Vue.js and Storybook: Designing and Documenting UI Components
Designing and documenting UI components is a crucial aspect of modern web development. Vue.js, known for its flexible and efficient framework, combined with Storybook, a powerful tool for UI component development, offers an excellent solution for creating and managing UI components. This article explores how Vue.js and Storybook can be integrated to streamline the process of designing, documenting, and testing UI components.
Understanding Vue.js and Storybook
Vue.js is a progressive JavaScript framework used for building user interfaces and single-page applications. It offers a component-based architecture that facilitates the development of reusable and maintainable UI elements. Storybook, on the other hand, is an open-source tool that allows developers to build and document UI components in isolation, making it easier to develop and test components independently from the main application.
Using Vue.js with Storybook
Integrating Storybook with Vue.js provides a powerful environment for developing and showcasing UI components. Below are some key aspects and examples demonstrating how to use Vue.js with Storybook for effective UI component design and documentation.
-
Setting Up Storybook for Vue.js
To get started with Storybook and Vue.js, you need to install Storybook and configure it for your Vue.js project. Here’s how you can set up Storybook:
Example: Installing and Configuring Storybook
bash Copy code # Install Storybook npx sb init --type vue # Run Storybook npm run storybook
Once installed, Storybook will create a .storybook directory in your project, which contains configuration files.
-
Creating Stories for Vue.js Components
Storybook uses stories to showcase different states and variations of UI components. Each story represents a specific state or variant of a component.
Example: Writing Stories for a Button Component
javascript Copy code // Button.stories.js import MyButton from './MyButton.vue'; export default { title: 'Components/MyButton', component: MyButton, }; export const Default = () => ({ components: { MyButton }, template: '<MyButton>Click Me</MyButton>', }); export const Disabled = () => ({ components: { MyButton }, template: '<MyButton :disabled="true">Disabled</MyButton>', });
In this example, Default and Disabled are stories that demonstrate different states of the MyButton component.
-
Designing and Documenting Components
Storybook provides an interactive environment to design and document components. It allows you to visualize how components look and behave with different props and states.
Example: Adding Documentation with Storybook’s Docs Addon
javascript Copy code // Button.stories.js import MyButton from './MyButton.vue'; import { Meta, Story } from '@storybook/vue3'; export default { title: 'Components/MyButton', component: MyButton, parameters: { docs: { description: { component: 'A button component that can be used for various actions.', }, }, }, } as Meta; const Template: Story = (args) => ({ components: { MyButton }, setup() { return { args }; }, template: '<MyButton v-bind="args">Click Me</MyButton>', }); export const Default = Template.bind({}); Default.args = { disabled: false, }; export const Disabled = Template.bind({}); Disabled.args = { disabled: true, };
By adding documentation parameters and descriptions, you can provide valuable information about each component directly within Storybook.
-
Testing UI Components
Storybook also supports testing UI components through addons like Storybook’s Interaction Tests and Storyshots.
Example: Setting Up Storyshots for Snapshot Testing
bash Copy code # Install Storyshots npm install @storybook/addon-storyshots --save-dev
Create a storyshots.test.js file:
javascript Copy code import initStoryshots from '@storybook/addon-storyshots'; initStoryshots();
This setup enables snapshot testing for your Storybook stories, ensuring that UI components render correctly and consistently.
Conclusion
Integrating Vue.js with Storybook enhances the process of designing, documenting, and testing UI components. By leveraging Storybook’s interactive environment and Vue.js’s component-based architecture, developers can streamline their UI development workflow and ensure consistency across their projects. Utilizing these tools effectively will lead to a more organized and efficient approach to UI component management.
Further Reading:
Table of Contents
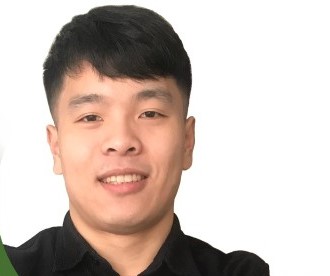
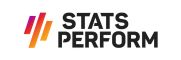