Vue.js Best Practices: Tips and Techniques for Clean Code
Vue.js is a popular framework for building dynamic and reactive user interfaces. To harness its full potential and create scalable applications, adhering to best practices is essential. This blog explores key best practices for writing clean, maintainable, and efficient Vue.js code.
Understanding Vue.js Best Practices
Writing clean code in Vue.js involves following guidelines and techniques that improve code readability, maintainability, and efficiency. By adopting these best practices, developers can create robust Vue.js applications that are easier to manage and extend.
Vue.js Best Practices for Clean Code
1. Structuring Your Project
Organizing your Vue.js project structure helps in maintaining a clean and manageable codebase. A well-organized project facilitates easier navigation and development.
Best Practice: Use a modular file structure that separates concerns.
Example:
```plaintext src/ ??? assets/ ? ??? images/ ??? components/ ? ??? Header.vue ? ??? Footer.vue ??? views/ ? ??? Home.vue ? ??? About.vue ??? router/ ? ??? index.js ??? store/ ? ??? index.js ??? App.vue ??? main.js ```
2. Utilizing Vue.js Single File Components
Vue.js Single File Components (SFCs) allow you to encapsulate HTML, CSS, and JavaScript in a single file, promoting modularity and reusability.
Best Practice: Keep components focused and modular, adhering to the Single Responsibility Principle.
Example:
```vue <template> <div> <h1>{{ title }}</h1> </div> </template> <script> export default { name: 'HeaderComponent', props: { title: String } } </script> <style scoped> h1 { color: 42b983; } </style> ```
3. Managing State with Vuex
For complex applications, managing state effectively is crucial. Vuex provides a centralized state management pattern for Vue.js applications.
Best Practice: Use Vuex to manage global state and maintain a clear separation of concerns.
Example:
```javascript // store/index.js import Vue from 'vue'; import Vuex from 'vuex'; Vue.use(Vuex); export default new Vuex.Store({ state: { count: 0 }, mutations: { increment(state) { state.count++; } }, actions: { increment({ commit }) { commit('increment'); } }, getters: { count: state => state.count } }); ```
4. Leveraging Vue.js Directives and Filters
Vue.js provides directives and filters to handle common tasks efficiently. Using built-in directives and creating custom ones can simplify your templates and code.
Best Practice: Use directives and filters to keep templates clean and handle data transformations effectively.
Example:
```vue <template> <div> <p v-if="isVisible">This is visible</p> <p>{{ message | uppercase }}</p> </div> </template> <script> export default { data() { return { isVisible: true, message: 'hello world' }; }, filters: { uppercase(value) { return value.toUpperCase(); } } } </script> ```
5. Implementing Vue.js Lifecycle Hooks
Vue.js lifecycle hooks allow you to execute code at specific stages of a component’s lifecycle. Proper use of these hooks can improve performance and maintainability.
Best Practice: Utilize lifecycle hooks to manage component behavior and resource cleanup effectively.
Example:
```javascript export default { data() { return { count: 0 }; }, created() { console.log('Component created'); }, mounted() { console.log('Component mounted'); }, beforeDestroy() { console.log('Component will be destroyed'); } } ```
6. Testing Your Vue.js Components
Testing ensures that your Vue.js components work as expected and helps in maintaining code quality.
Best Practice: Use tools like Vue Test Utils and Jest to write unit tests for your components.
Example:
```javascript import { shallowMount } from '@vue/test-utils'; import HeaderComponent from '@/components/HeaderComponent.vue'; describe('HeaderComponent.vue', () => { it('renders props.title when passed', () => { const title = 'Test Title'; const wrapper = shallowMount(HeaderComponent, { propsData: { title } }); expect(wrapper.text()).toMatch(title); }); }); ```
Conclusion
Adhering to best practices in Vue.js ensures that your codebase remains clean, maintainable, and efficient. By structuring your project properly, utilizing Vuex for state management, leveraging Vue.js directives, and implementing lifecycle hooks and testing, you can create robust and scalable Vue.js applications.
Further Reading
Table of Contents
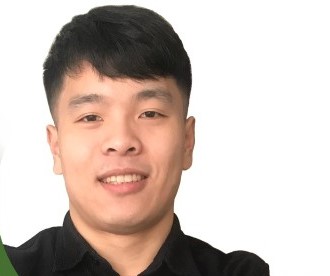
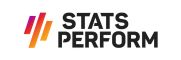