Vue.js Lazy Loading: Optimizing Load Times for Large Applications
In modern web development, optimizing load times is crucial for providing a smooth and responsive user experience. Vue.js, a popular JavaScript framework, offers powerful techniques for lazy loading, which can significantly improve the performance of large applications. This article explores how to implement lazy loading in Vue.js and provides practical examples to help you optimize your application’s load times.
Understanding Lazy Loading
Lazy loading is a design pattern that postpones the loading of non-essential resources until they are needed. This approach can reduce initial load times, decrease bandwidth usage, and improve overall performance by only loading components or data when they are required.
Implementing Lazy Loading in Vue.js
Vue.js provides several ways to implement lazy loading for components, routes, and other resources. Below are key techniques and examples to help you get started.
1. Lazy Loading Vue Components
Vue.js allows you to dynamically import components, which can be useful for reducing the initial bundle size. This technique is achieved using the `import()` function.
Example: Lazy Loading a Vue Component
Assume you have a large component that is not always needed. You can use the following code to lazy load it:
```javascript <template> <div> <button @click="showComponent">Load Component</button> <component :is="asyncComponent" /> </div> </template> <script> export default { data() { return { asyncComponent: null }; }, methods: { async showComponent() { this.asyncComponent = () => import('./LargeComponent.vue'); } } } </script> ```
2. Lazy Loading Vue Routes
For single-page applications (SPAs), lazy loading routes can be highly effective. Vue Router supports route-level code splitting, which can be implemented using dynamic imports.
Example: Lazy Loading Routes with Vue Router
Here’s how to configure lazy loading for routes in Vue Router:
```javascript import Vue from 'vue'; import Router from 'vue-router'; Vue.use(Router); const routes = [ { path: '/home', component: () => import('./components/Home.vue') }, { path: '/about', component: () => import('./components/About.vue') } ]; export default new Router({ routes }); ```
3. Lazy Loading Images
Lazy loading images can improve the loading time of pages that contain many images. Vue.js can be combined with libraries like `vue-lazyload` to achieve this.
Example: Using `vue-lazyload` for Image Optimization
First, install `vue-lazyload`:
```bash npm install vue-lazyload ```
Then, configure it in your Vue application:
```javascript import Vue from 'vue'; import VueLazyload from 'vue-lazyload'; Vue.use(VueLazyload); export default { template: ` <div> <img v-lazy="'/path/to/image.jpg'" alt="Lazy loaded image" /> </div> ` } ```
4. Optimizing Lazy Loading Performance
While lazy loading can greatly enhance performance, it’s essential to ensure it is implemented efficiently. Avoid excessive lazy loading, and monitor the impact on the user experience.
Example: Monitoring and Adjusting Lazy Loading
You can use performance monitoring tools to track the impact of lazy loading on your application. Analyze load times, user interactions, and resource usage to make informed adjustments.
Conclusion
Implementing lazy loading in Vue.js can significantly enhance the performance of large applications by optimizing load times and reducing resource usage. By using techniques such as lazy loading components, routes, and images, you can provide a smoother and more efficient user experience. Embrace these strategies to keep your Vue.js applications responsive and performant.
Further Reading:
Table of Contents
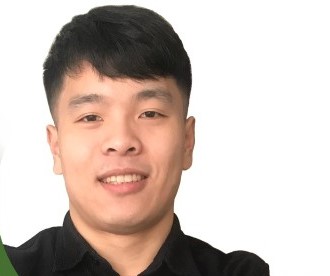
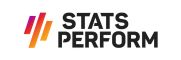