Vue.js and GraphQL: Efficient Data Fetching for Your Web App
In the realm of modern web development, efficiency is the name of the game. With users expecting seamless experiences and lightning-fast response times, developers need tools that allow them to optimize performance without sacrificing functionality. Vue.js and GraphQL have emerged as two powerful technologies that, when combined, offer a compelling solution for efficient data fetching in web applications.
Understanding Vue.js and GraphQL
Vue.js
Vue.js is a progressive JavaScript framework used for building user interfaces. Its simplicity, flexibility, and performance make it a popular choice among developers for building single-page applications (SPAs) and user interface components.
GraphQL
GraphQL is a query language for APIs and a runtime for executing those queries with your existing data. Unlike traditional RESTful APIs, GraphQL allows clients to request only the data they need, enabling more efficient data fetching and reducing over-fetching or under-fetching of data.
Benefits of Using Vue.js with GraphQL
Efficient Data Fetching
One of the primary advantages of using GraphQL with Vue.js is the ability to fetch only the data required by the client. This minimizes network bandwidth usage and reduces the load on servers, resulting in faster and more responsive applications.
Declarative Data Fetching
Vue.js’s reactive data-binding capabilities make it easy to integrate GraphQL queries directly into your components. By declaring the data dependencies of each component, Vue.js can automatically fetch and update the necessary data when it changes, ensuring that your UI remains in sync with your backend.
Improved Developer Experience
GraphQL’s strongly-typed schema and introspection capabilities provide developers with powerful tools for understanding and exploring their data graph. Combined with Vue.js’s intuitive syntax and comprehensive documentation, developers can quickly build and iterate on complex features with confidence.
Examples of Vue.js and GraphQL in Action
Fetching User Data
Suppose you’re building a social media platform with Vue.js and GraphQL. Instead of making separate API requests for a user’s profile information, posts, followers, and following, you can use a single GraphQL query to fetch all of this data in one go:
query { user(id: "123") { id name email posts { id title content } followers { id name } following { id name } } }
This query returns a nested structure containing all the relevant data for a user, allowing you to efficiently populate your Vue.js components with the necessary information.
Pagination and Infinite Scrolling
Let’s say you’re building a news website with Vue.js and GraphQL. To implement pagination and infinite scrolling, you can use GraphQL’s first and after arguments to fetch a subset of articles based on their publication date:
query { articles(first: 10, after: "2023-01-01T00:00:00Z") { edges { node { id title content publishedAt } } pageInfo { hasNextPage endCursor } } }
By fetching only the articles that are currently visible on the screen, you can minimize unnecessary data transfer and improve the performance of your Vue.js application.
Real-Time Updates with Subscriptions
Suppose you’re building a chat application with Vue.js and GraphQL. To enable real-time updates when new messages are sent, you can use GraphQL subscriptions to listen for changes to the chat room:
subscription { messageSent(roomId: "123") { id text createdAt sender { id name } } }
Whenever a new message is sent in the specified chat room, the subscription will notify your Vue.js application, allowing you to update the UI in real-time without polling the server repeatedly.
Conclusion
By combining the strengths of Vue.js and GraphQL, developers can build web applications that are not only powerful and feature-rich but also highly efficient and responsive. Whether you’re fetching user data, implementing pagination, or enabling real-time updates, Vue.js and GraphQL provide the tools you need to deliver exceptional user experiences on the web.
If you’re interested in learning more about Vue.js and GraphQL, check out the following resources:
Table of Contents
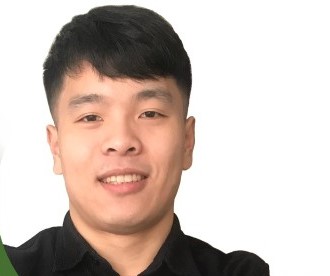
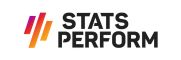