Vue.js and Laravel: Building Full-Stack Web Applications
Building full-stack web applications requires a robust combination of front-end and back-end technologies. Vue.js and Laravel are two popular frameworks that, when used together, offer a powerful solution for creating modern, efficient web applications. This article explores how Vue.js can be paired with Laravel to build comprehensive web applications, providing practical examples and best practices for integrating these technologies.
Understanding Vue.js and Laravel
Vue.js is a progressive JavaScript framework used for building user interfaces. It is designed to be incrementally adoptable, meaning it can be integrated into projects of various scales, from single-page applications (SPAs) to complex web applications.
Laravel is a PHP framework known for its elegant syntax and comprehensive feature set. It simplifies back-end development with tools for routing, authentication, and database management, making it a popular choice for building robust server-side applications.
Using Vue.js with Laravel
Combining Vue.js and Laravel allows you to build full-stack applications where Vue.js handles the client-side logic and user interface, while Laravel manages the server-side operations. Here’s how you can effectively integrate Vue.js with Laravel:
1. Setting Up the Environment
To get started, you need to set up a Laravel project and integrate Vue.js into it. Laravel comes with built-in support for Vue.js, which simplifies the integration process.
Example: Installing Laravel and Vue.js
1. Create a new Laravel project:
```bash composer create-project --prefer-dist laravel/laravel my-laravel-app ```
2. Navigate to the project directory:
```bash cd my-laravel-app ```
3. Install the necessary npm packages:
```bash npm install ```
4. Install Vue.js:
```bash npm install vue ```
5. Update `resources/js/app.js` to include Vue.js:
```javascript import Vue from 'vue'; import ExampleComponent from './components/ExampleComponent.vue'; new Vue({ el: 'app', components: { ExampleComponent } }); ```
2. Building Front-End Components with Vue.js
Vue.js allows you to build reusable components that can be used to create dynamic user interfaces.
Example: Creating a Vue Component
Create a new Vue component in `resources/js/components/ExampleComponent.vue`:
```html <template> <div> <h1>{{ message }}</h1> </div> </template> <script> export default { data() { return { message: 'Hello from Vue.js!' } } } </script> <style scoped> h1 { color: 42b983; } </style> ```
Include this component in your Laravel Blade template (`resources/views/welcome.blade.php`):
```html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Vue.js and Laravel</title> </head> <body> <div id="app"> <example-component></example-component> </div> <script src="{{ mix('js/app.js') }}"></script> </body> </html> ```
3. Handling Back-End Operations with Laravel
Laravel provides a robust back-end framework for handling server-side logic, including routing, data handling, and authentication.
Example: Creating an API Endpoint
1. Define a route in `routes/api.php`:
```php Route::get('/api/data', 'ApiController@getData'); ```
2. Create a controller (`app/Http/Controllers/ApiController.php`):
```php <?php namespace App\Http\Controllers; use Illuminate\Http\Request; class ApiController extends Controller { public function getData() { return response()->json(['message' => 'Data from Laravel API']); } } ```
3. Fetch data from this endpoint in your Vue component:
```javascript <template> <div> <h1>{{ apiData }}</h1> </div> </template> <script> export default { data() { return { apiData: '' } }, mounted() { fetch('/api/data') .then(response => response.json()) .then(data => { this.apiData = data.message; }); } } </script> ```
4. Best Practices for Integration
– Component Organization: Keep your Vue components modular and organized to ensure maintainability and scalability.
– API Design: Follow RESTful API design principles to make your back-end services easy to use and understand.
– State Management: Use Vuex for state management in larger applications to handle complex state logic efficiently.
– Error Handling: Implement proper error handling on both the client and server sides to provide a smooth user experience.
Conclusion
Integrating Vue.js with Laravel provides a powerful framework for building full-stack web applications. By combining Vue.js’s dynamic front-end capabilities with Laravel’s robust back-end features, developers can create efficient, scalable, and maintainable web applications. Leveraging these technologies together enables a streamlined development process and a seamless user experience.
Further Reading:
Table of Contents
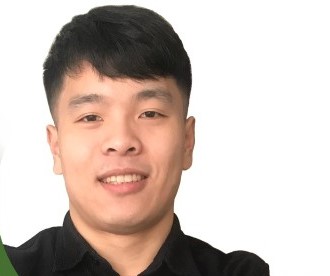
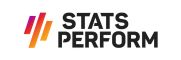