Vue.js Serverless Functions: Building Scalable Backend Services
As web applications grow in complexity and user demand increases, scalability becomes a critical concern. Serverless architecture provides a powerful solution for building scalable backend services without the need to manage traditional server infrastructure. Combining this with Vue.js, a popular front-end framework, can streamline development and improve application performance. This article explores how to use Vue.js with serverless functions to create efficient and scalable backend services.
Understanding Serverless Architecture
Serverless architecture allows developers to build and deploy applications without managing server infrastructure. Instead of handling server provisioning and maintenance, developers use cloud providers’ serverless functions to execute code in response to events. This approach offers automatic scaling, reduced operational overhead, and cost efficiency.
Using Vue.js with Serverless Functions
Vue.js is a progressive JavaScript framework for building user interfaces. By integrating Vue.js with serverless functions, you can handle backend logic and data processing efficiently. Below are key aspects and code examples demonstrating how Vue.js can interact with serverless functions.
1. Setting Up Vue.js for Serverless Integration
To begin, you’ll need to set up a Vue.js project and configure it to interact with serverless functions. You can use tools like the Vue CLI to initialize your project.
Example: Initializing a Vue.js Project
```bash vue create my-vue-app cd my-vue-app npm install axios ```
Using Axios to Communicate with Serverless Functions
Axios is a popular HTTP client that can be used in Vue.js to make requests to serverless functions.
```javascript // src/services/api.js import axios from 'axios'; const API_URL = 'https://your-serverless-function-url.com'; export const fetchData = async () => { try { const response = await axios.get(`${API_URL}/data`); return response.data; } catch (error) { console.error('Error fetching data:', error); throw error; } }; ```
2. Creating Serverless Functions
Serverless functions are deployed on cloud platforms like AWS Lambda, Azure Functions, or Google Cloud Functions. These functions handle backend logic and interact with databases or other services.
Example: AWS Lambda Function
Here’s a simple AWS Lambda function that responds to HTTP requests:
```javascript // index.js exports.handler = async (event) => { const response = { statusCode: 200, body: JSON.stringify({ message: 'Hello from serverless!' }), }; return response; }; ```
Deploying the Function
Deploy your function using the AWS CLI or AWS Management Console.
```bash aws lambda create-function --function-name my-function --runtime nodejs14.x --role arn:aws:iam::account-id:role/lambda-role --handler index.handler --zip-file fileb://function.zip ```
3. Connecting Vue.js with Serverless Functions
Once your serverless function is deployed, you can connect it with your Vue.js frontend. Use Axios or Fetch API to make HTTP requests to your serverless endpoint.
Example: Fetching Data in Vue.js
```javascript // src/components/DataComponent.vue <template> <div> <h1>Data from Serverless Function</h1> <pre>{{ data }}</pre> </div> </template> <script> import { fetchData } from '../services/api'; export default { data() { return { data: null, }; }, async created() { this.data = await fetchData(); }, }; </script> ```
4. Handling Authentication and Security
Ensure your serverless functions are secure by implementing authentication and authorization mechanisms. Use services like AWS API Gateway to manage access control.
Example: Securing an API Gateway
Configure API Gateway to use AWS Cognito for user authentication and restrict access to your serverless function.
Conclusion
Integrating Vue.js with serverless functions allows you to build scalable and efficient backend services without managing server infrastructure. By leveraging serverless architecture, you can focus on developing your Vue.js frontend while relying on cloud providers for backend scalability and maintenance. Embrace this approach to streamline your development process and enhance your application’s performance.
Further Reading:
Table of Contents
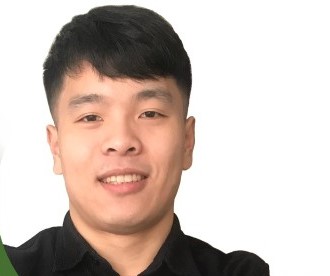
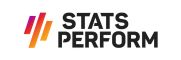