Vue.js and Google Maps Integration: Adding Maps to Your Web App
Integrating Google Maps into your Vue.js application can significantly enhance user experience by providing interactive and dynamic mapping features. Whether you’re building a location-based service, a delivery tracking system, or a property listing platform, Google Maps offers a range of functionalities that can be easily integrated with Vue.js. This article explores how to add Google Maps to your Vue.js application and provides practical examples to get you started.
Understanding Google Maps Integration
Google Maps integration involves embedding maps into your web application and utilizing various features such as markers, routes, and layers. Vue.js, with its reactive components and straightforward data binding, makes it easy to work with Google Maps APIs.
Using Vue.js for Google Maps Integration
Vue.js provides a reactive framework that simplifies the process of integrating and managing Google Maps in your application. Below are key aspects and code examples demonstrating how to integrate Google Maps with Vue.js.
1. Setting Up Google Maps API
To start, you’ll need an API key from Google Cloud Platform. Once you have your API key, you can include the Google Maps JavaScript API in your Vue.js project.
Example: Adding Google Maps Script to Vue Component
In your Vue component, you can dynamically load the Google Maps API script.
```javascript <template> <div id="map" style="height: 500px; width: 100%;"></div> </template> <script> export default { mounted() { const apiKey = 'YOUR_GOOGLE_MAPS_API_KEY'; const script = document.createElement('script'); script.src = `https://maps.googleapis.com/maps/api/js?key=${apiKey}&callback=initMap`; script.async = true; document.head.appendChild(script); window.initMap = this.initMap; }, methods: { initMap() { const mapOptions = { center: { lat: -34.397, lng: 150.644 }, zoom: 8, }; const map = new google.maps.Map(document.getElementById('map'), mapOptions); }, }, }; </script> ```
2. Adding Markers to the Map
Markers are essential for highlighting specific locations on the map. You can add markers to the map by utilizing the Google Maps JavaScript API.
Example: Adding a Marker
```javascript methods: { initMap() { const mapOptions = { center: { lat: -34.397, lng: 150.644 }, zoom: 8, }; const map = new google.maps.Map(document.getElementById('map'), mapOptions); const marker = new google.maps.Marker({ position: { lat: -34.397, lng: 150.644 }, map: map, title: 'Hello World!', }); }, } ```
3. Handling Map Events
Google Maps provides various events such as clicks and drags that can be utilized to enhance user interaction. You can handle these events in your Vue component.
Example: Handling Map Click Events
```javascript methods: { initMap() { const mapOptions = { center: { lat: -34.397, lng: 150.644 }, zoom: 8, }; const map = new google.maps.Map(document.getElementById('map'), mapOptions); google.maps.event.addListener(map, 'click', (event) => { new google.maps.Marker({ position: event.latLng, map: map, title: 'New Marker', }); }); }, } ```
4. Integrating with Vuex for State Management
For more complex applications, you may need to integrate Google Maps with Vuex for managing application state.
Example: Managing Map State with Vuex
```javascript // store.js export default new Vuex.Store({ state: { mapCenter: { lat: -34.397, lng: 150.644 }, }, mutations: { setMapCenter(state, center) { state.mapCenter = center; }, }, actions: { updateMapCenter({ commit }, center) { commit('setMapCenter', center); }, }, }); ```
In your Vue component:
```javascript computed: { mapCenter() { return this.$store.state.mapCenter; }, }, methods: { initMap() { const mapOptions = { center: this.mapCenter, zoom: 8, }; const map = new google.maps.Map(document.getElementById('map'), mapOptions); }, }, ```
Conclusion
Integrating Google Maps with Vue.js can greatly enhance the functionality and user experience of your web application. From setting up the API to adding markers, handling events, and managing state, Vue.js provides a robust framework for working with Google Maps. Leveraging these capabilities will help you create interactive and dynamic mapping features in your applications.
Further Reading:
Table of Contents
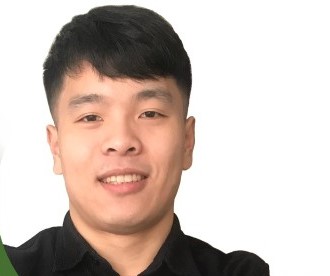
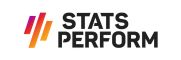