Demystifying Vue.js: An In-Depth Guide to Building Dynamic Web Applications
Vue.js, an open-source JavaScript framework, is an excellent choice for building dynamic web interfaces. This surge in popularity has resulted in a high demand to hire Vue.js developers. This post will guide both seasoned developers and those looking to hire Vue.js developers through the basic and advanced concepts of Vue.js. By the end of this guide, you’ll understand the simplicity and flexibility of Vue.js that helps in building robust web applications.
What is Vue.js?
Vue.js, pronounced as “view,” is a progressive JavaScript framework used to create dynamic and rich web applications. The framework was created by Evan You, a former Google engineer, in 2014, with an aim to simplify web development by creating an easy-to-understand, flexible, and performant JavaScript framework. Vue’s most significant advantages include its gentle learning curve, robust ecosystem, and reactivity, enabling developers to create complex applications with less code.
Getting Started with Vue.js
To begin with Vue.js, you need a basic understanding of HTML, CSS, and JavaScript. Aspiring Vue.js developers or businesses looking to hire Vue.js developers will find that this skillset is a great starting point. Let’s jump right into coding a basic Vue.js application. Whether you’re aiming to refine your skills or you’re preparing to hire Vue.js developers for your team, you can add Vue.js to your project by linking directly to the Vue.js CDN or by installing it via npm.
Example: Linking via CDN
```html <script src="https://unpkg.com/vue@next"></script> ```
Example: Installing via npm
```bash npm install vue@next ```
After installation, let’s create a simple Vue.js application.
```html <div id="app"> {{ message }} </div> <script> var app = new Vue({ el: '#app', data: { message: 'Hello Vue.js!' } }); </script> ```
In this example, the `{{ message }}` syntax is a placeholder for data that you want to display. Vue.js uses this syntax, known as declarative rendering, to keep the HTML and JavaScript code separated yet linked. Here, `message` is a property of the `data` object in the Vue instance.
Vue Components
One of the fundamental aspects of Vue.js is components. Components are reusable chunks of code that encapsulate a part of the user interface. Think of them as custom HTML elements with their own data and behavior.
Example: Defining a Component
```javascript Vue.component('my-component', { template: '<div>A custom component!</div>' }) ```
Example: Using a Component
```html <div id="app"> <my-component></my-component> </div> ```
Vue Directives
Vue.js uses directives to apply special reactive behavior to rendered DOM. These are special attributes with the `v-` prefix that indicate Vue.js should do something to a DOM element.
v-if, v-else
`v-if` and `v-else` directives can be used to conditionally render elements.
Example:
```html <div id="app"> <p v-if="seen">Now you see me</p> <p v-else>Now you don't</p> </div> <script> var app = new Vue({ el: '#app', data: { seen: true } }); </script> ```
v-for
`v-for` directive is used for rendering lists.
Example:
```html <div id="app"> <ol> <li v-for="item in items"> {{ item.text }} </li> </ol> </div> <script> var app = new Vue({ el: '#app', data: { items: [ { text: 'Learn JavaScript' }, { text: 'Learn Vue.js' }, { text: 'Build something awesome' } ] }
```html <div id="app"> <p>{{ message }}</p> <button v-on:click="reverseMessage">Reverse Message</button> </div> <script> var app = new Vue({ el: '#app', data: { message: 'Hello Vue.js!' }, methods: { reverseMessage: function () { this.message = this.message.split('').reverse().join('') } } }); </script> ```
In the example above, we’ve attached a click event listener to the button that triggers the `reverseMessage` method upon a click.
Vue Methods
Vue.js allows you to define methods that can be used in the Vue instance. This feature is handy for encapsulating actions that need to be performed multiple times throughout your application.
Example: Using Vue Methods
```html <div id="app"> <p>Original message: "{{ message }}"</p> <p>Computed reversed message: "{{ reversedMessage }}"</p> </div> <script> var app = new Vue({ el: '#app', data: { message: 'Hello Vue.js!' }, computed: { reversedMessage: function () { return this.message.split('').reverse().join('') } } }); </script> ```
Vue Computed Properties
For any complex logic, Vue.js provides computed properties. A computed property is like a method, but Vue.js caches it and only updates the value when a dependency changes.
Example: Using Computed Properties
```html <div id="app"> <p>Original message: "{{ message }}"</p> <p>Computed reversed message: "{{ reversedMessage }}"</p> </div> <script> var app = new Vue({ el: '#app', data: { message: 'Hello Vue.js!' }, computed: { reversedMessage: function () { return this.message.split('').reverse().join('') } } }); </script> ```
Vue Router
The Vue Router allows us to create single-page applications where navigation between the pages does not cause the page to refresh.
First, install the Vue Router:
```bash npm install vue-router@next ```
Then you can define the routes like this:
```javascript import { createRouter, createWebHistory } from 'vue-router' const routes = [ { path: '/', component: Home }, { path: '/about', component: About } ] const router = createRouter({ history: createWebHistory(), routes }) ```
You can then navigate between pages using the `<router-link>` component.
```html <router-link to="/">Home</router-link> <router-link to="/about">About</router-link> <router-view/> ```
Vuex for State Management
When working with complex applications, state management becomes crucial. Vuex is a state management library developed specifically for Vue.js to maintain a centralized store for all components in an application.
To use Vuex, install it via npm:
```bash npm install vuex@next ```
Then, create a store:
```javascript import { createStore } from 'vuex' const store = createStore({ state () { return { count: 0 } }, mutations: { increment (state) { state.count++ } } }) ```
You can then commit a mutation like this:
```javascript store.commit('increment') console.log(store.state.count) // -> 1 ```
Conclusion
And that’s it! You’ve now been introduced to the fundamental concepts of Vue.js, such as directives, components, methods, computed properties, Vue Router, and Vuex. It’s no wonder companies are eager to hire Vue.js developers. This powerful framework, when mastered, can drastically speed up your web development process. Its simple syntax and flexible structure make it a top choice for developers and companies alike. Whether you’re looking to hire Vue.js developers or you’re on your own coding journey, remember that with Vue.js, you’re only limited by your creativity. Happy coding!
Table of Contents
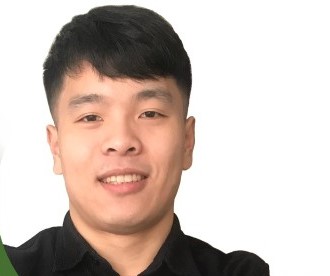
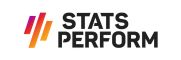