router' import Home from '../views/Home.vue' import About from '../views/About.vue' const routes = [ { path: '/', name: 'Home', component: Home }, { path: '/about', name: 'About', component: About } ] const router = createRouter({ history: createWebHistory(process.env.BASE_URL), routes }) export default router ```
In this example, we first import `createRouter` and `createWebHistory` from `vue-router` and our `Home` and `About` components. We then define our routes in a `routes` array, each route being an object specifying the path, name, and component. Finally, we create a new router instance and export it.
We can now use the `<router-link>` component for navigation:
```vue <template> <div> <router-link to="/">Home</router-link> | <router-link to="/about">About</router-link> <router-view/> </div> </template> ```
The `<router-view>` component is a functional component that renders the matched component for the given path.
Vuex for State Management
Vue provides a solution for state management through Vuex. It serves as a centralized store for all components in an application. Vuex is especially helpful when you need to share state between components that are far apart in your application’s component tree.
First, install Vuex using npm:
```shell npm install vuex ```
A Vuex store is pretty straightforward. Here’s an example:
```js import { createStore } from 'vuex' export default createStore({ state: { count: 0 }, mutations: { increment(state) { state.count++ } }, actions: { increment({ commit }) { commit('increment') } } }) ```
In the above example, we first define our state, which is an object that contains the state of our app. Next, we define mutations, which are functions that directly mutate the state. Finally, we define actions, which are functions that commit mutations.
To use Vuex in a component, you can do something like this:
```vue <template> <div> <button @click="increment">Increment</button> <p>Count: {{ $store.state.count }}</p> </div> </template> <script> export default { methods: { increment() { this.$store .dispatch('increment') } } } </script> ```
In the above example, we are calling the `increment` action when the button is clicked, and we’re displaying the count from the state using `$store.state.count`.
Conclusion
Vue.js provides a modern and comprehensive approach to JavaScript-based web development, providing developers with the tools they need to build user-friendly, performance-optimized web applications. This makes it an important skill to have for those looking to hire Vue.js developers. Whether you’re building a simple interface or an ambitious web application, Vue.js has the features and community support to help you succeed.
Remember, learning a new framework or library can feel daunting, especially when it comes with its own set of concepts and syntax. But with practice, patience, and real-world use, you’ll soon find yourself navigating Vue.js like a pro – an invaluable asset for anyone looking to hire Vue.js developers.
This blog post only scratches the surface of what Vue.js can do. However, we’ve covered some core concepts that will provide a solid foundation for you to further explore Vue.js. Whether your goal is to build your own Vue.js project or to hire Vue.js developers, getting to grips with these fundamentals is a crucial first step. If you want to dive deeper into Vue.js, their documentation is incredibly well-written and comprehensive. Happy coding!
Table of Contents
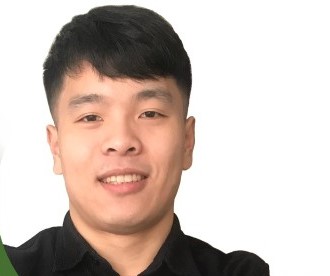
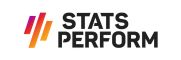