Vue.js and Tailwind UI: Designing Beautiful and Responsive Interfaces
Vue.js is a progressive JavaScript framework known for its flexibility and ease of use, while Tailwind UI is a utility-first CSS framework that enables rapid, responsive design. Combining these two technologies can lead to beautiful, efficient web applications with minimal effort. This article explores how to use Vue.js and Tailwind UI together to build visually appealing and responsive interfaces.
Understanding Vue.js and Tailwind UI
Vue.js is a popular front-end framework that simplifies the process of building interactive user interfaces. Its component-based architecture allows for modular and maintainable code.
Tailwind UI provides a set of pre-designed, customizable components built with Tailwind CSS. Tailwind CSS’s utility-first approach promotes rapid styling and responsiveness without writing custom CSS.
Integrating Vue.js with Tailwind UI
Combining Vue.js and Tailwind UI allows developers to build robust and visually appealing web applications efficiently. Here are some practical examples of how to achieve this integration:
1. Setting Up Your Project
Start by creating a new Vue.js project and installing Tailwind CSS. You can use Vue CLI to scaffold a new project and then add Tailwind CSS as a dependency.
Example: Setting Up Vue.js with Tailwind CSS
1. Create a New Vue Project:
```bash Vue create my-project ```
2. Navigate to the Project Directory:
```bash cd my-project ```
3. Install Tailwind CSS:
```bash npm install -D tailwindcss postcss autoprefixer ```
4. Create a Tailwind Configuration File:
```bash npx tailwindcss init ```
5. Configure Tailwind in `tailwind.config.js`:
```javascript module.exports = { content: [ "./src//*.{js,jsx,ts,tsx,vue}", ], theme: { extend: {}, }, plugins: [], } ```
6. Include Tailwind in Your CSS:
In your `src/assets/styles/tailwind.css` file:
```css @tailwind base; @tailwind components; @tailwind utilities; ``` And import it in your `main.js`: ```javascript import './assets/styles/tailwind.css' ```
2. Designing a Responsive Layout
With Tailwind UI’s pre-designed components, you can quickly create responsive layouts. For instance, using a responsive card component in Vue.js.
Example: Creating a Responsive Card Component
1. Install Tailwind UI Components:
Tailwind UI components are available for purchase, so include them in your project as per the guidelines provided.
2. Create a Vue Component Using Tailwind UI:
`Card.vue` ```html <template> <div class="max-w-sm mx-auto bg-white shadow-lg rounded-lg overflow-hidden"> <img class="w-full" :src="imageUrl" alt="Card image"> <div class="p-4"> <h2 class="text-xl font-bold mb-2">{{ title }}</h2> <p class="text-gray-700">{{ description }}</p> </div> </div> </template> <script> export default { props: { imageUrl: String, title: String, description: String } } </script> <style scoped> /* Custom styles if needed */ </style> ```
3. Use the Card Component in Your App:
`App.vue`
```html <template> <div class="p-6"> <Card imageUrl="https://example.com/image.jpg" title="Sample Card" description="This is a description of the card." /> </div> </template> <script> import Card from './components/Card.vue'; export default { components: { Card } } </script> ```
3. Building a Responsive Navigation Bar
Tailwind UI provides responsive navigation components that can be easily integrated into your Vue.js application.
Example: Creating a Responsive Navbar
1. Create a Navbar Component:
`Navbar.vue` ```html <template> <nav class="bg-gray-800 p-4"> <div class="container mx-auto flex justify-between items-center"> <div class="text-white text-xl">MyApp</div> <div class="hidden md:flex space-x-4"> <a href="#" class="text-gray-300 hover:text-white">Home</a> <a href="#" class="text-gray-300 hover:text-white">About</a> <a href="#" class="text-gray-300 hover:text-white">Contact</a> </div> <button class="md:hidden text-gray-300 hover:text-white"> <!-- Menu Icon for Mobile --> <svg class="w-6 h-6" fill="none" stroke="currentColor" viewBox="0 0 24 24" xmlns="http://www.w3.org/2000/svg"> <path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M4 6h16M4 12h16m-7 6h7"></path> </svg> </button> </div> </nav> </template> <script> export default { name: 'Navbar' } </script> ```
2. Use the Navbar Component in Your App:
`App.vue` ```html <template> <div> <Navbar /> <main class="p-6"> <!-- Main content here --> </main> </div> </template> <script> import Navbar from './components/Navbar.vue'; export default { components: { Navbar } } </script> ```
4. Leveraging Tailwind UI Components
Tailwind UI offers a wide range of components that can be customized and used within Vue.js applications. These components can help you maintain a consistent design while speeding up development.
Example: Using Tailwind UI Forms
- Create a Form Component Using Tailwind UI:
`ContactForm.vue` ```html <template> <div class="max-w-md mx-auto bg-white p-6 rounded-lg shadow-lg"> <h2 class="text-2xl font-bold mb-4">Contact Us</h2> <form> <div class="mb-4"> <label for="name" class="block text-sm font-medium text-gray-700">Name</label> <input type="text" id="name" class="mt-1 block w-full border-gray-300 rounded-md shadow-sm focus:border-indigo-500 focus:ring-indigo-500 sm:text-sm" /> </div> <div class="mb-4"> <label for="email" class="block text-sm font-medium text-gray-700">Email</label> <input type="email" id="email" class="mt-1 block w-full border-gray-300 rounded-md shadow-sm focus:border-indigo-500 focus:ring-indigo-500 sm:text-sm" /> </div> <div class="mb-4"> <label for="message" class="block text-sm font-medium text-gray-700">Message</label> <textarea id="message" rows="4" class="mt-1 block w-full border-gray-300 rounded-md shadow-sm focus:border-indigo-500 focus:ring-indigo-500 sm:text-sm"></textarea> </div> <button type="submit" class="bg-indigo-600 text-white px-4 py-2 rounded-md hover:bg-indigo-700">Send</button> </form> </div> </template> <script> export default { name: 'ContactForm' } </script> ```
2. Use the Form Component in Your App:
`App.vue`
```html <template> <div> <ContactForm /> </div> </template> <script> import ContactForm from './components/ContactForm.vue'; export default { components: { ContactForm } } </script> ```
Conclusion
Vue.js and Tailwind UI are a powerful combination for designing modern, responsive web interfaces. By leveraging Vue.js’s component-based architecture and Tailwind UI’s utility-first design, developers can create visually appealing and efficient web applications. With the examples provided, you can start building beautiful and responsive user interfaces in your Vue.js projects today.
Further Reading:
Table of Contents
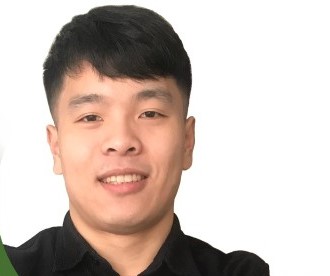
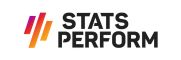