Vue.js Plugin Development: Creating and Distributing Reusable Extensions
Vue.js is a versatile framework that allows developers to build powerful web applications with ease. One of its strengths is its extensibility through plugins, which can enhance the functionality of your application. This guide explores how to develop Vue.js plugins, providing practical examples and best practices for creating and distributing reusable extensions.
Understanding Vue.js Plugins
Vue.js plugins are JavaScript libraries that extend the core functionality of Vue applications. They can add global features, components, directives, or instance methods to your Vue projects. Effective plugin development can simplify code, promote reusability, and facilitate better application architecture.
Developing a Vue.js Plugin
Creating a Vue.js plugin involves several key steps. Here’s a breakdown of how to develop and implement a Vue.js plugin:
1. Setting Up the Plugin Structure
A typical Vue.js plugin is a JavaScript file or module that exports an install function. This function is used to register the plugin with a Vue instance.
Example: Basic Plugin Structure
```javascript // my-plugin.js const MyPlugin = { install(Vue, options) { // Add a global method or property Vue.prototype.$myMethod = function () { console.log('Plugin method called'); }; // Add a global component Vue.component('my-component', { template: '<div>A custom component!</div>', }); } }; export default MyPlugin; ```
2. Installing the Plugin
To use the plugin in a Vue application, you need to import and register it with the Vue instance.
Example: Using the Plugin in a Vue App
```javascript import Vue from 'vue'; import App from './App.vue'; import MyPlugin from './my-plugin'; // Install the plugin Vue.use(MyPlugin); new Vue({ render: h => h(App), }).$mount('#app'); ```
3. Creating a Plugin with Options
Plugins often need to accept options to customize their behavior. The install function can accept an options parameter to handle such cases.
Example: Plugin with Options
```javascript // my-plugin.js const MyPlugin = { install(Vue, options) { // Use options to customize the plugin Vue.prototype.$myMethod = function () { console.log(options.message || 'Default message'); }; } }; export default MyPlugin; ```
Usage with Options
```javascript import Vue from 'vue'; import App from './App.vue'; import MyPlugin from './my-plugin'; // Install the plugin with options Vue.use(MyPlugin, { message: 'Hello from plugin!' }); new Vue({ render: h => h(App), }).$mount('#app'); ```
4. Testing Your Plugin
Testing is crucial to ensure your plugin works correctly. Consider writing unit tests to validate the functionality of your plugin.
Example: Basic Unit Test Using Jest
```javascript import Vue from 'vue'; import MyPlugin from './my-plugin'; Vue.use(MyPlugin); describe('MyPlugin', () => { it('should add a global method', () => { const vm = new Vue(); expect(typeof vm.$myMethod).toBe('function'); }); }); ```
Distributing Your Plugin
Once your plugin is ready, you can distribute it to the Vue community or your team. Here are some common ways to distribute Vue.js plugins:
1. Publishing to npm
You can publish your plugin to npm for easy installation and version management.
Steps to Publish to npm:
- Prepare your plugin: Ensure it’s properly packaged with a `package.json` file.
- Login to npm: Run `npm login` to authenticate.
- Publish the package: Run `npm publish` to upload your plugin.
2. Hosting on GitHub
You can also host your plugin on GitHub and provide installation instructions in your README file.
Example README Installation Instructions
```markdown ## Installation ```bash npm install my-plugin ``` ## Usage ```javascript import Vue from 'vue'; import MyPlugin from 'my-plugin'; Vue.use(MyPlugin); ``` ```
Conclusion
Vue.js plugin development allows you to extend and customize your Vue applications effectively. By following the steps outlined above, you can create powerful, reusable plugins that enhance your application’s functionality. Whether you’re distributing your plugin via npm or GitHub, the ability to share your work with the community or your team is a valuable skill.
Further Reading:
Table of Contents
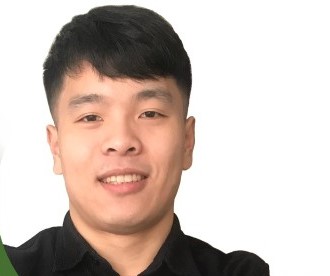
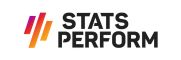